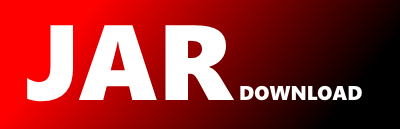
com.aliyun.datahub.client.model.AlarmRuleEntry Maven / Gradle / Ivy
package com.aliyun.datahub.client.model;
import com.aliyun.datahub.client.impl.serializer.AlarmRuleEntryDeserializer;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.Map;
@JsonDeserialize(using = AlarmRuleEntryDeserializer.class)
public class AlarmRuleEntry extends BaseResult {
@JsonProperty("RegionId")
private String regionId;
@JsonProperty("AlarmId")
private String alarmId;
@JsonProperty("ProjectName")
private String projectName;
@JsonProperty("TopicName")
private String topicName;
@JsonProperty("Uid")
private String uid;
@JsonProperty("AlarmType")
private AlarmType alarmType;
@JsonProperty("Rule")
private AlarmRule rule;
@JsonProperty("Config")
private Map config;
@JsonProperty("CreateTime")
private long createTime;
@JsonProperty("UpdateTime")
private long updateTime;
public String getAlarmId() {
return alarmId;
}
public void setAlarmId(String alarmId) {
this.alarmId = alarmId;
}
public String getProjectName() {
return projectName;
}
public void setProjectName(String projectName) {
this.projectName = projectName;
}
public String getTopicName() {
return topicName;
}
public void setTopicName(String topicName) {
this.topicName = topicName;
}
public AlarmType getAlarmType() {
return alarmType;
}
public void setAlarmType(AlarmType alarmType) {
this.alarmType = alarmType;
}
public AlarmRule getRule() {
return rule;
}
public void setRule(AlarmRule rule) {
this.rule = rule;
}
public Map getConfig() {
return config;
}
public void setConfig(Map config) {
this.config = config;
}
public long getCreateTime() {
return createTime;
}
public void setCreateTime(long createTime) {
this.createTime = createTime;
}
public long getUpdateTime() {
return updateTime;
}
public void setUpdateTime(long updateTime) {
this.updateTime = updateTime;
}
public String getRegionId() {
return regionId;
}
public void setRegionId(String regionId) {
this.regionId = regionId;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy