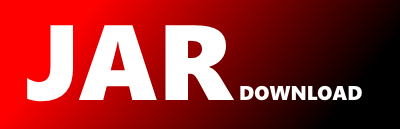
com.aliyun.uploader.BaseOssUploader Maven / Gradle / Ivy
package com.aliyun.uploader;
import com.aliyun.oss.ClientConfiguration;
import com.aliyun.oss.OSSClient;
import com.aliyun.oss.model.PutObjectRequest;
import com.aliyun.Context;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.edas.model.v20170801.QueryRegionConfigRequest;
import com.aliyuncs.edas.model.v20170801.QueryRegionConfigResponse;
import java.io.File;
import java.io.FileInputStream;
import java.net.URLEncoder;
public abstract class BaseOssUploader implements Uploader {
private static final String OSS_ENDPOINT_PTN = "http://oss-%s.aliyuncs.com";
private static final String OSS_DOWNLOAD_URL_PTN = "https://%s.oss-%s-internal.aliyuncs.com/%s";
protected String doUpload(
String edasEndpoint, String regionId, String bucket,
String key, File file, String ak, String sk, String token) throws Exception {
String ossEndpoint = String.format(OSS_ENDPOINT_PTN, regionId);
String downloadUrl = String.format(OSS_DOWNLOAD_URL_PTN, bucket, regionId,
URLEncoder.encode(key, "UTF-8").replaceAll("%2F", "/"));
if (!edasEndpoint.endsWith("aliyuncs.com")) {
DefaultAcsClient acsClient = Context.getAcsClient();
QueryRegionConfigRequest request = new QueryRegionConfigRequest();
QueryRegionConfigResponse response = acsClient.getAcsResponse(request);
if (response.getCode() != 200) {
throw new Exception(String.format(
"Unable to get oss endpoint by QueryRegionConfigRequest, code: %d, msg: %s",
response.getCode(), response.getMessage()));
}
QueryRegionConfigResponse.RegionConfig regionConfig = response.getRegionConfig();
if ("oss".equalsIgnoreCase(regionConfig.getFileServerType())
&& regionConfig.getFileServerConfig() != null) {
String internalHost = regionConfig.getFileServerConfig().getInternalUrl();
String publicHost = regionConfig.getFileServerConfig().getPublicUrl();
if (publicHost != null && internalHost != null) {
ossEndpoint = "http://" + publicHost;
downloadUrl = "http://" + bucket + "." + internalHost + "/"
+ URLEncoder.encode(key, "UTF-8").replaceAll("%2F", "/");
Context.getLogger().info("Use oss endpoint: " + ossEndpoint);
Context.getLogger().info("Use oss downloadUrl: " + downloadUrl);
} else {
Context.getLogger().info("InternalUrl in region config: " + internalHost);
Context.getLogger().info("PublicUrl in region config: " + publicHost);
}
} else {
throw new Exception(String.format(
"Fs type is %s, Fs server config is %s for current endpoint: %s",
regionConfig.getFileServerType(),
regionConfig.getFileServerConfig(),
edasEndpoint));
}
}
ClientConfiguration configuration = new ClientConfiguration();
configuration.setSupportCname(false);
OSSClient ossClient = (token == null) ? new OSSClient(ossEndpoint, ak, sk, configuration) : new OSSClient(ossEndpoint, ak,
sk, token, configuration);
ossClient.putObject(new PutObjectRequest(bucket, key, new FileInputStream(file))
.withProgressListener(new OssUploadProgressListener(file.length())));
ossClient.shutdown();
return downloadUrl;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy