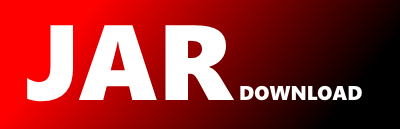
com.alibaba.qlexpress4.runtime.QLambda Maven / Gradle / Ivy
package com.alibaba.qlexpress4.runtime;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
public interface QLambda
extends Runnable, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy