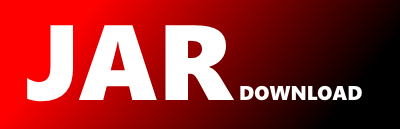
com.alicp.jetcache.LoadingCache Maven / Gradle / Ivy
The newest version!
package com.alicp.jetcache;
import com.alicp.jetcache.event.CacheEvent;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
import java.util.stream.Collectors;
/**
* Created on 2017/5/17.
*
* @author huangli
*/
public class LoadingCache extends SimpleProxyCache {
protected Consumer eventConsumer;
protected CacheConfig config;
public LoadingCache(Cache cache) {
super(cache);
this.config = config();
eventConsumer = CacheUtil.getAbstractCache(cache)::notify;
}
@Override
public V get(K key) throws CacheInvokeException {
CacheLoader loader = config.getLoader();
if (loader != null) {
return AbstractCache.computeIfAbsentImpl(key, loader,
config.isCacheNullValue() ,0, null, this);
} else {
return cache.get(key);
}
}
protected boolean needUpdate(V loadedValue, CacheLoader loader) {
if (loadedValue == null && !config.isCacheNullValue()) {
return false;
}
if (loader.vetoCacheUpdate()) {
return false;
}
return true;
}
@Override
public Map getAll(Set extends K> keys) throws CacheInvokeException {
CacheLoader loader = config.getLoader();
if (loader != null) {
MultiGetResult r = GET_ALL(keys);
Map kvMap;
if (r.isSuccess() || r.getResultCode() == CacheResultCode.PART_SUCCESS) {
kvMap = r.unwrapValues();
} else {
kvMap = new HashMap<>();
}
Set keysNeedLoad = new LinkedHashSet<>();
keys.forEach((k) -> {
if (!kvMap.containsKey(k)) {
keysNeedLoad.add(k);
}
});
if (!config.isCachePenetrationProtect()) {
if (eventConsumer != null) {
loader = CacheUtil.createProxyLoader(cache, loader, eventConsumer);
}
Map loadResult;
try {
loadResult = loader.loadAll(keysNeedLoad);
CacheLoader theLoader = loader;
Map updateValues = new HashMap<>();
loadResult.forEach((k,v)->{
if (needUpdate(v, theLoader)){
updateValues.put(k, v);
}
});
// batch put
if (!updateValues.isEmpty()) {
PUT_ALL(updateValues);
}
} catch (Throwable e) {
throw new CacheInvokeException(e);
}
kvMap.putAll(loadResult);
} else {
AbstractCache abstractCache = CacheUtil.getAbstractCache(cache);
loader = CacheUtil.createProxyLoader(cache, loader, eventConsumer);
for(K key : keysNeedLoad) {
Consumer cacheUpdater = (v) -> {
if(needUpdate(v, config.getLoader())) {
PUT(key, v);
}
};
V v = AbstractCache.synchronizedLoad(config, abstractCache, key, loader, cacheUpdater);
kvMap.put(key, v);
}
}
return kvMap;
} else {
return cache.getAll(keys);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy