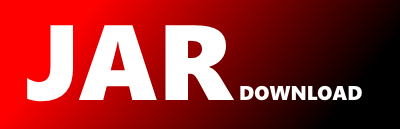
com.aliyun.datahub.client.http.TimeoutCache Maven / Gradle / Ivy
package com.aliyun.datahub.client.http;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class TimeoutCache {
private final Map> cache = new ConcurrentHashMap<>();
private long timeoutMs;
public TimeoutCache(long timeoutMs) {
this.timeoutMs = timeoutMs;
}
public void put(K key, V value) {
long now = System.currentTimeMillis();
for (Map.Entry> kvEntry : cache.entrySet()) {
if (kvEntry.getValue().isExpired(now)) {
cache.remove(kvEntry.getKey());
}
}
cache.put(key, new CacheItem<>(now, value));
}
public V get(K key) {
CacheItem item = cache.get(key);
if (item != null) {
item.visit();
return item.getValue();
}
return null;
}
public V remove(K key) {
CacheItem item = cache.remove(key);
if (item != null) {
return item.getValue();
}
return null;
}
private class CacheItem {
private volatile long timestamp;
private volatile boolean visited = true;
private T value;
CacheItem(long timestamp, T value) {
this.timestamp = timestamp;
this.value = value;
}
boolean isExpired(long timestamp) {
if (visited) {
this.timestamp = timestamp;
visited = false;
return false;
} else {
return timestamp - this.timestamp > timeoutMs;
}
}
void visit() {
this.visited = true;
}
public T getValue() {
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy