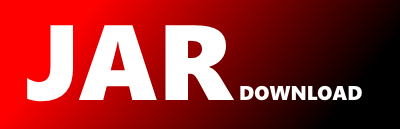
com.alibaba.rsocket.util.ByteBufTuples Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibaba-rsocket-service-common Show documentation
Show all versions of alibaba-rsocket-service-common Show documentation
RSocket service common to help Reactive Service API definition
package com.alibaba.rsocket.util;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import reactor.util.function.*;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
/**
* ByteBuf Tuples
*
* @author leijuan
*/
@SuppressWarnings("unchecked")
public class ByteBufTuples {
private static final ByteBuffer EMPTY_BYTE_BUFFER = ByteBuffer.allocate(0);
private static final Map, ByteBufValueReader>> READERS = new HashMap<>();
static {
READERS.put(Byte.class, ByteBuf::readByte);
READERS.put(Integer.class, ByteBuf::readInt);
READERS.put(Long.class, ByteBuf::readLong);
READERS.put(Double.class, ByteBuf::readDouble);
READERS.put(Boolean.class, buf -> buf.readByte() == 1);
READERS.put(byte[].class, buf -> {
int len = buf.readInt();
if (len == 0) {
return new byte[0];
} else {
byte[] bytes = new byte[len];
buf.readBytes(bytes);
return bytes;
}
});
READERS.put(ByteBuf.class, buf -> {
int len = buf.readInt();
if (len == 0) {
return Unpooled.EMPTY_BUFFER;
} else {
return buf.readSlice(len);
}
});
READERS.put(ByteBuffer.class, buf -> {
int len = buf.readInt();
if (len == 0) {
return EMPTY_BYTE_BUFFER;
} else {
return buf.readSlice(len).nioBuffer();
}
});
READERS.put(String.class, buf -> {
int len = buf.readInt();
if (len == 0) {
return "";
} else {
return buf.readCharSequence(len, StandardCharsets.UTF_8).toString();
}
});
}
public static Tuple2 of(ByteBuf buf, Class t1, Class t2) {
return (Tuple2) Tuples.of(read(buf, t1), read(buf, t2));
}
public static Tuple3 of(ByteBuf buf, Class t1, Class t2, Class t3) {
return (Tuple3) Tuples.of(read(buf, t1), read(buf, t2), read(buf, t3));
}
public static Tuple4 of(ByteBuf buf, Class t1, Class t2, Class t3, Class t4) {
return (Tuple4) Tuples.of(read(buf, t1), read(buf, t2), read(buf, t3), read(buf, t4));
}
public static Tuple5 of(
ByteBuf buf,
Class t1,
Class t2,
Class t3,
Class t4,
Class t5) {
return (Tuple5) Tuples.of(read(buf, t1), read(buf, t2), read(buf, t3), read(buf, t4), read(buf, t5));
}
public static Tuple6 of(
ByteBuf buf,
Class t1,
Class t2,
Class t3,
Class t4,
Class t5,
Class t6) {
return (Tuple6) Tuples.of(read(buf, t1), read(buf, t2), read(buf, t3), read(buf, t4), read(buf, t5), read(buf, t6));
}
public static > Object read(ByteBuf buf, T type) {
return READERS.get(type).read(buf);
}
@FunctionalInterface
public interface ByteBufValueReader {
T read(ByteBuf buf);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy