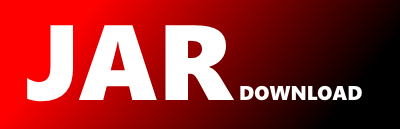
com.taobao.hsf.junit.HSFRunner Maven / Gradle / Ivy
The newest version!
package com.taobao.hsf.junit;
import com.taobao.hsf.standalone.HSFStarter;
import org.junit.runner.Description;
import org.junit.runner.Runner;
import org.junit.runner.notification.RunNotifier;
import org.junit.runners.JUnit4;
import org.junit.runners.model.InitializationError;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.util.Map;
/**
* @author bw on 10/26/15.
*/
public class HSFRunner extends Runner {
private Runner runner;
private Behavior behavior;
@SuppressWarnings("unchecked")
public HSFRunner(Class> testClass) throws InitializationError {
try {
this.behavior = prepareClassLoader(testClass);
this.runner = prepareDelegateRunner(testClass, behavior);
} catch (NoSuchMethodException e) {
throw new InitializationError(e);
} catch (InstantiationException e) {
throw new InitializationError(e);
} catch (IllegalAccessException e) {
throw new InitializationError(e);
} catch (InvocationTargetException e) {
throw new InitializationError(e);
} catch (ClassNotFoundException e) {
throw new InitializationError(e);
} catch (Exception e) {
throw new InitializationError(e);
}
}
@Override
public Description getDescription() {
return runner.getDescription();
}
@Override
public void run(RunNotifier notifier) {
try {
behavior.before();
runner.run(notifier);
} finally {
behavior.after();
}
}
@SuppressWarnings("unchecked")
private Runner prepareDelegateRunner(Class> clazz, Behavior classLoader) throws Exception {
Class runnerClass = classLoader.loadClass(JUnit4.class.getName());
DelegateTo delegateTo = clazz.getAnnotation(DelegateTo.class);
if (delegateTo != null) {
runnerClass = classLoader.loadClass(delegateTo.value().getName());
}
Class testClassToInvoke = classLoader.loadClass(clazz.getName());
Constructor constructor = runnerClass.getConstructor(Class.class);
constructor.setAccessible(true);
return (Runner) constructor.newInstance(testClassToInvoke);
}
@SuppressWarnings("unchecked")
private Behavior prepareClassLoader(Class> clazz) throws Exception {
Map> cache = findClassCache();
boolean share = true;
Sar sarInfo = clazz.getAnnotation(Sar.class);
if (sarInfo != null) {
share = sarInfo.share();
}
// FIXME: share = false only works between HSFRunner, but will break when HSFRunner and LightAPI are mixed.
return (cache == null) ? new DefaultBehavior(clazz) : (share) ? new ExistingCacheBehavior(cache, clazz) :
new DefaultBehavior(clazz);
}
@SuppressWarnings("unchecked")
static Map> findClassCache() throws NoSuchFieldException, IllegalAccessException {
Field exportedClassMapField = HSFStarter.class.getDeclaredField("exportedClassMap");
exportedClassMapField.setAccessible(true);
return (Map>) exportedClassMapField.get(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy