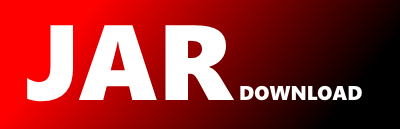
com.alibaba.graphscope.proto.groot.ClientWriteGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of groot-client Show documentation
Show all versions of groot-client Show documentation
The Java client of Groot, a persistence storage engine
package com.alibaba.graphscope.proto.groot;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.51.1)",
comments = "Source: write_service.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class ClientWriteGrpc {
private ClientWriteGrpc() {}
public static final String SERVICE_NAME = "gs.rpc.groot.ClientWrite";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getGetClientIdMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "getClientId",
requestType = com.alibaba.graphscope.proto.groot.GetClientIdRequest.class,
responseType = com.alibaba.graphscope.proto.groot.GetClientIdResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetClientIdMethod() {
io.grpc.MethodDescriptor getGetClientIdMethod;
if ((getGetClientIdMethod = ClientWriteGrpc.getGetClientIdMethod) == null) {
synchronized (ClientWriteGrpc.class) {
if ((getGetClientIdMethod = ClientWriteGrpc.getGetClientIdMethod) == null) {
ClientWriteGrpc.getGetClientIdMethod = getGetClientIdMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "getClientId"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.alibaba.graphscope.proto.groot.GetClientIdRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.alibaba.graphscope.proto.groot.GetClientIdResponse.getDefaultInstance()))
.setSchemaDescriptor(new ClientWriteMethodDescriptorSupplier("getClientId"))
.build();
}
}
}
return getGetClientIdMethod;
}
private static volatile io.grpc.MethodDescriptor getBatchWriteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "batchWrite",
requestType = com.alibaba.graphscope.proto.groot.BatchWriteRequest.class,
responseType = com.alibaba.graphscope.proto.groot.BatchWriteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getBatchWriteMethod() {
io.grpc.MethodDescriptor getBatchWriteMethod;
if ((getBatchWriteMethod = ClientWriteGrpc.getBatchWriteMethod) == null) {
synchronized (ClientWriteGrpc.class) {
if ((getBatchWriteMethod = ClientWriteGrpc.getBatchWriteMethod) == null) {
ClientWriteGrpc.getBatchWriteMethod = getBatchWriteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "batchWrite"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.alibaba.graphscope.proto.groot.BatchWriteRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.alibaba.graphscope.proto.groot.BatchWriteResponse.getDefaultInstance()))
.setSchemaDescriptor(new ClientWriteMethodDescriptorSupplier("batchWrite"))
.build();
}
}
}
return getBatchWriteMethod;
}
private static volatile io.grpc.MethodDescriptor getRemoteFlushMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "remoteFlush",
requestType = com.alibaba.graphscope.proto.groot.RemoteFlushRequest.class,
responseType = com.alibaba.graphscope.proto.groot.RemoteFlushResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRemoteFlushMethod() {
io.grpc.MethodDescriptor getRemoteFlushMethod;
if ((getRemoteFlushMethod = ClientWriteGrpc.getRemoteFlushMethod) == null) {
synchronized (ClientWriteGrpc.class) {
if ((getRemoteFlushMethod = ClientWriteGrpc.getRemoteFlushMethod) == null) {
ClientWriteGrpc.getRemoteFlushMethod = getRemoteFlushMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "remoteFlush"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.alibaba.graphscope.proto.groot.RemoteFlushRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.alibaba.graphscope.proto.groot.RemoteFlushResponse.getDefaultInstance()))
.setSchemaDescriptor(new ClientWriteMethodDescriptorSupplier("remoteFlush"))
.build();
}
}
}
return getRemoteFlushMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static ClientWriteStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ClientWriteStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ClientWriteStub(channel, callOptions);
}
};
return ClientWriteStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static ClientWriteBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ClientWriteBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ClientWriteBlockingStub(channel, callOptions);
}
};
return ClientWriteBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static ClientWriteFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ClientWriteFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ClientWriteFutureStub(channel, callOptions);
}
};
return ClientWriteFutureStub.newStub(factory, channel);
}
/**
*/
public static abstract class ClientWriteImplBase implements io.grpc.BindableService {
/**
*/
public void getClientId(com.alibaba.graphscope.proto.groot.GetClientIdRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetClientIdMethod(), responseObserver);
}
/**
*/
public void batchWrite(com.alibaba.graphscope.proto.groot.BatchWriteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBatchWriteMethod(), responseObserver);
}
/**
*/
public void remoteFlush(com.alibaba.graphscope.proto.groot.RemoteFlushRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRemoteFlushMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getGetClientIdMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.alibaba.graphscope.proto.groot.GetClientIdRequest,
com.alibaba.graphscope.proto.groot.GetClientIdResponse>(
this, METHODID_GET_CLIENT_ID)))
.addMethod(
getBatchWriteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.alibaba.graphscope.proto.groot.BatchWriteRequest,
com.alibaba.graphscope.proto.groot.BatchWriteResponse>(
this, METHODID_BATCH_WRITE)))
.addMethod(
getRemoteFlushMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.alibaba.graphscope.proto.groot.RemoteFlushRequest,
com.alibaba.graphscope.proto.groot.RemoteFlushResponse>(
this, METHODID_REMOTE_FLUSH)))
.build();
}
}
/**
*/
public static final class ClientWriteStub extends io.grpc.stub.AbstractAsyncStub {
private ClientWriteStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ClientWriteStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ClientWriteStub(channel, callOptions);
}
/**
*/
public void getClientId(com.alibaba.graphscope.proto.groot.GetClientIdRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetClientIdMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void batchWrite(com.alibaba.graphscope.proto.groot.BatchWriteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getBatchWriteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void remoteFlush(com.alibaba.graphscope.proto.groot.RemoteFlushRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRemoteFlushMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*/
public static final class ClientWriteBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private ClientWriteBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ClientWriteBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ClientWriteBlockingStub(channel, callOptions);
}
/**
*/
public com.alibaba.graphscope.proto.groot.GetClientIdResponse getClientId(com.alibaba.graphscope.proto.groot.GetClientIdRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetClientIdMethod(), getCallOptions(), request);
}
/**
*/
public com.alibaba.graphscope.proto.groot.BatchWriteResponse batchWrite(com.alibaba.graphscope.proto.groot.BatchWriteRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getBatchWriteMethod(), getCallOptions(), request);
}
/**
*/
public com.alibaba.graphscope.proto.groot.RemoteFlushResponse remoteFlush(com.alibaba.graphscope.proto.groot.RemoteFlushRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRemoteFlushMethod(), getCallOptions(), request);
}
}
/**
*/
public static final class ClientWriteFutureStub extends io.grpc.stub.AbstractFutureStub {
private ClientWriteFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ClientWriteFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ClientWriteFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getClientId(
com.alibaba.graphscope.proto.groot.GetClientIdRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetClientIdMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture batchWrite(
com.alibaba.graphscope.proto.groot.BatchWriteRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getBatchWriteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture remoteFlush(
com.alibaba.graphscope.proto.groot.RemoteFlushRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRemoteFlushMethod(), getCallOptions()), request);
}
}
private static final int METHODID_GET_CLIENT_ID = 0;
private static final int METHODID_BATCH_WRITE = 1;
private static final int METHODID_REMOTE_FLUSH = 2;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final ClientWriteImplBase serviceImpl;
private final int methodId;
MethodHandlers(ClientWriteImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_GET_CLIENT_ID:
serviceImpl.getClientId((com.alibaba.graphscope.proto.groot.GetClientIdRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BATCH_WRITE:
serviceImpl.batchWrite((com.alibaba.graphscope.proto.groot.BatchWriteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REMOTE_FLUSH:
serviceImpl.remoteFlush((com.alibaba.graphscope.proto.groot.RemoteFlushRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class ClientWriteBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
ClientWriteBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.alibaba.graphscope.proto.groot.WriteService.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("ClientWrite");
}
}
private static final class ClientWriteFileDescriptorSupplier
extends ClientWriteBaseDescriptorSupplier {
ClientWriteFileDescriptorSupplier() {}
}
private static final class ClientWriteMethodDescriptorSupplier
extends ClientWriteBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
ClientWriteMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (ClientWriteGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new ClientWriteFileDescriptorSupplier())
.addMethod(getGetClientIdMethod())
.addMethod(getBatchWriteMethod())
.addMethod(getRemoteFlushMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy