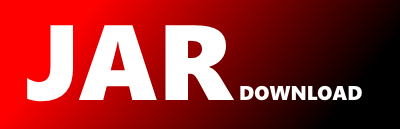
com.taobao.hsf.lightapi.util.ReflectionUtil Maven / Gradle / Ivy
The newest version!
package com.taobao.hsf.lightapi.util;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
/**
* Created by huangsheng.hs on 2014/11/30.
*/
public class ReflectionUtil {
/**
* @param instance
* @param methodName
* @return
* @throws Exception
*/
public static Object invoke(Object instance, String methodName)
throws Exception {
Method method = instance.getClass().getMethod(methodName);
method.setAccessible(true);
return method.invoke(instance, null);
}
/**
* @param instance
* @param methodName
* @param params
* @return
* @throws Exception
*/
public static Object invoke(Object instance, String methodName, Object... params)
throws Exception {
Class[] paramTypes = new Class[params.length];
for (int i = 0; i < params.length; i++) {
paramTypes[i] = params[i].getClass();
}
Method method = instance.getClass().getDeclaredMethod(methodName, paramTypes);
method.setAccessible(true);
Class returnType = method.getReturnType();
if (returnType == null) {// returnType instanceof Void
method.invoke(instance, params);
}
return method.invoke(instance, params);
}
/**
* @param clazz
* @param methodName
* @param params
* @return
* @throws Exception
*/
public static Object staticInvoke(Class clazz, String methodName, Object... params)
throws Exception {
Class[] paramTypes = new Class[params.length];
for (int i = 0; i < params.length; i++) {
paramTypes[i] = params[i].getClass();
}
Method method = clazz.getDeclaredMethod(methodName, paramTypes);
method.setAccessible(true);
return method.invoke(null, params);
}
/**
* @param clazz
* @param methodName
* @return
* @throws Exception
*/
public static Object staticInvoke(Class clazz, String methodName)
throws Exception {
Method method = clazz.getDeclaredMethod(methodName);
method.setAccessible(true);
return method.invoke(null, null);
}
/**
* @param instance
* @param fieldName
* @return
* @throws Exception
*/
public static Object getField(Object instance, String fieldName) throws Exception {
Class clazz = instance.getClass();
Field field = clazz.getDeclaredField(fieldName);
field.setAccessible(true);
return field.get(instance);
}
/**
* @param clazz
* @param fieldName
* @return
* @throws Exception
*/
public static Object getStaticField(Class clazz, String fieldName) throws Exception {
Field field = clazz.getDeclaredField(fieldName);
field.setAccessible(true);
return field.get(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy