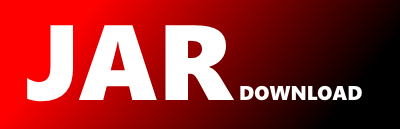
com.aliyun.ReportManager Maven / Gradle / Ivy
package com.aliyun;
import com.aliyun.bean.DefaultConfigBean;
import com.aliyun.bean.RDataModel;
import com.aliyun.utils.HttpUtils;
import com.aliyun.utils.PluginUtils;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.maven.plugin.logging.Log;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.UUID;
public class ReportManager {
private static final String RDATA_REMOTE_URL = "http://toolkit.aliyun.com/stat.html";
private static final String HOST_IDENTITY_FILE =
System.getProperty("user.home")
+ File.separator + ".cloud_toolkit" + File.separator + "maven_plugin_uuid";
private Log logger;
public ReportManager( Log logger) {
this.logger = logger;
}
public void report(DefaultConfigBean config, boolean success) {
try {
RDataModel model = getModel(config, success);
String jsonStr = modelToJson(model);
String body = jsonStr.substring(1, jsonStr.length() - 1);
HttpUtils.postPlainText(RDATA_REMOTE_URL, body, 1000);
} catch (Throwable t) {
//do nothing
}
}
private String modelToJson(RDataModel model) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.writeValueAsString(model);
}
private RDataModel getModel(DefaultConfigBean config, boolean success) {
RDataModel model = new RDataModel(getDeviceId());
model.setIdeType(RDataModel.IdeType.MAVEN);
model.setIdeVersion(getMavenVersion());
model.setPluginVersion(PluginUtils.getVersion());
model.setHasAKSK(true);
model.setDataVersion("1.0");
Map data = new HashMap<>();
data.put("accessKeyId", config.getEnv().getAccessKeyId());
data.put("appId", config.getApp().getAppId());
data.put("type", success ? "success" : "fail");
model.setEvent(new RDataModel.REvent(RDataModel.RecordPoint.EDAS, data));
return model;
}
private String getMavenVersion() {
try {
String mavenHome = System.getProperty("maven.home");
if (mavenHome != null) {
int i = mavenHome.lastIndexOf(File.separator);
if (i != -1) {
return mavenHome.substring(i+1);
}
}
} catch (Throwable t) {
//do nothing
}
return "unknown";
}
private String getDeviceId() {
String deviceId;
File file = new File(HOST_IDENTITY_FILE);
if (file.exists()) {
byte[] encoded = new byte[0];
try {
encoded = Files.readAllBytes(Paths.get(HOST_IDENTITY_FILE));
return new String(encoded);
} catch (IOException e) {
//do nothing
}
return UUID.randomUUID().toString();
} else {
UUID uuid = UUID.randomUUID();
deviceId = uuid.toString();
File dir = new File(file.getParent());
dir.mkdirs();
try (PrintWriter out = new PrintWriter(HOST_IDENTITY_FILE)) {
out.print(deviceId);
} catch (Throwable t) {
//do nothing
}
return deviceId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy