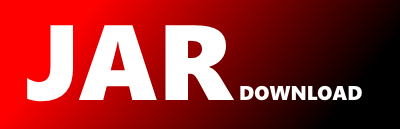
com.alibaba.csp.ahas.switchcenter.SwitchAutoConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-ahas-switch-client Show documentation
Show all versions of spring-boot-starter-ahas-switch-client Show documentation
The parent project of AHAS switch starter
/*
* Copyright 1999-2019 Alibaba Group Holding Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.csp.ahas.switchcenter;
import java.util.Iterator;
import org.springframework.aop.support.AopUtils;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.config.BeanFactoryPostProcessor;
import org.springframework.beans.factory.config.BeanPostProcessor;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.Ordered;
import org.springframework.core.PriorityOrdered;
import org.springframework.core.annotation.AnnotationUtils;
import org.springframework.util.StringUtils;
import com.alibaba.csp.ahas.switchcenter.anotation.Switch;
import com.alibaba.csp.ahas.switchcenter.anotation.SwitchListener;
import com.taobao.csp.switchcenter.core.Listener;
import com.taobao.csp.switchcenter.core.SwitchManager;
import com.taobao.csp.switchcenter.log.SwitchRecordLog;
@Configuration
@ConditionalOnProperty(name = "ahas.switch.enabled", matchIfMissing = true)
public class SwitchAutoConfiguration
implements BeanPostProcessor, BeanFactoryPostProcessor, ApplicationContextAware, PriorityOrdered {
private final String appNamePropertyKey = "project.name";
private final String namespacePropertyKey = "ahas.namespace";
private final String licensePropertyKey = "ahas.license";
private String appName;
private ApplicationContext applicationContext;
@Override
public void postProcessBeanFactory(ConfigurableListableBeanFactory beanFactory) throws BeansException {
if (beanFactory == null) {
return;
}
Iterator iterator = beanFactory.getBeanNamesIterator();
while (iterator.hasNext()) {
String beanName = iterator.next();
BeanDefinition beanDefinition;
try {
beanDefinition = beanFactory.getBeanDefinition(beanName);
} catch (NoSuchBeanDefinitionException e) {
continue;
}
String beanClassName = beanDefinition.getBeanClassName();
if (StringUtils.isEmpty(beanClassName)) {
continue;
}
try {
Class> beanClass = Class.forName(beanClassName);
Switch switchAnotation = (Switch) AnnotationUtils.findAnnotation(beanClass, Switch.class);
if (switchAnotation != null) {
String appName = this.appName;
if (!switchAnotation.appName().equals("default_appName")) {
appName = switchAnotation.appName();
}
if (StringUtils.isEmpty(appName)) {
SwitchManager.init(beanClass);
} else {
SwitchManager.init(appName, beanClass);
}
}
} catch (ClassNotFoundException e) {
SwitchRecordLog.warn("[SwitchCenter starter] beanClass {0}, not found", beanClassName);
} catch (Throwable e) {
System.out.println(beanClassName + " " + e.getStackTrace());
}
}
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
Class> beanClass = AopUtils.getTargetClass(bean);
SwitchListener switchListener = (SwitchListener) AnnotationUtils.findAnnotation(beanClass,
SwitchListener.class);
if (switchListener != null) {
if (!(bean instanceof Listener))
throw new IllegalArgumentException(
AopUtils.getTargetClass(bean).getName() + " not a instance of " + Listener.class.getName());
SwitchManager.addListener((Listener) bean);
}
return bean;
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.applicationContext = applicationContext;
this.appName = this.applicationContext.getEnvironment().getProperty(appNamePropertyKey);
if (appName != null) {
System.setProperty(appNamePropertyKey, appName);
}
String namespace = this.applicationContext.getEnvironment().getProperty(namespacePropertyKey);
if (namespace != null) {
System.setProperty(namespacePropertyKey, namespace);
}
String license = this.applicationContext.getEnvironment().getProperty(licensePropertyKey);
if (license != null) {
System.setProperty(licensePropertyKey, license);
}
}
@Override
public int getOrder() {
return Ordered.HIGHEST_PRECEDENCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy