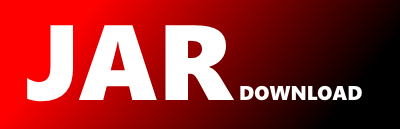
com.aliyun.manager.DeployPackageManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toolkit-maven-plugin
Show all versions of toolkit-maven-plugin
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun.manager;
import com.aliyun.Context;
import com.aliyun.bean.config.RunningEnvironment;
import com.aliyun.bean.config.ToolkitPackageConfig;
import com.aliyun.bean.config.ToolkitDeployConfig;
import com.aliyun.utils.CommonUtils;
import com.aliyun.enums.Constants;
import com.aliyun.utils.PluginUtils;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.edas.model.v20170801.DeployApplicationRequest;
import com.aliyuncs.edas.model.v20170801.DeployApplicationResponse;
import com.aliyuncs.edas.model.v20170801.DeployK8sApplicationRequest;
import com.aliyuncs.edas.model.v20170801.DeployK8sApplicationResponse;
import com.aliyuncs.edas.model.v20170801.GetK8sApplicationRequest;
import com.aliyuncs.edas.model.v20170801.GetK8sApplicationResponse;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.plugin.logging.Log;
import static com.aliyun.enums.Constants.WAR;
public class DeployPackageManager {
private Log logger;
public DeployPackageManager() {
this.logger = Context.getLogger();
}
public String deployPackage(
DefaultAcsClient defaultAcsClient,
ToolkitDeployConfig deployConfig,
ToolkitPackageConfig entityConfig) throws Exception {
if (Constants.SERVERLESS.equals(deployConfig.getSpec().getType())) {
return deployServerlessPackage(deployConfig, entityConfig, defaultAcsClient);
} else if (Constants.KUBERNETES.equals(deployConfig.getSpec().getType())){
return deployKubernetesPackage(deployConfig, entityConfig, defaultAcsClient);
} else {
return deployNonServerlessPackage(deployConfig, entityConfig, defaultAcsClient);
}
}
private String deployServerlessPackage(
ToolkitDeployConfig deployConfig,
ToolkitPackageConfig entityConfig,
DefaultAcsClient defaultAcsClient) throws Exception {
ToolkitDeployConfig.Spec spec = deployConfig.getSpec();
ObjectMapper jsonMapper = new ObjectMapper();
jsonMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
//init request
com.aliyuncs.sae.model.v20190506.DeployApplicationRequest request = new com.aliyuncs.sae.model.v20190506.DeployApplicationRequest();
request.putHeaderParameter(Constants.DEPLOY_SOURCE_HEADER_KEY, PluginUtils.getVersion());
String routeKey = CommonUtils.getPopApiRouteKey();
if (routeKey != null) {
request.putHeaderParameter(Constants.POP_API_ROUTE_KEY, routeKey);
}
request.setAppId(deployConfig.getSpec().getTarget().getAppId());
request.setPackageUrl(entityConfig.getSpec().getPackageUrl());
request.setPackageVersion(deployConfig.getSpec().getVersion());
if (entityConfig.getSpec().getImageUrl() != null) {
request.setImageUrl(entityConfig.getSpec().getImageUrl());
}
if (spec.getBatchWaitTime() != null) {
request.setBatchWaitTime(spec.getBatchWaitTime());
}
if (spec.getJdk() != null) {
request.setJdk(spec.getJdk());
}
if (deployConfig.getSpec().getWebContainer() != null) {
request.setWebContainer(deployConfig.getSpec().getWebContainer());
}
if (spec.getCommand() != null) {
request.setCommand(spec.getCommand());
}
if (spec.getCommandArgs() != null) {
request.setCommandArgs(jsonMapper.writeValueAsString(spec.getCommandArgs()));
}
if (spec.getJarStartOptions() != null) {
request.setJarStartOptions(spec.getJarStartOptions());
}
if (spec.getJarStartArgs() != null) {
request.setJarStartArgs(spec.getJarStartArgs());
}
if (spec.getEnvs() != null) {
request.setEnvs(jsonMapper.writeValueAsString(spec.getEnvs()));
}
if (spec.getCustomHostAlias() != null) {
request.setCustomHostAlias(jsonMapper.writeValueAsString(spec.getCustomHostAlias()));
}
if (spec.getLiveness() != null) {
request.setLiveness(jsonMapper.writeValueAsString(spec.getLiveness()));
}
if (spec.getReadiness() != null) {
request.setReadiness(jsonMapper.writeValueAsString(spec.getReadiness()));
}
if (spec.getMinReadyInstances() != null) {
request.setMinReadyInstances(spec.getMinReadyInstances());
}
if (spec.getUpdateStrategy() != null) {
request.setUpdateStrategy(jsonMapper.writeValueAsString(spec.getUpdateStrategy()));
}
com.aliyuncs.sae.model.v20190506.DeployApplicationResponse response = defaultAcsClient.getAcsResponse(request);
if ("200".equals(response.getCode())) {
return response.getData().getChangeOrderId();
} else {
String msg = String.format(
"Failed to send deploy request, requestId: %s, code: %s, msg: %s",
response.getRequestId(),
response.getCode(),
response.getMessage());
throw new Exception(msg);
}
}
private String deployNonServerlessPackage(
ToolkitDeployConfig deployConfig,
ToolkitPackageConfig entityConfig,
DefaultAcsClient defaultAcsClient) throws Exception {
ToolkitDeployConfig.Spec deployConfigSpec = deployConfig.getSpec();
//init request
DeployApplicationRequest request = new DeployApplicationRequest();
request.putHeaderParameter(Constants.DEPLOY_SOURCE_HEADER_KEY, PluginUtils.getVersion());
String routeKey = CommonUtils.getPopApiRouteKey();
if (routeKey != null) {
request.putHeaderParameter(Constants.POP_API_ROUTE_KEY, routeKey);
}
request.setAppId(deployConfig.getSpec().getTarget().getAppId());
request.setGroupId(deployConfigSpec.getGroupId());
if (entityConfig.getSpec().getPackageUrl() != null) {
request.setDeployType("url");
request.setWarUrl(entityConfig.getSpec().getPackageUrl());
//System.out.println(entityConfig.getSpec().getPackageUrl());
} else {
request.setDeployType("image");
request.setImageUrl(entityConfig.getSpec().getImageUrl());
}
if (deployConfigSpec.getBatch() != null) {
request.setBatch(deployConfigSpec.getBatch());
}
if (deployConfigSpec.getBatchWaitTime() != null) {
request.setBatchWaitTime(deployConfigSpec.getBatchWaitTime());
}
if (deployConfigSpec.getVersion() != null) {
request.setPackageVersion(deployConfigSpec.getVersion());
} else {
request.setPackageVersion(CommonUtils.getCurrentTime());
}
if (deployConfigSpec.getDesc() != null) {
request.setDesc(deployConfigSpec.getDesc());
}
if (deployConfigSpec.getReleaseType() != null) {
request.setReleaseType(new Long(deployConfigSpec.getReleaseType()));
}
//send request
logger.info("Sending deploy request to EDAS...");
DeployApplicationResponse response = defaultAcsClient.getAcsResponse(request);
if (response.getCode() == 200) {
String changeOrderId = response.getChangeOrderId();
logger.info("Deploy request sent, changeOrderId is: " + changeOrderId);
return changeOrderId;
} else {
String msg = String.format(
"Failed to send deploy request, requestId: %s, code: %d, msg: %s",
response.getRequestId(),
response.getCode(),
response.getMessage());
throw new Exception(msg);
}
}
private String deployKubernetesPackage(ToolkitDeployConfig deployConfig, ToolkitPackageConfig entityConfig,
DefaultAcsClient defaultAcsClient) throws Exception {
RunningEnvironment runningEnvironment = getK8sAppRunningEnvironment(
deployConfig.getSpec().getTarget().getAppId(), defaultAcsClient);
ToolkitDeployConfig.Spec spec = deployConfig.getSpec();
ObjectMapper jsonMapper = new ObjectMapper();
jsonMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
//init request
DeployK8sApplicationRequest request = new DeployK8sApplicationRequest();
request.putHeaderParameter(Constants.DEPLOY_SOURCE_HEADER_KEY, PluginUtils.getVersion());
String routeKey = CommonUtils.getPopApiRouteKey();
if (routeKey != null) {
request.putHeaderParameter(Constants.POP_API_ROUTE_KEY, routeKey);
}
request.setAppId(deployConfig.getSpec().getTarget().getAppId());
if (Constants.IMAGE.equals(entityConfig.getSpec().getPackageType())) {
if (entityConfig.getSpec().getImageUrl() != null) {
request.setImage(entityConfig.getSpec().getImageUrl());
}
} else {
request.setPackageUrl(entityConfig.getSpec().getPackageUrl());
request.setPackageVersion(deployConfig.getSpec().getVersion());
}
if (spec.getBatchWaitTime() != null) {
request.setBatchWaitTime(spec.getBatchWaitTime());
}
if (spec.getJdk() != null) {
request.setJDK(spec.getJdk());
} else {
request.setJDK(runningEnvironment.getJdk());
}
if (deployConfig.getSpec().getWebContainer() != null) {
request.setWebContainer(deployConfig.getSpec().getWebContainer());
} else {
request.setWebContainer(runningEnvironment.getWebContainer());
}
if (spec.getCommand() != null) {
request.setCommand(spec.getCommand());
}
if (spec.getCommandArgs() != null) {
request.setArgs(jsonMapper.writeValueAsString(spec.getCommandArgs()));
}
if (spec.getEnvs() != null) {
request.setEnvs(jsonMapper.writeValueAsString(spec.getEnvs()));
}
if (spec.getLiveness() != null) {
request.setLiveness(jsonMapper.writeValueAsString(spec.getLiveness()));
}
if (spec.getReadiness() != null) {
request.setReadiness(jsonMapper.writeValueAsString(spec.getReadiness()));
}
if (spec.getUpdateStrategy() != null) {
request.setUpdateStrategy(jsonMapper.writeValueAsString(spec.getUpdateStrategy()));
}
DeployK8sApplicationResponse response = defaultAcsClient.getAcsResponse(request);
if (response.getCode() == 200) {
String changeOrderId = response.getChangeOrderId();
logger.info("Deploy k8s application request sent, changeOrderId is: " + changeOrderId);
return changeOrderId;
} else {
String msg = String.format(
"Failed to send deploy k8s application request, requestId: %s, code: %d, msg: %s",
response.getRequestId(),
response.getCode(),
response.getMessage());
throw new Exception(msg);
}
}
public RunningEnvironment getK8sAppRunningEnvironment(String appId, DefaultAcsClient defaultAcsClient) throws Exception {
if (StringUtils.isBlank(appId)) {
throw new Exception("Application must be selected");
}
RunningEnvironment runningEnvironment = new RunningEnvironment();
GetK8sApplicationRequest request = new GetK8sApplicationRequest();
request.setAppId(appId);
request.setFrom("deploy");
GetK8sApplicationResponse response = defaultAcsClient.getAcsResponse(request);
if (response.getCode() == 200) {
if (response.getApplcation().getDeployGroups().size() > 0) {
for (GetK8sApplicationResponse.Applcation.DeployGroup.ComponentsItem componentsItem : response.getApplcation().getDeployGroups().get(0).getComponents()) {
if (componentsItem.getComponentKey().startsWith("Open JDK")) {
runningEnvironment.setJdk(componentsItem.getComponentKey());
}
}
}
if (response.getApplcation().getApp().getBuildpackId() == -1 && response.getApplcation().getApp().getApplicationType().equals(WAR)) {
if (response.getApplcation().getDeployGroups().size() > 0) {
for (GetK8sApplicationResponse.Applcation.DeployGroup.ComponentsItem componentsItem : response.getApplcation().getDeployGroups().get(0).getComponents()) {
if (componentsItem.getComponentKey().startsWith("apache-tomcat-")) {
runningEnvironment.setWebContainer(componentsItem.getComponentKey());
}
}
} else {
runningEnvironment.setWebContainer("apache-tomcat-7.0.91");
}
} else {
runningEnvironment.setWebContainer("");
}
return runningEnvironment;
} else {
throw new Exception(response.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy