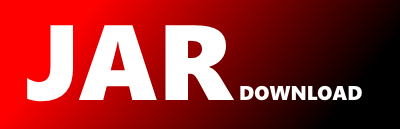
com.alicp.jetcache.embedded.AbstractEmbeddedCache Maven / Gradle / Ivy
The newest version!
/**
* Created on 13-10-17 23:01
*/
package com.alicp.jetcache.embedded;
import com.alicp.jetcache.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.ReentrantLock;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* @author huangli
*/
public abstract class AbstractEmbeddedCache extends AbstractCache {
protected EmbeddedCacheConfig config;
protected InnerMap innerMap;
protected abstract InnerMap createAreaCache();
private final ReentrantLock lock = new ReentrantLock();
public AbstractEmbeddedCache(EmbeddedCacheConfig config) {
this.config = config;
innerMap = createAreaCache();
}
@Override
public CacheConfig config() {
return config;
}
public Object buildKey(K key) {
Object newKey = key;
Function keyConvertor = config.getKeyConvertor();
if (keyConvertor != null) {
newKey = keyConvertor.apply(key);
}
return newKey;
}
@Override
protected CacheGetResult do_GET(K key) {
Object newKey = buildKey(key);
CacheValueHolder holder = (CacheValueHolder) innerMap.getValue(newKey);
return parseHolderResult(holder);
}
protected CacheGetResult parseHolderResult(CacheValueHolder holder) {
long now = System.currentTimeMillis();
if (holder == null) {
return CacheGetResult.NOT_EXISTS_WITHOUT_MSG;
} else if (now >= holder.getExpireTime()) {
return CacheGetResult.EXPIRED_WITHOUT_MSG;
} else {
lock.lock();
try{
long accessTime = holder.getAccessTime();
if (config.isExpireAfterAccess()) {
long expireAfterAccess = config.getExpireAfterAccessInMillis();
if (now >= accessTime + expireAfterAccess) {
return CacheGetResult.EXPIRED_WITHOUT_MSG;
}
}
holder.setAccessTime(now);
}finally {
lock.unlock();
}
return new CacheGetResult(CacheResultCode.SUCCESS, null, holder);
}
}
@Override
protected MultiGetResult do_GET_ALL(Set extends K> keys) {
ArrayList keyList = new ArrayList(keys.size());
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy