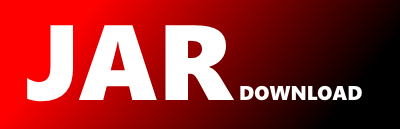
com.alilitech.swagger.web.entity.Path Maven / Gradle / Ivy
/**
* Copyright 2017-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alilitech.swagger.web.entity;
import java.util.List;
import java.util.Map;
/**
*
* @author Zhou Xiaoxiang
* @since 1.0
*/
public class Path {
private List tags;
private String summary;
private String operationId;
private List produces;
private List consumes;
private List parameters;
private Map responses;
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public String getOperationId() {
return operationId;
}
public void setOperationId(String operationId) {
this.operationId = operationId;
}
public List getProduces() {
return produces;
}
public void setProduces(List produces) {
this.produces = produces;
}
public List getConsumes() {
return consumes;
}
public void setConsumes(List consumes) {
this.consumes = consumes;
}
public List getParameters() {
return parameters;
}
public void setParameters(List parameters) {
this.parameters = parameters;
}
public Map getResponses() {
return responses;
}
public void setResponses(Map responses) {
this.responses = responses;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy