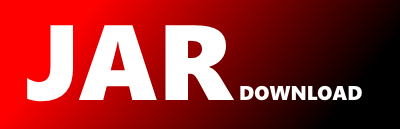
com.alibaba.csp.ahas.sentinel.acm.InnerCloudAhasInitService Maven / Gradle / Ivy
/*
* Copyright 1999-2020 Alibaba Group Holding Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.csp.ahas.sentinel.acm;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.alibaba.csp.ahas.sentinel.datasource.parser.ApplicationClusterInfoParser;
import com.alibaba.csp.ahas.sentinel.datasource.parser.cluster.ClusterAssignStateParser;
import com.alibaba.csp.ahas.sentinel.datasource.parser.cluster.ClusterClientAssignConfigParser;
import com.alibaba.csp.ahas.sentinel.datasource.parser.cluster.ClusterClientCommonConfigParser;
import com.alibaba.csp.ahas.sentinel.datasource.parser.setting.SentinelAdapterSettingParser;
import com.alibaba.csp.ahas.sentinel.datasource.parser.setting.SentinelAdaptiveFlowSettingParser;
import com.alibaba.csp.ahas.sentinel.datasource.parser.setting.SentinelGeneralSettingParser;
import com.alibaba.csp.sentinel.adaptive.config.AdaptiveFlowSettingEntity;
import com.alibaba.csp.sentinel.adaptive.config.AdaptiveFlowSettingManager;
import com.alibaba.csp.sentinel.cluster.ClusterStateManager;
import com.alibaba.csp.sentinel.cluster.client.auth.ClientAuthConfigRegistry;
import com.alibaba.csp.sentinel.cluster.client.auth.ClientCredentialSupplier;
import com.alibaba.csp.sentinel.cluster.client.config.ClusterClientAssignConfig;
import com.alibaba.csp.sentinel.cluster.client.config.ClusterClientConfig;
import com.alibaba.csp.sentinel.cluster.client.config.ClusterClientConfigManager;
import com.alibaba.csp.sentinel.cluster.registry.ConfigSupplierRegistry;
import com.alibaba.csp.sentinel.datasource.Converter;
import com.alibaba.csp.sentinel.datasource.ReadableDataSource;
import com.alibaba.csp.sentinel.datasource.acm.InternalDiamondDataSource;
import com.alibaba.csp.sentinel.datasource.acm.parser.RetryRuleListParser;
import com.alibaba.csp.sentinel.log.RecordLog;
import com.alibaba.csp.sentinel.machine.MachineGroupEntity;
import com.alibaba.csp.sentinel.machine.MachineGroupManager;
import com.alibaba.csp.sentinel.node.IntervalProperty;
import com.alibaba.csp.sentinel.node.OccupyTimeoutProperty;
import com.alibaba.csp.sentinel.node.SampleCountProperty;
import com.alibaba.csp.sentinel.retry.RetryRule;
import com.alibaba.csp.sentinel.retry.RetryRuleManager;
import com.alibaba.csp.sentinel.setting.adapter.AdapterSettingManager;
import com.alibaba.csp.sentinel.setting.adapter.SentinelAdapterSettingEntity;
import com.alibaba.csp.sentinel.setting.general.GeneralSettingManager;
import com.alibaba.csp.sentinel.setting.general.SentinelGeneralSettingEntity;
import com.alibaba.csp.sentinel.slots.block.authority.AuthorityRule;
import com.alibaba.csp.sentinel.slots.block.authority.AuthorityRuleManager;
import com.alibaba.csp.sentinel.slots.block.degrade.DegradeRule;
import com.alibaba.csp.sentinel.slots.block.degrade.DegradeRuleManager;
import com.alibaba.csp.sentinel.slots.block.flow.FlowRule;
import com.alibaba.csp.sentinel.slots.block.flow.FlowRuleManager;
import com.alibaba.csp.sentinel.slots.block.flow.param.ParamFlowRule;
import com.alibaba.csp.sentinel.slots.block.flow.param.ParamFlowRuleManager;
import com.alibaba.csp.sentinel.slots.stresstest.StressingTestModeConfigManager;
import com.alibaba.csp.sentinel.slots.system.SystemRule;
import com.alibaba.csp.sentinel.slots.system.SystemRuleManager;
import com.alibaba.csp.sentinel.util.AppNameUtil;
import com.alibaba.csp.sentinel.util.function.Supplier;
import com.alibaba.fastjson.JSONObject;
import com.taobao.csp.ahas.auth.api.AuthUtil;
import com.taobao.csp.ahas.service.api.client.ClientInfoService;
import com.taobao.csp.ahas.service.component.AgwProductCode;
import com.taobao.csp.sentinel.datasource.LegacyAuthorityRuleParser;
import com.taobao.csp.sentinel.datasource.LegacyDegradeRuleParser;
import com.taobao.csp.sentinel.datasource.LegacyFlowRuleParser;
import com.taobao.csp.sentinel.datasource.LegacyParamFlowRuleParser;
import com.taobao.csp.sentinel.datasource.LegacySystemRuleParser;
import com.taobao.diamond.exception.DiamondException;
import static com.taobao.csp.sentinel.datasource.InternalSentinelDiamondConstants.*;
/**
* @author Eric Zhao
*/
public class InnerCloudAhasInitService extends AbstractSentinelSdkInitService {
public InnerCloudAhasInitService(ClientInfoService clientInfoService) {
super(clientInfoService);
}
@Override
protected void init0(String sdkVersion) throws Exception {
RecordLog.info("AHAS Sentinel is running in internal mode");
initClusterFlow(sdkVersion);
initDiamondDataSource();
}
private void initClusterFlow(final String sdkVersion) {
// TODO: be careful: handle transport failure
final String userId = clientInfoService.getUserId();
final String ahasNamespace = clientInfoService.getNamespace();
ConfigSupplierRegistry.setNamespaceSupplier(new Supplier() {
@Override
public String get() {
return userId + "|" + ahasNamespace + "|" + clientInfoService.getAppName();
}
});
ClientAuthConfigRegistry.setClientCredentialSupplier(new ClientCredentialSupplier() {
@Override
public Map getCredentials() {
Map map = new HashMap(8);
map.put("uid", userId);
map.put("ahasNs", ahasNamespace);
map.put("sk", AuthUtil.getSecretKey());
map.put("instanceId", clientInfoService.getAid(AgwProductCode.SENTINEL));
map.put("clientType", "JAVA_SDK");
map.put("clientVersion", sdkVersion);
map.put("cpbId", String.valueOf(1));
return map;
}
});
// TODO: SSL cert
}
private void initDiamondDataSource() {
try {
final String appName = AppNameUtil.getAppName();
if (appName == null) {
RecordLog.warn("[InnerCloudAhasInitService] appName is null, can not init Sentinel data-source");
return;
}
initCommonRuleDs(appName);
initApplicationClusterInfoDs(appName);
initSentinelClusterDs(appName);
initSettingDs(appName);
initInternalSettingDs(appName);
RecordLog.info("[InnerCloudAhasInitService] Sentinel Diamond data-source successfully initialized");
} catch (Throwable e) {
RecordLog.error("[InnerCloudAhasInitService] Failed to initialize Diamond data-source", e);
}
}
private void initCommonRuleDs(String appName) throws DiamondException {
ReadableDataSource> flowRuleDataSource = new InternalDiamondDataSource>(
appName + FLOW_SUFFIX, DIAMOND_GROUP, new LegacyFlowRuleParser());
FlowRuleManager.register2Property(flowRuleDataSource.getProperty());
ReadableDataSource> degradeDataSource
= new InternalDiamondDataSource>(
appName + DEGRADE_SUFFIX, DIAMOND_GROUP, new LegacyDegradeRuleParser());
DegradeRuleManager.register2Property(degradeDataSource.getProperty());
ReadableDataSource> authorityDS
= new InternalDiamondDataSource>(
appName + AUTHORITY_SUFFIX, DIAMOND_GROUP, new LegacyAuthorityRuleParser());
AuthorityRuleManager.register2Property(authorityDS.getProperty());
ReadableDataSource> systemRuleDataSource
= new InternalDiamondDataSource>(
appName + SYSTEM_SUFFIX, DIAMOND_GROUP, new LegacySystemRuleParser());
SystemRuleManager.register2Property(systemRuleDataSource.getProperty());
ReadableDataSource> paramFlowDataSource
= new InternalDiamondDataSource>(
appName + HOT_PARAM_SUFFIX, DIAMOND_GROUP, new LegacyParamFlowRuleParser(HOT_ITEM_PREFIX, DIAMOND_GROUP));
ParamFlowRuleManager.register2Property(paramFlowDataSource.getProperty());
ReadableDataSource> retryRuleRuleDataSource
= new InternalDiamondDataSource>(
appName + RETRY_RULE_DATA_ID_SUFFIX, DIAMOND_GROUP, new RetryRuleListParser());
RetryRuleManager.getInstance().register2Property(retryRuleRuleDataSource.getProperty());
}
private void initSentinelClusterDs(String appName) {
String assignMapDataId = appName + CLUSTER_ASSIGN_MAP;
// Init token client related data source.
ReadableDataSource clientAssignDs
= new InternalDiamondDataSource(
assignMapDataId, DIAMOND_GROUP, new ClusterClientAssignConfigParser());
ClusterClientConfigManager.registerServerAssignProperty(clientAssignDs.getProperty());
String clientConfigDataId = appName + CLUSTER_TOKEN_CLIENT_CONFIG_DATA_ID_SUFFIX;
ReadableDataSource clientConfigDs
= new InternalDiamondDataSource(clientConfigDataId, DIAMOND_GROUP,
new ClusterClientCommonConfigParser());
ClusterClientConfigManager.registerClientConfigProperty(clientConfigDs.getProperty());
// Init cluster state property for extracting mode from cluster map data source.
ReadableDataSource clusterStateDs = new InternalDiamondDataSource(
assignMapDataId, DIAMOND_GROUP, new ClusterAssignStateParser());
ClusterStateManager.registerProperty(clusterStateDs.getProperty());
}
private void initSettingDs(String appName) {
// We could register the observer for adapter settings here.
// For example, we could leverage the consumer to update the configuration of Web URL prefix cleaner.
String gsDataId = appName + GENERAL_SETTING_DATA_ID_SUFFIX;
InternalDiamondDataSource generalSettingDs
= new InternalDiamondDataSource(gsDataId, DIAMOND_GROUP,
new SentinelGeneralSettingParser());
GeneralSettingManager.register2Property(generalSettingDs.getProperty());
String asDataId = appName + ADAPTER_SETTING_DATA_ID_SUFFIX;
InternalDiamondDataSource adapterSettingDs
= new InternalDiamondDataSource(
asDataId, DIAMOND_GROUP, new SentinelAdapterSettingParser());
AdapterSettingManager.register2Property(adapterSettingDs.getProperty());
String afSettingDataId = appName + ADAPTIVE_FLOW_SETTING_DATA_ID_SUFFIX;
InternalDiamondDataSource adaptiveFlowSettingDs
= new InternalDiamondDataSource(
afSettingDataId, DIAMOND_GROUP, new SentinelAdaptiveFlowSettingParser());
AdaptiveFlowSettingManager.register2Property(adaptiveFlowSettingDs.getProperty());
}
private void initInternalSettingDs(String appName) {
// interval of the total sliding window (in second)
ReadableDataSource flowIntervalDs = new InternalDiamondDataSource(
appName + FLOW_INTERVAL,
DIAMOND_GROUP, new Converter() {
@Override
public Integer convert(String source) {
Integer v = JSONObject.parseObject(source, Integer.class);
// convert seconds to milliseconds.
if (v != null) {
v = v * 1000;
}
return v;
}
});
IntervalProperty.register2Property(flowIntervalDs.getProperty());
ReadableDataSource sampleCountDs = new InternalDiamondDataSource(
appName + SAMPLE_COUNT,
DIAMOND_GROUP, jsonIntParser);
SampleCountProperty.register2Property(sampleCountDs.getProperty());
ReadableDataSource stressTestModeConfigDs = new InternalDiamondDataSource(
appName + STRESSING_TEST,
DIAMOND_GROUP, new Converter() {
@Override
public Boolean convert(String s) {
return JSONObject.parseObject(s, Boolean.class);
}
});
StressingTestModeConfigManager.register2Property(stressTestModeConfigDs.getProperty());
ReadableDataSource priorityTimeoutConfigDs = new InternalDiamondDataSource(
appName + PRIORITY_TIMEOUT,
DIAMOND_GROUP, jsonIntParser);
OccupyTimeoutProperty.register2Property(priorityTimeoutConfigDs.getProperty());
}
private void initApplicationClusterInfoDs(String appName) {
// 应用集群的基本状态信息(如在线机器数).
String machineGroupDataId = appName + APP_CLUSTER_INFO_DATA_ID_SUFFIX;
InternalDiamondDataSource clusterInfoDs = new InternalDiamondDataSource(
machineGroupDataId, DIAMOND_GROUP, new ApplicationClusterInfoParser());
MachineGroupManager.register2Property(clusterInfoDs.getProperty());
}
private final Converter jsonIntParser = new Converter() {
@Override
public Integer convert(String s) {
return JSONObject.parseObject(s, Integer.class);
}
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy