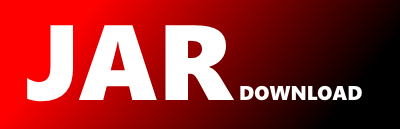
com.alibaba.csp.ahas.switchcenter.util.HttpRequestEvent Maven / Gradle / Ivy
package com.alibaba.csp.ahas.switchcenter.util;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.net.Socket;
import java.net.URLDecoder;
import java.nio.charset.Charset;
import java.util.HashMap;
import java.util.Map;
import com.alibaba.acm.shaded.com.alibaba.metrics.StringUtils;
import com.alibaba.csp.ahas.switchcenter.AhasHttpRequestHandler;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONException;
import com.taobao.csp.switchcenter.command.CommandHandler;
import com.taobao.csp.switchcenter.command.CommandRequest;
import com.taobao.csp.switchcenter.command.CommandResponse;
import com.taobao.csp.switchcenter.log.SwitchRecordLog;
import com.taobao.csp.switchcenter.util.StringUtil;
import com.taobao.csp.switchcenter.util.SwitchUtil;
/**
* @author lixin.lb
*/
public class HttpRequestEvent implements Runnable{
private static final String DEFAULT_CHARSET = System.getProperty("csp.server.charset", "GBK");
private Socket socket;
public HttpRequestEvent(Socket socket) {
this.socket = socket;
}
@Override
public void run() {
if (socket == null) {
return;
}
BufferedReader in = null;
PrintWriter out = null;
try {
in = new BufferedReader(new InputStreamReader(socket.getInputStream(), DEFAULT_CHARSET));
OutputStream outputStream = socket.getOutputStream();
out = new PrintWriter(new OutputStreamWriter(outputStream, Charset.forName(DEFAULT_CHARSET)));
String line = in.readLine();
Request request = parseRequest(line);
out.print("HTTP/1.1 200 OK\r\n\r\n");
out.flush();
if (StringUtils.isBlank(request.getTarget())) {
out.println("query paramater is blank");
out.flush();
return;
}
CommandHandler command = AhasHttpRequestHandler.getCommand(request.getTarget());
if (null != command) {
PrintWriter printOut = SwitchUtil.getPrintWriter(outputStream);
CommandRequest commandRequest = convertRequest(request);
CommandResponse commandResponse = command.handle(commandRequest);
printOut.write(commandResponse.getResult());
printOut.flush();
} else {
out.println("query paramater is incorrcet");
}
out.flush();
SwitchRecordLog.info("[CommandCenter] deal a socket task:" + line + "," + socket.getInetAddress());
} catch (Throwable e) {
SwitchRecordLog.info("CommandCenter error", e);
try {
if (out != null){
out.println("CommandCenter failed, message is " + e.getMessage());
out.flush();
}
} catch (Exception e1) {
SwitchRecordLog.info("CommandCenter close serverSocket failed", e);
}
} finally {
try {
if (out != null){
out.close();
}
if (in != null){
in.close();
}
socket.close();
} catch (Exception e) {
SwitchRecordLog.info("CommandCenter close resource failed", e);
}
}
}
/**
* @param line
* @return
*/
private Request parseRequest(String line) {
Request request = new Request();
if (StringUtils.isBlank(line)) {
return request;
}
int start = line.indexOf('/');
int ask = line.indexOf('?') == -1 ? line.lastIndexOf(' ') : line.indexOf('?');
int space = line.lastIndexOf(' ');
String target = line.substring(start != -1 ? start + 1 : 0, ask != -1 ? ask : line.length());
request.setTarget(target);
if (ask == -1 || ask == space) {
return request;
}
String parameterStr = line.substring(ask != -1 ? ask + 1 : 0, space != -1 ? space : line.length());
for (String parameter : parameterStr.split("&")) {
if (StringUtils.isBlank(parameter)) {
continue;
}
String[] key_value = parameter.split("=");
if (key_value.length != 2) {
continue;
}
String value = StringUtil.trim(key_value[1]);
try {
value = URLDecoder.decode(value, DEFAULT_CHARSET);
} catch (UnsupportedEncodingException e) {
}
request.addParameter(StringUtil.trim(key_value[0]), value);
}
return request;
}
private com.taobao.csp.switchcenter.command.CommandRequest convertRequest(Request request) {
com.taobao.csp.switchcenter.command.CommandRequest commandRequest = new com.taobao.csp.switchcenter.command.CommandRequest();
if (request == null) {
return commandRequest;
}
commandRequest.getParameters().putAll(request.getParameters());
return commandRequest;
}
class Request {
private String target;
private Map parameters = new HashMap();
String getTarget() {
return target;
}
void setTarget(String target) {
this.target = target;
}
Map getParameters() {
return parameters;
}
String getParameter(String key) {
return getParameters().get(key);
}
public String getParameter(String key, String defaultValue) {
String value = getParameter(key);
return StringUtil.isBlank(value) ? defaultValue : value;
}
void addParameter(String key, String value) {
this.parameters.put(key, value);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy