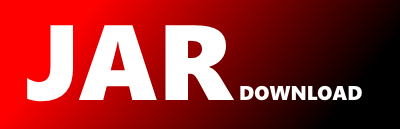
com.aliyun.manager.InitManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toolkit-maven-plugin
Show all versions of toolkit-maven-plugin
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun.manager;
import com.aliyun.Context;
import com.aliyun.bean.config.DefaultConfigBean;
import com.aliyun.enums.Constants;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.edas.model.v20170801.*;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.project.MavenProject;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.UUID;
public class InitManager {
/*
private Log logger;
private ImageManager imageManager;
private ChangeOrderManager changeOrderManager;
private UploadPackageManager uploadPackageManager;
public InitManager(MavenProject project) {
this.logger = Context.getLogger();
uploadPackageManager = new UploadPackageManager(project);
changeOrderManager = new ChangeOrderManager();
imageManager = new ImageManager();
}
public void process(DefaultConfigBean config, DefaultAcsClient client, MavenProject project) throws Exception {
processServerlessInit(config, client, project);
}
private void processServerlessInit(DefaultConfigBean config, DefaultAcsClient client, MavenProject project) throws Exception {
if (config.getInit() == null || config.getInit().getAppName() == null) {
return;
}
DefaultConfigBean.Init init = config.getInit();
logger.info("Processing init config...");
if (appExists(init, client)) {
return;
}
if (init.getPackageVersion() == null) {
init.setPackageVersion(new SimpleDateFormat("ddHHmmss").format(new Date()));
}
String imageUrl = init.getImageUrl();
String packageUrl = init.getPackageUrl();
if (Constants.IMAGE.equalsIgnoreCase(init.getPackageType())) {
if (imageUrl == null) {
imageUrl = buildAndUploadImage(init, project);
config.getInit().setImageUrl(imageUrl);
} else {
logger.info(String.format("image_url(%s) is set, use it to create serverless application...", imageUrl));
}
} else {
if (packageUrl == null) {
DefaultConfigBean.App app = config.getApp();
DefaultConfigBean.Oss oss = config.getOss();
String endpoint = config.getEnv().getEndpoint();
String regionId = config.getEnv().getRegionId();
String edasAK = config.getEnv().getAccessKeyId();
String edasSK = config.getEnv().getAccessKeySecret();
String appId = UUID.randomUUID().toString(); //创建应用前没有appId
String packageVersion = config.getInit().getPackageVersion();
packageUrl = uploadPackageManager.uploadPackage(endpoint, regionId, edasAK, edasSK, appId, packageVersion, oss);
init.setPackageUrl(packageUrl);
} else {
logger.info(String.format("package_url(%s) is set, use it to create serverless application...", packageUrl));
}
}
String appId = createServerlessApplication(init, client);
boolean status = checkAppCreateStatus(client, appId, 60);
if (!status) {
throw new Exception(String.format("Send create application request successfully, but failed to get application config. "
+ "Please login EDAS console to check if application(%s) exists.", config.getInit().getAppName()));
}
}
private boolean checkAppCreateStatus(DefaultAcsClient client, String appId, int timeout) {
logger.info("Querying application status...");
long end = System.currentTimeMillis() + timeout * 1000;
while (System.currentTimeMillis() < end) {
try {
GetServerlessAppConfigDetailRequest detailRequest = new GetServerlessAppConfigDetailRequest();
detailRequest.setAppId(appId);
GetServerlessAppConfigDetailResponse detailResponse = client.getAcsResponse(detailRequest);
if (detailResponse.getCode() == 200) {
logger.info("Create application successfully.");
return true;
}
} catch (Exception ex) {
logger.warn("Failed to query application status: " + ex.getMessage());
}
}
return false;
}
private String createServerlessApplication(
DefaultConfigBean.Init init, DefaultAcsClient client) throws Exception {
logger.info("Creating serverless application...");
CreateServerlessApplicationRequest request = new CreateServerlessApplicationRequest();
request.setAppName(init.getAppName());
request.setAppDescription(init.getAppDescription());
request.setNamespaceId(init.getNamespaceId());
request.setVpcId(init.getVpcId());
request.setVSwitchId(init.getVswitchId());
request.setPackageType(init.getPackageType());
request.setPackageVersion(init.getPackageVersion());
request.setPackageUrl(init.getPackageUrl());
request.setImageUrl(init.getImageUrl());
request.setJdk(init.getJdk());
request.setWebContainer(init.getWebContainer());
request.setCpu(init.getCpu());
request.setMemory(init.getMemory());
request.setReplicas(init.getReplicas());
request.setCommand(init.getCommand());
request.setCommandArgs(init.getCommandArgs());
request.setEnvs(init.getEnvs());
request.setCustomHostAlias(init.getCustomHostAlias());
request.setJarStartOptions(init.getJarStartOptions());
request.setJarStartArgs(init.getJarStartArgs());
request.setLiveness(init.getLiveness());
request.setReadiness(init.getReadiness());
CreateServerlessApplicationResponse response = client.getAcsResponse(request);
if (response.getCode() != 200) {
throw new Exception(String.format(
"Failed to create serverless application, code: %d, msg: %s",
response.getCode(), response.getMessage()));
}
logger.info(String.format(
"Send create application request successfully, app id is %s",
response.getData().getAppId()));
//record if has deploy
Context.setHasDeploy(Boolean.TRUE.equals(init.getDeploy()));
return response.getData().getAppId();
}
private String buildAndUploadImage(DefaultConfigBean.Init init, MavenProject project) throws Exception {
logger.info("image_url is not found in init process, try to build image...");
String repoAddress = init.getImageRepoAddress();
if (repoAddress == null) {
throw new Exception("image_repo_address is not configured in init");
}
String tag = init.getImageTag();
if (tag == null) {
throw new Exception("image_tag is not configured in init");
}
String user = init.getImageRepoUser();
if (user == null) {
throw new Exception("image_repo_user is not configured in init");
}
String password = init.getImageRepoPassword();
if (password == null) {
throw new Exception("image_repo_password is not configured in init");
}
String dockerfile = init.getDockerfile();
if (dockerfile == null) {
dockerfile = Constants.DOCKERFILE;
}
logger.info(String.format(
"Use %s to build image in current build repository(%s)",
dockerfile, project.getBasedir().getPath()));
String imageUrl = imageManager.buildAndUploadImage(
dockerfile, repoAddress, tag,
user, password, project);
logger.info("Upload image successfully, image_url is: " + imageUrl);
return imageUrl;
}
private boolean appExists(DefaultConfigBean.Init init, DefaultAcsClient client) throws Exception {
logger.info(String.format("Checking app(%s) if exists...", init.getAppName()));
if (init.getNamespaceId() == null) {
throw new Exception("namespace_id is empty in 'Init'.");
}
ListApplicationRequest request = new ListApplicationRequest();
ListApplicationResponse response = client.getAcsResponse(request);
if (response.getCode() != 200) {
throw new Exception(String.format(
"Failed to check app if exists by ListApplicationRequest, code: %d, msg: %s",
response.getCode(), response.getMessage()));
}
if (response.getApplicationList() == null) {
return false;
}
for (ListApplicationResponse.Application application: response.getApplicationList()) {
if (application.getBizRegionId().equals(init.getNamespaceId())
&& application.getName().equals(init.getAppName())) {
logger.info(String.format(
"App(%s) already exists in namespace(%s), skip init process",
init.getAppName(), init.getNamespaceId()));
return true;
}
}
return false;
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy