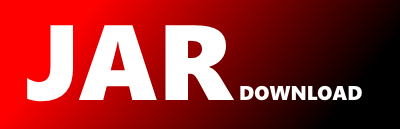
com.alicp.jetcache.AbstractCacheBuilder Maven / Gradle / Ivy
The newest version!
package com.alicp.jetcache;
import java.util.List;
import java.util.concurrent.TimeUnit;
import java.util.function.Function;
/**
* Created on 16/9/7.
*
* @author huangli
*/
public abstract class AbstractCacheBuilder> implements CacheBuilder, Cloneable {
protected CacheConfig config;
private Function buildFunc;
public abstract CacheConfig getConfig();
protected T self() {
return (T) this;
}
public T buildFunc(Function buildFunc) {
this.buildFunc = buildFunc;
return self();
}
protected void beforeBuild() {
}
@Deprecated
public final Cache build() {
return buildCache();
}
@Override
public final Cache buildCache() {
if (buildFunc == null) {
throw new CacheConfigException("no buildFunc");
}
beforeBuild();
CacheConfig c = getConfig().clone();
Cache cache = buildFunc.apply(c);
if (c.getLoader() != null) {
if (c.getRefreshPolicy() == null) {
cache = new LoadingCache<>(cache);
} else {
cache = new RefreshCache<>(cache);
}
}
return cache;
}
@Override
public Object clone() {
AbstractCacheBuilder copy = null;
try {
copy = (AbstractCacheBuilder) super.clone();
copy.config = getConfig().clone();
return copy;
} catch (CloneNotSupportedException e) {
throw new CacheException(e);
}
}
public T keyConvertor(Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy