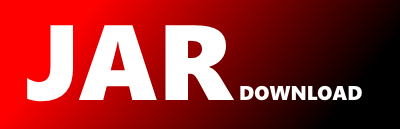
com.alicp.jetcache.MultiLevelCacheBuilder Maven / Gradle / Ivy
The newest version!
package com.alicp.jetcache;
import java.util.List;
import java.util.concurrent.TimeUnit;
import java.util.function.Function;
/**
* Created on 2017/5/24.
*
* @author huangli
*/
public class MultiLevelCacheBuilder> extends AbstractCacheBuilder {
public static class MultiLevelCacheBuilderImpl extends MultiLevelCacheBuilder {
}
public static MultiLevelCacheBuilderImpl createMultiLevelCacheBuilder() {
return new MultiLevelCacheBuilderImpl();
}
protected MultiLevelCacheBuilder() {
buildFunc(config -> new MultiLevelCache((MultiLevelCacheConfig) config));
}
@Override
public MultiLevelCacheConfig getConfig() {
if (config == null) {
config = new MultiLevelCacheConfig();
}
return (MultiLevelCacheConfig) config;
}
public T addCache(Cache... caches) {
for (Cache c : caches) {
getConfig().getCaches().add(c);
}
return self();
}
public void setCaches(List caches) {
getConfig().setCaches(caches);
}
public T useExpireOfSubCache(boolean useExpireOfSubCache) {
getConfig().setUseExpireOfSubCache(useExpireOfSubCache);
return self();
}
public void setUseExpireOfSubCache(boolean useExpireOfSubCache) {
getConfig().setUseExpireOfSubCache(useExpireOfSubCache);
}
@Override
public T keyConvertor(Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy