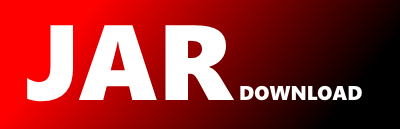
com.aliyun.datahub.model.serialize.SerializerFactory Maven / Gradle / Ivy
package com.aliyun.datahub.model.serialize;
import com.aliyun.datahub.common.transport.DefaultRequest;
import com.aliyun.datahub.common.transport.Response;
import com.aliyun.datahub.exception.DatahubClientException;
import com.aliyun.datahub.model.*;
public interface SerializerFactory {
public ErrorParser getErrorParser() throws DatahubClientException;
public Serializer getCreateProjectRequestSer() throws DatahubClientException;
public Deserializer getCreateProjectResultDeser() throws DatahubClientException;
public Serializer getDeleteProjectRequestSer() throws DatahubClientException;
public Deserializer getDeleteProjectResultDeser() throws DatahubClientException;
public Serializer getGetProjectRequestSer() throws DatahubClientException;
public Deserializer getGetProjectResultDeser() throws DatahubClientException;
public Serializer getListProjectRequestSer() throws DatahubClientException;
public Deserializer getListProjectResultDeser() throws DatahubClientException;
public Serializer getCreateTopicRequestSer() throws DatahubClientException;
public Deserializer getCreateTopicResultDeser() throws DatahubClientException;
public Serializer getDeleteTopicRequestSer() throws DatahubClientException;
public Deserializer getDeleteTopicResultDeser() throws DatahubClientException;
public Serializer getGetTopicRequestSer() throws DatahubClientException;
public Deserializer getGetTopicResultDeser() throws DatahubClientException;
public Serializer getListTopicRequestSer() throws DatahubClientException;
public Deserializer getListTopicResultDeser() throws DatahubClientException;
public Serializer getUpdateTopicRequestSer() throws DatahubClientException;
public Deserializer getUpdateTopicResultDeser() throws DatahubClientException;
public Serializer getListShardRequestSer() throws DatahubClientException;
public Deserializer getListShardResultDeser() throws DatahubClientException;
public Serializer getGetCursorRequestSer() throws DatahubClientException;
public Deserializer getGetCursorResultDeser() throws DatahubClientException;
public Serializer getGetRecordsRequestSer() throws DatahubClientException;
public Deserializer getGetRecordsResultDeser() throws DatahubClientException;
public Serializer getPutRecordsRequestSer() throws DatahubClientException;
public Deserializer getPutRecordsResultDeser() throws DatahubClientException;
public Serializer getSplitShardRequestSer() throws DatahubClientException;
public Deserializer getSplitShardResultDeser() throws DatahubClientException;
public Serializer getMergeShardRequestSer() throws DatahubClientException;
public Deserializer getMergeShardResultDeser() throws DatahubClientException;
public Serializer getListConnectorRequestSer() throws DatahubClientException;
public Deserializer getListConnectorResultDeser() throws DatahubClientException;
public Serializer getCreateConnectorRequestSer() throws DatahubClientException;
public Deserializer getCreateConnectorResultDeser() throws DatahubClientException;
public Serializer getGetConnectorRequestSer() throws DatahubClientException;
public Deserializer getGetConnectorResultDeser() throws DatahubClientException;
public Serializer getDeleteConnectorRequestSer() throws DatahubClientException;
public Deserializer getDeleteConnectorResultDeser() throws DatahubClientException;
public Serializer getReloadConnectorRequestSer() throws DatahubClientException;
public Deserializer getReloadConnectorResultDeser() throws DatahubClientException;
public Serializer getGetConnectorShardStatusRequestSer() throws DatahubClientException;
public Deserializer getGetConnectorShardStatusResultDeser() throws DatahubClientException;
public Serializer getGetBlobRecordsRequestSer() throws DatahubClientException;
public Deserializer getGetBlobRecordsResultDeser() throws DatahubClientException;
public Serializer getPutBlobRecordsRequestSer() throws DatahubClientException;
public Deserializer getPutBlobRecordsResultDeser() throws DatahubClientException;
public Serializer getExtendShardRequestSer() throws DatahubClientException;
public Deserializer getExtendShardResultDeser() throws DatahubClientException;
public Serializer getAppendFieldRequestSer() throws DatahubClientException;
public Deserializer getAppendFieldResultDeser() throws DatahubClientException;
public Serializer getAppendConnectorFieldRequestSer() throws DatahubClientException;
public Deserializer getAppendConnectorFieldResultDeser() throws DatahubClientException;
public Serializer getUpdateConnectorStateRequestSer() throws DatahubClientException;
public Deserializer getUpdateConnectorStateResultDeser() throws DatahubClientException;
public Serializer getUpdateConnectorShardContextRequestSer() throws DatahubClientException;
public Deserializer getUpdateConnectorShardContextResultDeser() throws DatahubClientException;
public Serializer getGetMeteringInfoRequestSer() throws DatahubClientException;
public Deserializer getGetMeteringInfoResultDeser() throws DatahubClientException;
public Serializer getListDataConnectorRequestSer() throws DatahubClientException;
public Deserializer getListDataConnectorResultDeser() throws DatahubClientException;
public Serializer getCreateDataConnectorRequestSer() throws DatahubClientException;
public Deserializer getCreateDataConnectorResultDeser() throws DatahubClientException;
public Serializer getGetDataConnectorRequestSer() throws DatahubClientException;
public Deserializer getGetDataConnectorResultDeser() throws DatahubClientException;
public Serializer getDeleteDataConnectorRequestSer() throws DatahubClientException;
public Deserializer getDeleteDataConnectorResultDeser() throws DatahubClientException;
public Serializer getReloadDataConnectorRequestSer() throws DatahubClientException;
public Deserializer getReloadDataConnectorResultDeser() throws DatahubClientException;
public Serializer getGetDataConnectorShardStatusRequestSer() throws DatahubClientException;
public Deserializer getGetDataConnectorShardStatusResultDeser() throws DatahubClientException;
public Serializer getAppendDataConnectorFieldRequestSer() throws DatahubClientException;
public Deserializer getAppendDataConnectorFieldResultDeser() throws DatahubClientException;
public Serializer getCreateSubscriptionRequestSer() throws DatahubClientException;
public Deserializer getCreateSubscriptionResultDeser() throws DatahubClientException;
public Serializer getDeleteSubscriptionRequestSer() throws DatahubClientException;
public Deserializer getDeleteSubscriptionResultDeser() throws DatahubClientException;
public Serializer getGetOffsetRequestSer() throws DatahubClientException;
public Deserializer getGetOffsetResultDeser() throws DatahubClientException;
public Serializer getGetSubscriptionRequestSer() throws DatahubClientException;
public Deserializer getGetSubscriptionResultDeser() throws DatahubClientException;
public Serializer getQuerySubscriptionRequestSer() throws DatahubClientException;
public Deserializer getQuerySubscriptionResultDeser() throws DatahubClientException;
public Serializer getCommitOffsetRequestSer() throws DatahubClientException;
public Deserializer getCommitOffsetResultDeser() throws DatahubClientException;
public Serializer getUpdateSubscriptionRequestSer() throws DatahubClientException;
public Deserializer getUpdateSubscriptionResultDerser() throws DatahubClientException;
public Serializer getGetDataConnectorDoneTimeRequestSer() throws DatahubClientException;
public Deserializer getGetDataConnectorDoneTimeResultDeser() throws DatahubClientException;
public Serializer getUpdateSubscriptionStateRequestSer() throws DatahubClientException;
public Deserializer getUpdateSubscriptionStateResultDerser() throws DatahubClientException;
public Serializer getInitOffsetContextRequestSer() throws DatahubClientException;
public Deserializer getInitOffsetContextResultDerser() throws DatahubClientException;
public Serializer getResetOffsetRequestSer() throws DatahubClientException;
public Deserializer getResetOffsetResultDeser() throws DatahubClientException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy