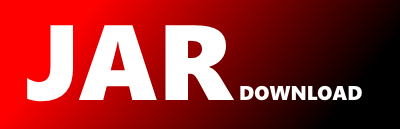
com.alibaba.fluss.protogen.tests.Bytes Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: bytes.proto
// Protobuf Java Version: 3.25.5
package com.alibaba.fluss.protogen.tests;
public final class Bytes {
private Bytes() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface BOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.alibaba.fluss.protogen.tests.B)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes payload = 1;
* @return Whether the payload field is set.
*/
boolean hasPayload();
/**
* optional bytes payload = 1;
* @return The payload.
*/
com.google.protobuf.ByteString getPayload();
/**
* repeated bytes extra_items = 2;
* @return A list containing the extraItems.
*/
java.util.List getExtraItemsList();
/**
* repeated bytes extra_items = 2;
* @return The count of extraItems.
*/
int getExtraItemsCount();
/**
* repeated bytes extra_items = 2;
* @param index The index of the element to return.
* @return The extraItems at the given index.
*/
com.google.protobuf.ByteString getExtraItems(int index);
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.B}
*/
public static final class B extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.alibaba.fluss.protogen.tests.B)
BOrBuilder {
private static final long serialVersionUID = 0L;
// Use B.newBuilder() to construct.
private B(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private B() {
payload_ = com.google.protobuf.ByteString.EMPTY;
extraItems_ = emptyList(com.google.protobuf.ByteString.class);
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new B();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_B_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_B_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.B.class, com.alibaba.fluss.protogen.tests.Bytes.B.Builder.class);
}
private int bitField0_;
public static final int PAYLOAD_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes payload = 1;
* @return Whether the payload field is set.
*/
@java.lang.Override
public boolean hasPayload() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes payload = 1;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
public static final int EXTRA_ITEMS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.ProtobufList extraItems_ =
emptyList(com.google.protobuf.ByteString.class);
/**
* repeated bytes extra_items = 2;
* @return A list containing the extraItems.
*/
@java.lang.Override
public java.util.List
getExtraItemsList() {
return extraItems_;
}
/**
* repeated bytes extra_items = 2;
* @return The count of extraItems.
*/
public int getExtraItemsCount() {
return extraItems_.size();
}
/**
* repeated bytes extra_items = 2;
* @param index The index of the element to return.
* @return The extraItems at the given index.
*/
public com.google.protobuf.ByteString getExtraItems(int index) {
return extraItems_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, payload_);
}
for (int i = 0; i < extraItems_.size(); i++) {
output.writeBytes(2, extraItems_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, payload_);
}
{
int dataSize = 0;
for (int i = 0; i < extraItems_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(extraItems_.get(i));
}
size += dataSize;
size += 1 * getExtraItemsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.alibaba.fluss.protogen.tests.Bytes.B)) {
return super.equals(obj);
}
com.alibaba.fluss.protogen.tests.Bytes.B other = (com.alibaba.fluss.protogen.tests.Bytes.B) obj;
if (hasPayload() != other.hasPayload()) return false;
if (hasPayload()) {
if (!getPayload()
.equals(other.getPayload())) return false;
}
if (!getExtraItemsList()
.equals(other.getExtraItemsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPayload()) {
hash = (37 * hash) + PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getPayload().hashCode();
}
if (getExtraItemsCount() > 0) {
hash = (37 * hash) + EXTRA_ITEMS_FIELD_NUMBER;
hash = (53 * hash) + getExtraItemsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.B parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.alibaba.fluss.protogen.tests.Bytes.B prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.B}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.alibaba.fluss.protogen.tests.B)
com.alibaba.fluss.protogen.tests.Bytes.BOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_B_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_B_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.B.class, com.alibaba.fluss.protogen.tests.Bytes.B.Builder.class);
}
// Construct using com.alibaba.fluss.protogen.tests.Bytes.B.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
payload_ = com.google.protobuf.ByteString.EMPTY;
extraItems_ = emptyList(com.google.protobuf.ByteString.class);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_B_descriptor;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.B getDefaultInstanceForType() {
return com.alibaba.fluss.protogen.tests.Bytes.B.getDefaultInstance();
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.B build() {
com.alibaba.fluss.protogen.tests.Bytes.B result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.B buildPartial() {
com.alibaba.fluss.protogen.tests.Bytes.B result = new com.alibaba.fluss.protogen.tests.Bytes.B(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.alibaba.fluss.protogen.tests.Bytes.B result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.payload_ = payload_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
extraItems_.makeImmutable();
result.extraItems_ = extraItems_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alibaba.fluss.protogen.tests.Bytes.B) {
return mergeFrom((com.alibaba.fluss.protogen.tests.Bytes.B)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alibaba.fluss.protogen.tests.Bytes.B other) {
if (other == com.alibaba.fluss.protogen.tests.Bytes.B.getDefaultInstance()) return this;
if (other.hasPayload()) {
setPayload(other.getPayload());
}
if (!other.extraItems_.isEmpty()) {
if (extraItems_.isEmpty()) {
extraItems_ = other.extraItems_;
extraItems_.makeImmutable();
bitField0_ |= 0x00000002;
} else {
ensureExtraItemsIsMutable();
extraItems_.addAll(other.extraItems_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
payload_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.google.protobuf.ByteString v = input.readBytes();
ensureExtraItemsIsMutable();
extraItems_.add(v);
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes payload = 1;
* @return Whether the payload field is set.
*/
@java.lang.Override
public boolean hasPayload() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes payload = 1;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
/**
* optional bytes payload = 1;
* @param value The payload to set.
* @return This builder for chaining.
*/
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
payload_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional bytes payload = 1;
* @return This builder for chaining.
*/
public Builder clearPayload() {
bitField0_ = (bitField0_ & ~0x00000001);
payload_ = getDefaultInstance().getPayload();
onChanged();
return this;
}
private com.google.protobuf.Internal.ProtobufList extraItems_ = emptyList(com.google.protobuf.ByteString.class);
private void ensureExtraItemsIsMutable() {
if (!extraItems_.isModifiable()) {
extraItems_ = makeMutableCopy(extraItems_);
}
bitField0_ |= 0x00000002;
}
/**
* repeated bytes extra_items = 2;
* @return A list containing the extraItems.
*/
public java.util.List
getExtraItemsList() {
extraItems_.makeImmutable();
return extraItems_;
}
/**
* repeated bytes extra_items = 2;
* @return The count of extraItems.
*/
public int getExtraItemsCount() {
return extraItems_.size();
}
/**
* repeated bytes extra_items = 2;
* @param index The index of the element to return.
* @return The extraItems at the given index.
*/
public com.google.protobuf.ByteString getExtraItems(int index) {
return extraItems_.get(index);
}
/**
* repeated bytes extra_items = 2;
* @param index The index to set the value at.
* @param value The extraItems to set.
* @return This builder for chaining.
*/
public Builder setExtraItems(
int index, com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureExtraItemsIsMutable();
extraItems_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* repeated bytes extra_items = 2;
* @param value The extraItems to add.
* @return This builder for chaining.
*/
public Builder addExtraItems(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureExtraItemsIsMutable();
extraItems_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* repeated bytes extra_items = 2;
* @param values The extraItems to add.
* @return This builder for chaining.
*/
public Builder addAllExtraItems(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureExtraItemsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, extraItems_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* repeated bytes extra_items = 2;
* @return This builder for chaining.
*/
public Builder clearExtraItems() {
extraItems_ = emptyList(com.google.protobuf.ByteString.class);
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.alibaba.fluss.protogen.tests.B)
}
// @@protoc_insertion_point(class_scope:com.alibaba.fluss.protogen.tests.B)
private static final com.alibaba.fluss.protogen.tests.Bytes.B DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.alibaba.fluss.protogen.tests.Bytes.B();
}
public static com.alibaba.fluss.protogen.tests.Bytes.B getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public B parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.B getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RDOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.alibaba.fluss.protogen.tests.RD)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes records = 1;
* @return Whether the records field is set.
*/
boolean hasRecords();
/**
* optional bytes records = 1;
* @return The records.
*/
com.google.protobuf.ByteString getRecords();
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.RD}
*/
public static final class RD extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.alibaba.fluss.protogen.tests.RD)
RDOrBuilder {
private static final long serialVersionUID = 0L;
// Use RD.newBuilder() to construct.
private RD(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RD() {
records_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RD();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RD_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RD_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.RD.class, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder.class);
}
private int bitField0_;
public static final int RECORDS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString records_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes records = 1;
* @return Whether the records field is set.
*/
@java.lang.Override
public boolean hasRecords() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes records = 1;
* @return The records.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRecords() {
return records_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, records_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, records_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.alibaba.fluss.protogen.tests.Bytes.RD)) {
return super.equals(obj);
}
com.alibaba.fluss.protogen.tests.Bytes.RD other = (com.alibaba.fluss.protogen.tests.Bytes.RD) obj;
if (hasRecords() != other.hasRecords()) return false;
if (hasRecords()) {
if (!getRecords()
.equals(other.getRecords())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRecords()) {
hash = (37 * hash) + RECORDS_FIELD_NUMBER;
hash = (53 * hash) + getRecords().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.alibaba.fluss.protogen.tests.Bytes.RD prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.RD}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.alibaba.fluss.protogen.tests.RD)
com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RD_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RD_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.RD.class, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder.class);
}
// Construct using com.alibaba.fluss.protogen.tests.Bytes.RD.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
records_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RD_descriptor;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RD getDefaultInstanceForType() {
return com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance();
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RD build() {
com.alibaba.fluss.protogen.tests.Bytes.RD result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RD buildPartial() {
com.alibaba.fluss.protogen.tests.Bytes.RD result = new com.alibaba.fluss.protogen.tests.Bytes.RD(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.alibaba.fluss.protogen.tests.Bytes.RD result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.records_ = records_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alibaba.fluss.protogen.tests.Bytes.RD) {
return mergeFrom((com.alibaba.fluss.protogen.tests.Bytes.RD)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alibaba.fluss.protogen.tests.Bytes.RD other) {
if (other == com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance()) return this;
if (other.hasRecords()) {
setRecords(other.getRecords());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
records_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString records_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes records = 1;
* @return Whether the records field is set.
*/
@java.lang.Override
public boolean hasRecords() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes records = 1;
* @return The records.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRecords() {
return records_;
}
/**
* optional bytes records = 1;
* @param value The records to set.
* @return This builder for chaining.
*/
public Builder setRecords(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
records_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional bytes records = 1;
* @return This builder for chaining.
*/
public Builder clearRecords() {
bitField0_ = (bitField0_ & ~0x00000001);
records_ = getDefaultInstance().getRecords();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.alibaba.fluss.protogen.tests.RD)
}
// @@protoc_insertion_point(class_scope:com.alibaba.fluss.protogen.tests.RD)
private static final com.alibaba.fluss.protogen.tests.Bytes.RD DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.alibaba.fluss.protogen.tests.Bytes.RD();
}
public static com.alibaba.fluss.protogen.tests.Bytes.RD getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RD parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RD getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ORDOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.alibaba.fluss.protogen.tests.ORD)
com.google.protobuf.MessageOrBuilder {
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
* @return Whether the rd field is set.
*/
boolean hasRd();
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
* @return The rd.
*/
com.alibaba.fluss.protogen.tests.Bytes.RD getRd();
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder getRdOrBuilder();
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.ORD}
*/
public static final class ORD extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.alibaba.fluss.protogen.tests.ORD)
ORDOrBuilder {
private static final long serialVersionUID = 0L;
// Use ORD.newBuilder() to construct.
private ORD(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ORD() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ORD();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_ORD_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_ORD_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.ORD.class, com.alibaba.fluss.protogen.tests.Bytes.ORD.Builder.class);
}
private int bitField0_;
public static final int RD_FIELD_NUMBER = 1;
private com.alibaba.fluss.protogen.tests.Bytes.RD rd_;
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
* @return Whether the rd field is set.
*/
@java.lang.Override
public boolean hasRd() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
* @return The rd.
*/
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RD getRd() {
return rd_ == null ? com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance() : rd_;
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder getRdOrBuilder() {
return rd_ == null ? com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance() : rd_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getRd());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRd());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.alibaba.fluss.protogen.tests.Bytes.ORD)) {
return super.equals(obj);
}
com.alibaba.fluss.protogen.tests.Bytes.ORD other = (com.alibaba.fluss.protogen.tests.Bytes.ORD) obj;
if (hasRd() != other.hasRd()) return false;
if (hasRd()) {
if (!getRd()
.equals(other.getRd())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRd()) {
hash = (37 * hash) + RD_FIELD_NUMBER;
hash = (53 * hash) + getRd().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.alibaba.fluss.protogen.tests.Bytes.ORD prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.ORD}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.alibaba.fluss.protogen.tests.ORD)
com.alibaba.fluss.protogen.tests.Bytes.ORDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_ORD_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_ORD_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.ORD.class, com.alibaba.fluss.protogen.tests.Bytes.ORD.Builder.class);
}
// Construct using com.alibaba.fluss.protogen.tests.Bytes.ORD.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRdFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
rd_ = null;
if (rdBuilder_ != null) {
rdBuilder_.dispose();
rdBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_ORD_descriptor;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.ORD getDefaultInstanceForType() {
return com.alibaba.fluss.protogen.tests.Bytes.ORD.getDefaultInstance();
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.ORD build() {
com.alibaba.fluss.protogen.tests.Bytes.ORD result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.ORD buildPartial() {
com.alibaba.fluss.protogen.tests.Bytes.ORD result = new com.alibaba.fluss.protogen.tests.Bytes.ORD(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.alibaba.fluss.protogen.tests.Bytes.ORD result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.rd_ = rdBuilder_ == null
? rd_
: rdBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alibaba.fluss.protogen.tests.Bytes.ORD) {
return mergeFrom((com.alibaba.fluss.protogen.tests.Bytes.ORD)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alibaba.fluss.protogen.tests.Bytes.ORD other) {
if (other == com.alibaba.fluss.protogen.tests.Bytes.ORD.getDefaultInstance()) return this;
if (other.hasRd()) {
mergeRd(other.getRd());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getRdFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.alibaba.fluss.protogen.tests.Bytes.RD rd_;
private com.google.protobuf.SingleFieldBuilderV3<
com.alibaba.fluss.protogen.tests.Bytes.RD, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder, com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder> rdBuilder_;
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
* @return Whether the rd field is set.
*/
public boolean hasRd() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
* @return The rd.
*/
public com.alibaba.fluss.protogen.tests.Bytes.RD getRd() {
if (rdBuilder_ == null) {
return rd_ == null ? com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance() : rd_;
} else {
return rdBuilder_.getMessage();
}
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder setRd(com.alibaba.fluss.protogen.tests.Bytes.RD value) {
if (rdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rd_ = value;
} else {
rdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder setRd(
com.alibaba.fluss.protogen.tests.Bytes.RD.Builder builderForValue) {
if (rdBuilder_ == null) {
rd_ = builderForValue.build();
} else {
rdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder mergeRd(com.alibaba.fluss.protogen.tests.Bytes.RD value) {
if (rdBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
rd_ != null &&
rd_ != com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance()) {
getRdBuilder().mergeFrom(value);
} else {
rd_ = value;
}
} else {
rdBuilder_.mergeFrom(value);
}
if (rd_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder clearRd() {
bitField0_ = (bitField0_ & ~0x00000001);
rd_ = null;
if (rdBuilder_ != null) {
rdBuilder_.dispose();
rdBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RD.Builder getRdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRdFieldBuilder().getBuilder();
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder getRdOrBuilder() {
if (rdBuilder_ != null) {
return rdBuilder_.getMessageOrBuilder();
} else {
return rd_ == null ?
com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance() : rd_;
}
}
/**
* optional .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.alibaba.fluss.protogen.tests.Bytes.RD, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder, com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>
getRdFieldBuilder() {
if (rdBuilder_ == null) {
rdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.alibaba.fluss.protogen.tests.Bytes.RD, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder, com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>(
getRd(),
getParentForChildren(),
isClean());
rd_ = null;
}
return rdBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.alibaba.fluss.protogen.tests.ORD)
}
// @@protoc_insertion_point(class_scope:com.alibaba.fluss.protogen.tests.ORD)
private static final com.alibaba.fluss.protogen.tests.Bytes.ORD DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.alibaba.fluss.protogen.tests.Bytes.ORD();
}
public static com.alibaba.fluss.protogen.tests.Bytes.ORD getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ORD parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.ORD getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RRDOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.alibaba.fluss.protogen.tests.RRD)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
java.util.List
getRdList();
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
com.alibaba.fluss.protogen.tests.Bytes.RD getRd(int index);
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
int getRdCount();
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
java.util.List extends com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>
getRdOrBuilderList();
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder getRdOrBuilder(
int index);
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.RRD}
*/
public static final class RRD extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.alibaba.fluss.protogen.tests.RRD)
RRDOrBuilder {
private static final long serialVersionUID = 0L;
// Use RRD.newBuilder() to construct.
private RRD(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RRD() {
rd_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RRD();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RRD_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RRD_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.RRD.class, com.alibaba.fluss.protogen.tests.Bytes.RRD.Builder.class);
}
public static final int RD_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List rd_;
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
@java.lang.Override
public java.util.List getRdList() {
return rd_;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
@java.lang.Override
public java.util.List extends com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>
getRdOrBuilderList() {
return rd_;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
@java.lang.Override
public int getRdCount() {
return rd_.size();
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RD getRd(int index) {
return rd_.get(index);
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder getRdOrBuilder(
int index) {
return rd_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < rd_.size(); i++) {
output.writeMessage(1, rd_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < rd_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, rd_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.alibaba.fluss.protogen.tests.Bytes.RRD)) {
return super.equals(obj);
}
com.alibaba.fluss.protogen.tests.Bytes.RRD other = (com.alibaba.fluss.protogen.tests.Bytes.RRD) obj;
if (!getRdList()
.equals(other.getRdList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getRdCount() > 0) {
hash = (37 * hash) + RD_FIELD_NUMBER;
hash = (53 * hash) + getRdList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.alibaba.fluss.protogen.tests.Bytes.RRD prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.alibaba.fluss.protogen.tests.RRD}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.alibaba.fluss.protogen.tests.RRD)
com.alibaba.fluss.protogen.tests.Bytes.RRDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RRD_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RRD_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.fluss.protogen.tests.Bytes.RRD.class, com.alibaba.fluss.protogen.tests.Bytes.RRD.Builder.class);
}
// Construct using com.alibaba.fluss.protogen.tests.Bytes.RRD.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (rdBuilder_ == null) {
rd_ = java.util.Collections.emptyList();
} else {
rd_ = null;
rdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.alibaba.fluss.protogen.tests.Bytes.internal_static_com_alibaba_fluss_protogen_tests_RRD_descriptor;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RRD getDefaultInstanceForType() {
return com.alibaba.fluss.protogen.tests.Bytes.RRD.getDefaultInstance();
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RRD build() {
com.alibaba.fluss.protogen.tests.Bytes.RRD result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RRD buildPartial() {
com.alibaba.fluss.protogen.tests.Bytes.RRD result = new com.alibaba.fluss.protogen.tests.Bytes.RRD(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.alibaba.fluss.protogen.tests.Bytes.RRD result) {
if (rdBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
rd_ = java.util.Collections.unmodifiableList(rd_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.rd_ = rd_;
} else {
result.rd_ = rdBuilder_.build();
}
}
private void buildPartial0(com.alibaba.fluss.protogen.tests.Bytes.RRD result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.alibaba.fluss.protogen.tests.Bytes.RRD) {
return mergeFrom((com.alibaba.fluss.protogen.tests.Bytes.RRD)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.alibaba.fluss.protogen.tests.Bytes.RRD other) {
if (other == com.alibaba.fluss.protogen.tests.Bytes.RRD.getDefaultInstance()) return this;
if (rdBuilder_ == null) {
if (!other.rd_.isEmpty()) {
if (rd_.isEmpty()) {
rd_ = other.rd_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRdIsMutable();
rd_.addAll(other.rd_);
}
onChanged();
}
} else {
if (!other.rd_.isEmpty()) {
if (rdBuilder_.isEmpty()) {
rdBuilder_.dispose();
rdBuilder_ = null;
rd_ = other.rd_;
bitField0_ = (bitField0_ & ~0x00000001);
rdBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRdFieldBuilder() : null;
} else {
rdBuilder_.addAllMessages(other.rd_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.alibaba.fluss.protogen.tests.Bytes.RD m =
input.readMessage(
com.alibaba.fluss.protogen.tests.Bytes.RD.PARSER,
extensionRegistry);
if (rdBuilder_ == null) {
ensureRdIsMutable();
rd_.add(m);
} else {
rdBuilder_.addMessage(m);
}
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List rd_ =
java.util.Collections.emptyList();
private void ensureRdIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
rd_ = new java.util.ArrayList(rd_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.alibaba.fluss.protogen.tests.Bytes.RD, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder, com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder> rdBuilder_;
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public java.util.List getRdList() {
if (rdBuilder_ == null) {
return java.util.Collections.unmodifiableList(rd_);
} else {
return rdBuilder_.getMessageList();
}
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public int getRdCount() {
if (rdBuilder_ == null) {
return rd_.size();
} else {
return rdBuilder_.getCount();
}
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RD getRd(int index) {
if (rdBuilder_ == null) {
return rd_.get(index);
} else {
return rdBuilder_.getMessage(index);
}
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder setRd(
int index, com.alibaba.fluss.protogen.tests.Bytes.RD value) {
if (rdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRdIsMutable();
rd_.set(index, value);
onChanged();
} else {
rdBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder setRd(
int index, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder builderForValue) {
if (rdBuilder_ == null) {
ensureRdIsMutable();
rd_.set(index, builderForValue.build());
onChanged();
} else {
rdBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder addRd(com.alibaba.fluss.protogen.tests.Bytes.RD value) {
if (rdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRdIsMutable();
rd_.add(value);
onChanged();
} else {
rdBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder addRd(
int index, com.alibaba.fluss.protogen.tests.Bytes.RD value) {
if (rdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRdIsMutable();
rd_.add(index, value);
onChanged();
} else {
rdBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder addRd(
com.alibaba.fluss.protogen.tests.Bytes.RD.Builder builderForValue) {
if (rdBuilder_ == null) {
ensureRdIsMutable();
rd_.add(builderForValue.build());
onChanged();
} else {
rdBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder addRd(
int index, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder builderForValue) {
if (rdBuilder_ == null) {
ensureRdIsMutable();
rd_.add(index, builderForValue.build());
onChanged();
} else {
rdBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder addAllRd(
java.lang.Iterable extends com.alibaba.fluss.protogen.tests.Bytes.RD> values) {
if (rdBuilder_ == null) {
ensureRdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rd_);
onChanged();
} else {
rdBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder clearRd() {
if (rdBuilder_ == null) {
rd_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
rdBuilder_.clear();
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public Builder removeRd(int index) {
if (rdBuilder_ == null) {
ensureRdIsMutable();
rd_.remove(index);
onChanged();
} else {
rdBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RD.Builder getRdBuilder(
int index) {
return getRdFieldBuilder().getBuilder(index);
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder getRdOrBuilder(
int index) {
if (rdBuilder_ == null) {
return rd_.get(index); } else {
return rdBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public java.util.List extends com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>
getRdOrBuilderList() {
if (rdBuilder_ != null) {
return rdBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rd_);
}
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RD.Builder addRdBuilder() {
return getRdFieldBuilder().addBuilder(
com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance());
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public com.alibaba.fluss.protogen.tests.Bytes.RD.Builder addRdBuilder(
int index) {
return getRdFieldBuilder().addBuilder(
index, com.alibaba.fluss.protogen.tests.Bytes.RD.getDefaultInstance());
}
/**
* repeated .com.alibaba.fluss.protogen.tests.RD rd = 1;
*/
public java.util.List
getRdBuilderList() {
return getRdFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.alibaba.fluss.protogen.tests.Bytes.RD, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder, com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>
getRdFieldBuilder() {
if (rdBuilder_ == null) {
rdBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.alibaba.fluss.protogen.tests.Bytes.RD, com.alibaba.fluss.protogen.tests.Bytes.RD.Builder, com.alibaba.fluss.protogen.tests.Bytes.RDOrBuilder>(
rd_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
rd_ = null;
}
return rdBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.alibaba.fluss.protogen.tests.RRD)
}
// @@protoc_insertion_point(class_scope:com.alibaba.fluss.protogen.tests.RRD)
private static final com.alibaba.fluss.protogen.tests.Bytes.RRD DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.alibaba.fluss.protogen.tests.Bytes.RRD();
}
public static com.alibaba.fluss.protogen.tests.Bytes.RRD getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RRD parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.alibaba.fluss.protogen.tests.Bytes.RRD getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_alibaba_fluss_protogen_tests_B_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_alibaba_fluss_protogen_tests_B_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_alibaba_fluss_protogen_tests_RD_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_alibaba_fluss_protogen_tests_RD_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_alibaba_fluss_protogen_tests_ORD_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_alibaba_fluss_protogen_tests_ORD_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_alibaba_fluss_protogen_tests_RRD_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_alibaba_fluss_protogen_tests_RRD_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\013bytes.proto\022 com.alibaba.fluss.protoge" +
"n.tests\")\n\001B\022\017\n\007payload\030\001 \001(\014\022\023\n\013extra_i" +
"tems\030\002 \003(\014\"\025\n\002RD\022\017\n\007records\030\001 \001(\014\"7\n\003ORD" +
"\0220\n\002rd\030\001 \001(\0132$.com.alibaba.fluss.protoge" +
"n.tests.RD\"7\n\003RRD\0220\n\002rd\030\001 \003(\0132$.com.alib" +
"aba.fluss.protogen.tests.RD"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_com_alibaba_fluss_protogen_tests_B_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_alibaba_fluss_protogen_tests_B_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_alibaba_fluss_protogen_tests_B_descriptor,
new java.lang.String[] { "Payload", "ExtraItems", });
internal_static_com_alibaba_fluss_protogen_tests_RD_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_com_alibaba_fluss_protogen_tests_RD_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_alibaba_fluss_protogen_tests_RD_descriptor,
new java.lang.String[] { "Records", });
internal_static_com_alibaba_fluss_protogen_tests_ORD_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_com_alibaba_fluss_protogen_tests_ORD_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_alibaba_fluss_protogen_tests_ORD_descriptor,
new java.lang.String[] { "Rd", });
internal_static_com_alibaba_fluss_protogen_tests_RRD_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_com_alibaba_fluss_protogen_tests_RRD_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_alibaba_fluss_protogen_tests_RRD_descriptor,
new java.lang.String[] { "Rd", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy