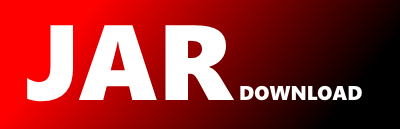
com.aliyun.mojo.ToolkitDeployMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toolkit-maven-plugin
Show all versions of toolkit-maven-plugin
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun.mojo;
import com.aliyun.bean.common.BuildProfile;
import com.aliyun.bean.common.OssProfile;
import com.aliyun.bean.common.ToolkitProfile;
import com.aliyun.bean.config.DefaultConfigBean;
import com.aliyun.bean.config.ToolkitPackageConfig;
import com.aliyun.bean.config.ToolkitDeployConfig;
import com.aliyun.enums.Constants;
import com.aliyun.exception.ErrMsg;
import com.aliyun.manager.*;
import com.aliyun.Context;
import com.aliyun.manager.config.DefaultConfigManager;
import com.aliyun.manager.config.ToolkitPackageConfigManager;
import com.aliyun.manager.config.ToolkitDeployConfigManager;
import com.aliyun.manager.config.ToolkitProfileConfigManager;
import com.aliyun.manager.deploy.ToolkitDeployManager;
import com.aliyun.utils.CommonUtils;
import com.aliyuncs.DefaultAcsClient;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.Strings;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import java.io.File;
import java.nio.file.Paths;
import java.util.List;
import static com.aliyun.enums.Constants.*;
@Mojo( name = "deploy", defaultPhase = LifecyclePhase.PACKAGE)
public class ToolkitDeployMojo extends AbstractMojo {
private static final String OLD_DEFAULT_DEPLOY_CONFIG_FILE = ".edas_config.yaml";
@Parameter(readonly = true, defaultValue = "${project}")
private MavenProject project;
/**
* path to profile
*/
@Parameter(property = "toolkit_profile")
private String toolkitProfile;
/**
* path to toolkit_create config file
*/
@Parameter(property = "toolkit_deploy")
private String toolkitDeploy;
/**
* path to toolkit_package file
*/
@Parameter(property = "toolkit_package")
private String toolkitPackage;
@Parameter(property = "edas_config")
private String configFile;
@Parameter(property = "access_key_id")
private String accessKeyId;
@Parameter(property = "access_key_secret")
private String accessKeySecret;
@Parameter(property = "access_key_file")
private String accessKeyFile;
@Parameter(property = "deploy_version")
private String deployVersion;
@Parameter(property = "deploy_desc")
private String deployDesc;
@Parameter(property = "jar_path")
private String jarPath;
@Parameter(property = "skip")
private String skip;
/*
* image_tag for docker build
* image_url for directly deployment
*/
@Parameter(property = "image_tag")
private String imageTag;
@Parameter(property = "image_url")
private String imageUrl;
@Parameter(property = "app_id")
private String appId;
/**
* indicate the artifacts which will be deployed. If there are more than one artifact to deploy,
* use comma to split.
* since 1.0.3
*/
@Parameter(property = "deploy_artifacts")
private String deployArtifacts;
@Override
public void execute() throws MojoExecutionException {
if ("true".equalsIgnoreCase(skip)) {
return;
}
Log logger = getLog();
Context.setLogger(logger);
Context.setProject(project);
/*
* 通过指定模块名的方式支持在父工程里打包部署子工程
* Added in 1.0.3
*/
if (! deployCurrentArtifact()) {
return;
}
loadProperties();
initAcsClient();
deploy();
}
private void deploy() throws MojoExecutionException {
try {
ToolkitDeployManager deployManager = new ToolkitDeployManager();
deployManager.deploy(Context.getToolkitDeployConfig(), Context.getToolkitPackageConfig());
} catch (Exception ex) {
getLog().error(ex.getMessage(), ex);
throw new MojoExecutionException("Failed to deploy: " + ex.getMessage());
}
}
private void initAcsClient() throws MojoExecutionException {
try {
DefaultAcsClient client = CommonUtils.initAcsClient(Context.getToolkitProfileConfig());
Context.setAcsClient(client);
} catch (Exception ex) {
String msg = "Failed to init acs client: " + ex.getMessage();
getLog().error(msg, ex);
throw new MojoExecutionException(msg);
}
}
private void transformAndSetContext(DefaultConfigBean oldConfig) throws Exception {
DefaultConfigBean.App app = oldConfig.getApp();
if (app == null) {
app = new DefaultConfigBean.App();
oldConfig.setApp(app);
}
DefaultConfigBean.Oss oss = oldConfig.getOss();
if (oss == null) {
oss = new DefaultConfigBean.Oss();
oldConfig.setOss(oss);
}
if (app.getAppId() == null && Strings.isNullOrEmpty(appId)) {
throw new Exception("app_id is not defined in config file.");
}
if (!Strings.isNullOrEmpty(appId)) {
app.setAppId(appId);
}
ToolkitDeployConfig deployConfig = new ToolkitDeployConfig();
deployConfig.setKind(Constants.APP_DEPLOYMENT);
ToolkitDeployConfig.Spec spec = new ToolkitDeployConfig.Spec();
if (CommonUtils.isNotEmpty(oldConfig.getApp()) && CommonUtils.isNotEmpty(oldConfig.getApp().getType())) {
spec.setType(oldConfig.getApp().getType());
} else {
spec.setType("edas");
}
spec.setTarget(new ToolkitDeployConfig.Target());
spec.getTarget().setAppId(app.getAppId());
//优先部署包配置参数-Ddeploy_version
if (!Strings.isNullOrEmpty(deployVersion)) {
spec.setVersion(deployVersion);
} else {
spec.setVersion(app.getPackageVersion());
}
spec.setBatchWaitTime(app.getBatchWaitTime());
//优先部署包配置参数-Ddeploy_desc
if (!Strings.isNullOrEmpty(deployDesc)) {
spec.setDesc(deployDesc);
} else {
spec.setDesc(app.getDesc());
}
spec.setGroupId(app.getGroupId());
spec.setBatch(app.getBatch());
if (app.getAppEnv() != null) {
//json
ObjectMapper jsonMapper = new ObjectMapper();
jsonMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
List envs = jsonMapper.readValue(
app.getAppEnv(), new TypeReference>(){});
spec.setEnvs(envs);
}
spec.setStageTimeout(app.getStageTimeout());
spec.setServiceStageTimeout(app.getServiceStageTimeout());
spec.setInstanceStageTimeout(app.getInstanceStageTimeout());
spec.setReleaseType(app.getReleaseType());
deployConfig.setSpec(spec);
Context.setToolkitDeployConfig(deployConfig);
//app entity
ToolkitPackageConfig packageConfig = new ToolkitPackageConfig();
packageConfig.setKind(APP_PACKAGE);
packageConfig.setSpec(new ToolkitPackageConfig.Spec());
packageConfig.getSpec().setImageUrl(app.getImageUrl());
if (imageUrl != null) {
packageConfig.getSpec().setImageUrl(imageUrl);
}
packageConfig.getSpec().setPackageUrl(app.getWarUrl());
if (app.getImageUrl() != null || imageUrl != null) {
packageConfig.getSpec().setPackageType(IMAGE);
} else {
if ("pom".equalsIgnoreCase(project.getPackaging())) {
throw new Exception(ErrMsg.getPomDeployErrMsg());
} else if ("jar".equalsIgnoreCase(project.getPackaging())) {
packageConfig.getSpec().setPackageType(FATJAR);
} else if ("war".equalsIgnoreCase(project.getPackaging())) {
packageConfig.getSpec().setPackageType(WAR);
} else {
throw new Exception("Unknown packaing: " + project.getPackaging());
}
}
packageConfig.getSpec().setBuild(new BuildProfile());
if (oss.getBucket() != null
|| oss.getUseVpcEndpoint() != null
|| oss.getUseHttps() != null
|| oss.getUseMultipartUpload() != null) {
OssProfile ossProfile = new OssProfile();
packageConfig.getSpec().getBuild().setOss(ossProfile);
ossProfile.setBucket(oss.getBucket());
ossProfile.setRegionId(oss.getRegionId());
ossProfile.setAccessKeyId(oss.getAccessKeyId());
ossProfile.setAccessKeySecret(oss.getAccessKeySecret());
ossProfile.setKey(oss.getKey());
ossProfile.setUseVpcEndpoint(oss.getUseVpcEndpoint());
ossProfile.setUseHttps(oss.getUseHttps());
ossProfile.setUseMultipartUpload(oss.getUseMultipartUpload());
}
Context.setToolkitPackageConfig(packageConfig);
}
private boolean deployCurrentArtifact() {
if (StringUtils.isNotBlank(deployArtifacts)) {
boolean target = false;
for (String artifact: deployArtifacts.split(",")) {
if (artifact.equals(project.getArtifactId())) {
target = true;
break;
}
}
if (!target) {
return false;
}
}
return true;
}
private void loadProperties() throws MojoExecutionException {
try {
File baseDir = project.getBasedir();
//将相对文件路径根据baseDir转换为绝对文件路径
transformConfigFilePath(baseDir.getAbsolutePath());
//load deployment
String newDefaultDeployFile = baseDir.getPath() + CommonUtils.getFileSeparator() + TOOLKIT_DEFAULT_DEPLOY_CONFIG;
if (toolkitDeploy != null || new File(newDefaultDeployFile).exists()) {
ToolkitDeployConfigManager deployConfigManager = new ToolkitDeployConfigManager();
ToolkitDeployConfig deployConfig =
deployConfigManager.loadProperties(toolkitDeploy, newDefaultDeployFile);
// 优先-Ddeploy_version
if (!Strings.isNullOrEmpty(deployVersion)) {
deployConfig.getSpec().setVersion(deployVersion);
}
// 优先-Ddeploy_desc
if (!Strings.isNullOrEmpty(deployDesc)) {
deployConfig.getSpec().setDesc(deployDesc);
}
// 优先-Dapp_id
if (!Strings.isNullOrEmpty(appId)) {
if (deployConfig.getSpec().getTarget() == null) {
deployConfig.getSpec().setTarget(new ToolkitDeployConfig.Target());
}
deployConfig.getSpec().getTarget().setAppId(appId);
}
Context.setToolkitDeployConfig(deployConfig);
//load package
String defaultPackageFile =
baseDir.getPath() + CommonUtils.getFileSeparator() + TOOLKIT_DEFAULT_PACKAGE_CONFIG;
ToolkitPackageConfigManager packageConfigManager = new ToolkitPackageConfigManager();
ToolkitPackageConfig packageConfig =
packageConfigManager.loadProperties(toolkitPackage, defaultPackageFile);
if (packageConfig.getSpec().getBuild() == null) {
packageConfig.getSpec().setBuild(new BuildProfile());
}
// 优先-Dimage_tag
if (!Strings.isNullOrEmpty(imageTag)) {
packageConfig.getSpec().getBuild().getDocker().setImageTag(imageTag);
}
// 优先-Dimage_url
if (!Strings.isNullOrEmpty(imageUrl)) {
packageConfig.getSpec().setImageUrl(imageUrl);
}
Context.setToolkitPackageConfig(packageConfig);
} else {
String oldDefaultDeployFile = baseDir.getPath() + CommonUtils.getFileSeparator() + OLD_DEFAULT_DEPLOY_CONFIG_FILE;
DefaultConfigManager defaultConfigManager = new DefaultConfigManager();
DefaultConfigBean oldConfig = defaultConfigManager.loadProperties(configFile, oldDefaultDeployFile);
transformAndSetContext(oldConfig);
}
//load profile
ToolkitProfileConfigManager profileConfigManager = new ToolkitProfileConfigManager();
ToolkitProfile profile = null;
String defaultProfile = baseDir.getPath() + CommonUtils.getFileSeparator() + TOOLKIT_DEFAULT_PROFILE;
if (toolkitProfile != null || new File(defaultProfile).exists()) {
profile = profileConfigManager.loadProperties(
toolkitProfile, defaultProfile, accessKeyId, accessKeySecret);
}
//load profile
if (profile == null) {
if ((toolkitDeploy == null && !(new File(newDefaultDeployFile).exists()))) {
//fallback to old way
String defaultConfigFile = baseDir.getPath() + CommonUtils.getFileSeparator() + OLD_DEFAULT_DEPLOY_CONFIG_FILE;
LoadConfigManager loadConfigManager = new LoadConfigManager();
DefaultConfigBean oldProfileBean =
loadConfigManager.loadProperties(
configFile,
defaultConfigFile,
accessKeyFile, accessKeyId, accessKeySecret);
profile = new ToolkitProfile();
DefaultConfigBean.Env env = oldProfileBean.getEnv();
profile.setAccessKeyId(env.getAccessKeyId());
profile.setAccessKeySecret(env.getAccessKeySecret());
profile.setRegionId(env.getRegionId());
profile.setEndpoint(env.getEndpoint());
profile.setJarPath(env.getJarPath());
if (profile.getEndpoint() == null) {
profile.setEndpoint("edas." + env.getRegionId() + ".aliyuncs.com");
}
} else {
throw new Exception("No toolkit profile is found for deploy.");
}
}
// 重置jarPath
if (StringUtils.isNotBlank(jarPath)) {
profile.setJarPath(jarPath);
}
if (StringUtils.isNotBlank(profile.getJarPath())) {
profile.setJarPath(transformPathToAbsolutePath(baseDir.getAbsolutePath(), profile.getJarPath()));
}
Context.setToolkitProfileConfig(profile);
} catch (Exception ex) {
String msg = "Failed to load config file: " + ex.getMessage();
//getLog().error(msg, ex);
throw new MojoExecutionException(msg);
}
}
private String transformPathToAbsolutePath(String baseDir, String path) {
if (StringUtils.isNotBlank(path) && !Paths.get(path).isAbsolute()) {
return baseDir + File.separator + path;
}
return path;
}
private void transformConfigFilePath(String baseDir) {
toolkitProfile = transformPathToAbsolutePath(baseDir, toolkitProfile);
toolkitDeploy = transformPathToAbsolutePath(baseDir, toolkitDeploy);
toolkitPackage = transformPathToAbsolutePath(baseDir, toolkitPackage);
configFile = transformPathToAbsolutePath(baseDir, configFile);
accessKeyFile = transformPathToAbsolutePath(baseDir, accessKeyFile);
accessKeyFile = transformPathToAbsolutePath(baseDir, accessKeyFile);
jarPath = transformPathToAbsolutePath(baseDir, jarPath);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy