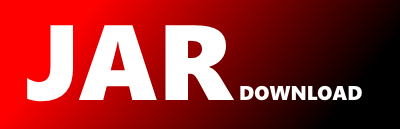
com.alibaba.graphscope.groot.sdk.schema.Edge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of groot-client Show documentation
Show all versions of groot-client Show documentation
The Java client of Groot, a persistence storage engine
package com.alibaba.graphscope.groot.sdk.schema;
import com.alibaba.graphscope.proto.groot.*;
import java.util.Map;
public class Edge {
public String label;
public String srcLabel;
public String dstLabel;
public Map srcPk;
public Map dstPk;
public Map properties;
public long eid;
/**
* Construct an edge
* @param label edge label
* @param srcLabel source vertex label
* @param dstLabel destination vertex label
* @param srcPk source primary keys
* @param dstPk destination primary keys
* @param properties edge properties
*/
public Edge(
String label,
String srcLabel,
String dstLabel,
Map srcPk,
Map dstPk,
Map properties) {
this.label = label;
this.srcLabel = srcLabel;
this.dstLabel = dstLabel;
this.srcPk = srcPk;
this.dstPk = dstPk;
this.properties = properties;
}
public Edge(
String label,
String srcLabel,
String dstLabel,
Map srcPk,
Map dstPk) {
this(label, srcLabel, dstLabel, srcPk, dstPk, null);
}
public Edge(String label, Vertex src, Vertex dst, Map properties) {
this(
label,
src.getLabel(),
dst.getLabel(),
src.getProperties(),
dst.getProperties(),
properties);
}
public Edge(String label, Vertex src, Vertex dst) {
this(label, src, dst, null);
}
public void setEid(long eid) {
this.eid = eid;
}
public String getLabel() {
return label;
}
public String getSrcLabel() {
return srcLabel;
}
public String getDstLabel() {
return dstLabel;
}
public Map getSrcPk() {
return srcPk;
}
public Map getDstPk() {
return dstPk;
}
public Map getProperties() {
return properties;
}
public EdgeRecordKeyPb toEdgeRecordKey() {
return EdgeRecordKeyPb.newBuilder()
.setLabel(label)
.setSrcVertexKey(toVertexRecordKey(srcLabel, srcPk))
.setDstVertexKey(toVertexRecordKey(dstLabel, dstPk))
.setInnerId(eid)
.build();
}
public DataRecordPb toDataRecord() {
DataRecordPb.Builder builder =
DataRecordPb.newBuilder().setEdgeRecordKey(toEdgeRecordKey());
if (properties != null) {
builder.putAllProperties(properties);
}
return builder.build();
}
public WriteRequestPb toWriteRequest(WriteTypePb writeType) {
return WriteRequestPb.newBuilder()
.setWriteType(writeType)
.setDataRecord(toDataRecord())
.build();
}
private VertexRecordKeyPb toVertexRecordKey(String label, Map properties) {
VertexRecordKeyPb.Builder builder = VertexRecordKeyPb.newBuilder().setLabel(label);
builder.putAllPkProperties(properties);
return builder.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy