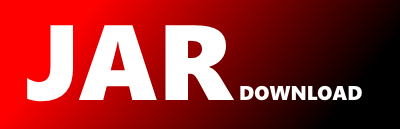
com.alibaba.nacos.spring.context.annotation.discovery.EnableNacosDiscovery Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.nacos.spring.context.annotation.discovery;
import static com.alibaba.nacos.api.PropertyKeyConst.PASSWORD;
import static com.alibaba.nacos.api.PropertyKeyConst.USERNAME;
import static com.alibaba.nacos.api.annotation.NacosProperties.ACCESS_KEY;
import static com.alibaba.nacos.api.annotation.NacosProperties.CLUSTER_NAME;
import static com.alibaba.nacos.api.annotation.NacosProperties.CONTEXT_PATH;
import static com.alibaba.nacos.api.annotation.NacosProperties.ENCODE;
import static com.alibaba.nacos.api.annotation.NacosProperties.ENDPOINT;
import static com.alibaba.nacos.api.annotation.NacosProperties.NAMESPACE;
import static com.alibaba.nacos.api.annotation.NacosProperties.SECRET_KEY;
import static com.alibaba.nacos.api.annotation.NacosProperties.SERVER_ADDR;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import org.springframework.context.annotation.Import;
import com.alibaba.nacos.api.annotation.NacosInjected;
import com.alibaba.nacos.api.annotation.NacosProperties;
import com.alibaba.nacos.spring.context.annotation.NacosBeanDefinitionRegistrar;
/**
* Annotation for enabling Nacos discovery features.
*
* @author Mercy
* @see NacosBeanDefinitionRegistrar
* @since 0.1.0
*/
@Target({ ElementType.TYPE, ElementType.ANNOTATION_TYPE })
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Import({NacosDiscoveryBeanDefinitionRegistrar.class, EnableNacosDiscoveryAotProcessor.class})
public @interface EnableNacosDiscovery {
/**
* The prefix of property name of Nacos discovery
*/
String DISCOVERY_PREFIX = NacosProperties.PREFIX + "discovery.";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.endpoint:${nacos.endpoint:}}"
*/
String ENDPOINT_PLACEHOLDER = "${" + DISCOVERY_PREFIX + ENDPOINT + ":"
+ NacosProperties.ENDPOINT_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.namespace:${nacos.namespace:}}"
*/
String NAMESPACE_PLACEHOLDER = "${" + DISCOVERY_PREFIX + NAMESPACE + ":"
+ NacosProperties.NAMESPACE_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.access-key:${nacos.access-key:}}"
*/
String ACCESS_KEY_PLACEHOLDER = "${" + DISCOVERY_PREFIX + ACCESS_KEY + ":"
+ NacosProperties.ACCESS_KEY_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.secret-key:${nacos.secret-key:}}"
*/
String SECRET_KEY_PLACEHOLDER = "${" + DISCOVERY_PREFIX + SECRET_KEY + ":"
+ NacosProperties.SECRET_KEY_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.server-addr:${nacos.server-addr:}}"
*/
String SERVER_ADDR_PLACEHOLDER = "${" + DISCOVERY_PREFIX + SERVER_ADDR + ":"
+ NacosProperties.SERVER_ADDR_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.context-path:${nacos.context-path:}}"
*/
String CONTEXT_PATH_PLACEHOLDER = "${" + DISCOVERY_PREFIX + CONTEXT_PATH + ":"
+ NacosProperties.CONTEXT_PATH_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.cluster-name:${nacos.cluster-name:}}"
*/
String CLUSTER_NAME_PLACEHOLDER = "${" + DISCOVERY_PREFIX + CLUSTER_NAME + ":"
+ NacosProperties.CLUSTER_NAME_PLACEHOLDER + "}";
/**
* The placeholder of {@link NacosProperties#ENCODE encode}, the value is
* "${nacos.discovery.encode:${nacos.encode:UTF-8}}"
*/
String ENCODE_PLACEHOLDER = "${" + DISCOVERY_PREFIX + ENCODE + ":"
+ NacosProperties.ENCODE_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.username:${nacos.username:}}"
*/
String USERNAME_PLACEHOLDER = "${" + DISCOVERY_PREFIX + USERNAME + ":"
+ NacosProperties.USERNAME_PLACEHOLDER + "}";
/**
* The placeholder of endpoint, the value is
* "${nacos.discovery.password:${nacos.password:}}"
*/
String PASSWORD_PLACEHOLDER = "${" + DISCOVERY_PREFIX + PASSWORD + ":"
+ NacosProperties.PASSWORD_PLACEHOLDER + "}";
/**
* Global {@link NacosProperties Nacos Properties}
*
* @return required
* @see NacosInjected#properties()
*/
NacosProperties globalProperties() default @NacosProperties(username = USERNAME_PLACEHOLDER, password = PASSWORD_PLACEHOLDER, endpoint = ENDPOINT_PLACEHOLDER, namespace = NAMESPACE_PLACEHOLDER, accessKey = ACCESS_KEY_PLACEHOLDER, secretKey = SECRET_KEY_PLACEHOLDER, serverAddr = SERVER_ADDR_PLACEHOLDER, contextPath = CONTEXT_PATH_PLACEHOLDER, clusterName = CLUSTER_NAME_PLACEHOLDER, encode = ENCODE_PLACEHOLDER);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy