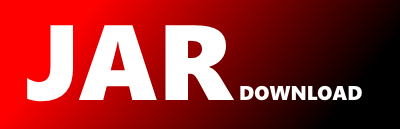
com.aliyun.datahub.client.http.common.HttpResponse Maven / Gradle / Ivy
package com.aliyun.datahub.client.http.common;
import com.aliyun.datahub.client.common.DatahubConstant;
import com.aliyun.datahub.client.common.HttpHeaders;
import com.aliyun.datahub.client.model.BaseResult;
import com.aliyun.datahub.client.util.JsonUtils;
import com.fasterxml.jackson.core.type.TypeReference;
import okhttp3.Response;
import java.util.HashMap;
import java.util.Map;
import static com.aliyun.datahub.client.http.common.Constants.CONTENT_JSON;
import static com.aliyun.datahub.client.http.common.Constants.CONTENT_PROTOBUF;
public class HttpResponse {
private int httpStatus;
private Map headers = new HashMap<>();
private String bodyStr;
public HttpResponse(HttpRequest request, Response response, String bodyStr) {
this.httpStatus = response.code();
this.bodyStr = bodyStr;
for (String name : response.headers().names()) {
headers.put(name.toLowerCase(), response.header(name));
}
}
public T getEntity(Class returnType) {
T result = null;
if (returnType != null) {
if (String.class.equals(returnType)) {
result = returnType.cast(bodyStr);
} else if (isMediaSupport()) {
result = JsonUtils.fromJson(bodyStr, returnType);
if (result != null && BaseResult.class.isAssignableFrom(returnType)) {
String requestId = headers.get(DatahubConstant.X_DATAHUB_REQUEST_ID);
((BaseResult)result).setRequestId(requestId);
}
}
}
return result;
}
public T getEntity(TypeReference returnType) {
T result = null;
if (returnType != null) {
if (isMediaSupport()) {
result = JsonUtils.fromJson(bodyStr, returnType);
}
}
return result;
}
private boolean isMediaSupport() {
String mediaType = headers.get(HttpHeaders.CONTENT_TYPE.toLowerCase());
return mediaType != null && (
mediaType.contains(CONTENT_JSON) || mediaType.contains(CONTENT_PROTOBUF));
}
public int getHttpStatus() {
return httpStatus;
}
public Map getHeaders() {
return headers;
}
public String getHeader(String key) {
return headers.get(key);
}
public String getBodyStr() {
return bodyStr;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy