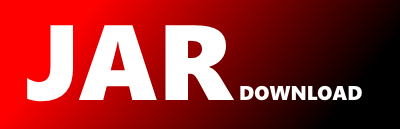
com.alipay.rdf.file.model.FileInfo Maven / Gradle / Ivy
package com.alipay.rdf.file.model;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
/**
* Copyright (C) 2013-2018 Ant Financial Services Group
*
* @author hongwei.quhw
* @version $Id: FileInfo.java, v 0.1 2018年3月12日 下午4:21:59 hongwei.quhw Exp $
*/
public class FileInfo {
/** 文件名 */
private String fileName;
/** 是否存在 */
private boolean exists;
/** 文件大小 */
private long size;
/** 最后修改时间*/
private Date lastModifiedDate;
/** 非用户自定义的元数据。 */
private final Map metadata = new HashMap();
/** 用户自定义的元数据,表示以x-oss-meta-为前缀的请求头。 */
private final Map userMetadata = new HashMap();
/**
* Getter method for property fileName.
*
* @return property value of exists
*/
public String getFileName() {
return fileName;
}
/**
* Setter method for property fileName.
*
* @return property value of exists
*/
public void setFileName(String fileName) {
this.fileName = fileName;
}
/**
* Getter method for property exists.
*
* @return property value of exists
*/
public boolean isExists() {
return exists;
}
/**
* Setter method for property exists.
*
* @param exists value to be assigned to property exists
*/
public void setExists(boolean exists) {
this.exists = exists;
}
/**
* Getter method for property size.
*
* @return property value of size
*/
public long getSize() {
return size;
}
/**
* Getter method for property lastModifiedDate.
*
* @return property value of lastModifiedDate
*/
public Date getLastModifiedDate() {
return lastModifiedDate;
}
/**
* Setter method for property lastModifiedDate.
*
* @param lastModifiedDate value to be assigned to property lastModifiedDate
*/
public void setLastModifiedDate(Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
* Setter method for property size.
*
* @param size value to be assigned to property size
*/
public void setSize(long size) {
this.size = size;
}
/**
* Getter method for property metadata.
*
* @return property value of metadata
*/
public Map getMetadata() {
return metadata;
}
/**
* 根据Key获取元数据
*
* @param key
* @return
*/
public Object getMetadata(String key) {
return metadata.get(key);
}
/**
* Getter method for property userMetadata.
*
* @return property value of userMetadata
*/
public Map getUserMetadata() {
return userMetadata;
}
/**
* 根据Key获取用户元数据
*
* @param key
* @return
*/
public String getUserMetadata(String key) {
return userMetadata.get(key);
}
@Override
public String toString() {
return "FileInfo[fileName=" + fileName + ", size=" + size + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy