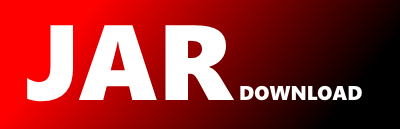
apsara.odps.lot.PythonTransformProtos Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: lot/pythontransform.proto
package apsara.odps.lot;
public final class PythonTransformProtos {
private PythonTransformProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface PythonTransformOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated group Resources = 1 {
java.util.List
getResourcesList();
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources getResources(int index);
int getResourcesCount();
java.util.List extends apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder>
getResourcesOrBuilderList();
apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder getResourcesOrBuilder(
int index);
// required string ClassName = 2;
boolean hasClassName();
String getClassName();
// repeated .apsara.odps.ReferencedURI URIs = 3;
java.util.List
getURIsList();
apsara.odps.ReferencedURIProtos.ReferencedURI getURIs(int index);
int getURIsCount();
java.util.List extends apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder>
getURIsOrBuilderList();
apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder getURIsOrBuilder(
int index);
}
public static final class PythonTransform extends
com.google.protobuf.GeneratedMessage
implements PythonTransformOrBuilder {
// Use PythonTransform.newBuilder() to construct.
private PythonTransform(Builder builder) {
super(builder);
}
private PythonTransform(boolean noInit) {}
private static final PythonTransform defaultInstance;
public static PythonTransform getDefaultInstance() {
return defaultInstance;
}
public PythonTransform getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_fieldAccessorTable;
}
public interface ResourcesOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string Project = 1;
boolean hasProject();
String getProject();
// required string ResourceName = 2;
boolean hasResourceName();
String getResourceName();
}
public static final class Resources extends
com.google.protobuf.GeneratedMessage
implements ResourcesOrBuilder {
// Use Resources.newBuilder() to construct.
private Resources(Builder builder) {
super(builder);
}
private Resources(boolean noInit) {}
private static final Resources defaultInstance;
public static Resources getDefaultInstance() {
return defaultInstance;
}
public Resources getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_Resources_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_Resources_fieldAccessorTable;
}
private int bitField0_;
// required string Project = 1;
public static final int PROJECT_FIELD_NUMBER = 1;
private java.lang.Object project_;
public boolean hasProject() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getProject() {
java.lang.Object ref = project_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
project_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getProjectBytes() {
java.lang.Object ref = project_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
project_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string ResourceName = 2;
public static final int RESOURCENAME_FIELD_NUMBER = 2;
private java.lang.Object resourceName_;
public boolean hasResourceName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
resourceName_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
project_ = "";
resourceName_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasProject()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasResourceName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getProjectBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getResourceNameBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getProjectBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getResourceNameBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_Resources_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_Resources_fieldAccessorTable;
}
// Construct using apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
project_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
resourceName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.getDescriptor();
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources getDefaultInstanceForType() {
return apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.getDefaultInstance();
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources build() {
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources buildPartial() {
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources result = new apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.project_ = project_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.resourceName_ = resourceName_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources) {
return mergeFrom((apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources other) {
if (other == apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.getDefaultInstance()) return this;
if (other.hasProject()) {
setProject(other.getProject());
}
if (other.hasResourceName()) {
setResourceName(other.getResourceName());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasProject()) {
return false;
}
if (!hasResourceName()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
project_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
resourceName_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required string Project = 1;
private java.lang.Object project_ = "";
public boolean hasProject() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getProject() {
java.lang.Object ref = project_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
project_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setProject(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
project_ = value;
onChanged();
return this;
}
public Builder clearProject() {
bitField0_ = (bitField0_ & ~0x00000001);
project_ = getDefaultInstance().getProject();
onChanged();
return this;
}
void setProject(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000001;
project_ = value;
onChanged();
}
// required string ResourceName = 2;
private java.lang.Object resourceName_ = "";
public boolean hasResourceName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
resourceName_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setResourceName(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
resourceName_ = value;
onChanged();
return this;
}
public Builder clearResourceName() {
bitField0_ = (bitField0_ & ~0x00000002);
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
void setResourceName(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
resourceName_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:apsara.odps.lot.PythonTransform.Resources)
}
static {
defaultInstance = new Resources(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:apsara.odps.lot.PythonTransform.Resources)
}
private int bitField0_;
// repeated group Resources = 1 {
public static final int RESOURCES_FIELD_NUMBER = 1;
private java.util.List resources_;
public java.util.List getResourcesList() {
return resources_;
}
public java.util.List extends apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder>
getResourcesOrBuilderList() {
return resources_;
}
public int getResourcesCount() {
return resources_.size();
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources getResources(int index) {
return resources_.get(index);
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder getResourcesOrBuilder(
int index) {
return resources_.get(index);
}
// required string ClassName = 2;
public static final int CLASSNAME_FIELD_NUMBER = 2;
private java.lang.Object className_;
public boolean hasClassName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getClassName() {
java.lang.Object ref = className_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
className_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getClassNameBytes() {
java.lang.Object ref = className_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
className_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated .apsara.odps.ReferencedURI URIs = 3;
public static final int URIS_FIELD_NUMBER = 3;
private java.util.List uRIs_;
public java.util.List getURIsList() {
return uRIs_;
}
public java.util.List extends apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder>
getURIsOrBuilderList() {
return uRIs_;
}
public int getURIsCount() {
return uRIs_.size();
}
public apsara.odps.ReferencedURIProtos.ReferencedURI getURIs(int index) {
return uRIs_.get(index);
}
public apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder getURIsOrBuilder(
int index) {
return uRIs_.get(index);
}
private void initFields() {
resources_ = java.util.Collections.emptyList();
className_ = "";
uRIs_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasClassName()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getResourcesCount(); i++) {
if (!getResources(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getURIsCount(); i++) {
if (!getURIs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < resources_.size(); i++) {
output.writeGroup(1, resources_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(2, getClassNameBytes());
}
for (int i = 0; i < uRIs_.size(); i++) {
output.writeMessage(3, uRIs_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < resources_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(1, resources_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getClassNameBytes());
}
for (int i = 0; i < uRIs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, uRIs_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static apsara.odps.lot.PythonTransformProtos.PythonTransform parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(apsara.odps.lot.PythonTransformProtos.PythonTransform prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements apsara.odps.lot.PythonTransformProtos.PythonTransformOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return apsara.odps.lot.PythonTransformProtos.internal_static_apsara_odps_lot_PythonTransform_fieldAccessorTable;
}
// Construct using apsara.odps.lot.PythonTransformProtos.PythonTransform.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getResourcesFieldBuilder();
getURIsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (resourcesBuilder_ == null) {
resources_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
resourcesBuilder_.clear();
}
className_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (uRIsBuilder_ == null) {
uRIs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
uRIsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return apsara.odps.lot.PythonTransformProtos.PythonTransform.getDescriptor();
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform getDefaultInstanceForType() {
return apsara.odps.lot.PythonTransformProtos.PythonTransform.getDefaultInstance();
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform build() {
apsara.odps.lot.PythonTransformProtos.PythonTransform result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private apsara.odps.lot.PythonTransformProtos.PythonTransform buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
apsara.odps.lot.PythonTransformProtos.PythonTransform result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform buildPartial() {
apsara.odps.lot.PythonTransformProtos.PythonTransform result = new apsara.odps.lot.PythonTransformProtos.PythonTransform(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (resourcesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
resources_ = java.util.Collections.unmodifiableList(resources_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.resources_ = resources_;
} else {
result.resources_ = resourcesBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.className_ = className_;
if (uRIsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
uRIs_ = java.util.Collections.unmodifiableList(uRIs_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.uRIs_ = uRIs_;
} else {
result.uRIs_ = uRIsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof apsara.odps.lot.PythonTransformProtos.PythonTransform) {
return mergeFrom((apsara.odps.lot.PythonTransformProtos.PythonTransform)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(apsara.odps.lot.PythonTransformProtos.PythonTransform other) {
if (other == apsara.odps.lot.PythonTransformProtos.PythonTransform.getDefaultInstance()) return this;
if (resourcesBuilder_ == null) {
if (!other.resources_.isEmpty()) {
if (resources_.isEmpty()) {
resources_ = other.resources_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureResourcesIsMutable();
resources_.addAll(other.resources_);
}
onChanged();
}
} else {
if (!other.resources_.isEmpty()) {
if (resourcesBuilder_.isEmpty()) {
resourcesBuilder_.dispose();
resourcesBuilder_ = null;
resources_ = other.resources_;
bitField0_ = (bitField0_ & ~0x00000001);
resourcesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getResourcesFieldBuilder() : null;
} else {
resourcesBuilder_.addAllMessages(other.resources_);
}
}
}
if (other.hasClassName()) {
setClassName(other.getClassName());
}
if (uRIsBuilder_ == null) {
if (!other.uRIs_.isEmpty()) {
if (uRIs_.isEmpty()) {
uRIs_ = other.uRIs_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureURIsIsMutable();
uRIs_.addAll(other.uRIs_);
}
onChanged();
}
} else {
if (!other.uRIs_.isEmpty()) {
if (uRIsBuilder_.isEmpty()) {
uRIsBuilder_.dispose();
uRIsBuilder_ = null;
uRIs_ = other.uRIs_;
bitField0_ = (bitField0_ & ~0x00000004);
uRIsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getURIsFieldBuilder() : null;
} else {
uRIsBuilder_.addAllMessages(other.uRIs_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasClassName()) {
return false;
}
for (int i = 0; i < getResourcesCount(); i++) {
if (!getResources(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getURIsCount(); i++) {
if (!getURIs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 11: {
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder subBuilder = apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.newBuilder();
input.readGroup(1, subBuilder, extensionRegistry);
addResources(subBuilder.buildPartial());
break;
}
case 18: {
bitField0_ |= 0x00000002;
className_ = input.readBytes();
break;
}
case 26: {
apsara.odps.ReferencedURIProtos.ReferencedURI.Builder subBuilder = apsara.odps.ReferencedURIProtos.ReferencedURI.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addURIs(subBuilder.buildPartial());
break;
}
}
}
}
private int bitField0_;
// repeated group Resources = 1 {
private java.util.List resources_ =
java.util.Collections.emptyList();
private void ensureResourcesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
resources_ = new java.util.ArrayList(resources_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder, apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder> resourcesBuilder_;
public java.util.List getResourcesList() {
if (resourcesBuilder_ == null) {
return java.util.Collections.unmodifiableList(resources_);
} else {
return resourcesBuilder_.getMessageList();
}
}
public int getResourcesCount() {
if (resourcesBuilder_ == null) {
return resources_.size();
} else {
return resourcesBuilder_.getCount();
}
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources getResources(int index) {
if (resourcesBuilder_ == null) {
return resources_.get(index);
} else {
return resourcesBuilder_.getMessage(index);
}
}
public Builder setResources(
int index, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcesIsMutable();
resources_.set(index, value);
onChanged();
} else {
resourcesBuilder_.setMessage(index, value);
}
return this;
}
public Builder setResources(
int index, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder builderForValue) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.set(index, builderForValue.build());
onChanged();
} else {
resourcesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
public Builder addResources(apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcesIsMutable();
resources_.add(value);
onChanged();
} else {
resourcesBuilder_.addMessage(value);
}
return this;
}
public Builder addResources(
int index, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcesIsMutable();
resources_.add(index, value);
onChanged();
} else {
resourcesBuilder_.addMessage(index, value);
}
return this;
}
public Builder addResources(
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder builderForValue) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.add(builderForValue.build());
onChanged();
} else {
resourcesBuilder_.addMessage(builderForValue.build());
}
return this;
}
public Builder addResources(
int index, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder builderForValue) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.add(index, builderForValue.build());
onChanged();
} else {
resourcesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
public Builder addAllResources(
java.lang.Iterable extends apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources> values) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
super.addAll(values, resources_);
onChanged();
} else {
resourcesBuilder_.addAllMessages(values);
}
return this;
}
public Builder clearResources() {
if (resourcesBuilder_ == null) {
resources_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
resourcesBuilder_.clear();
}
return this;
}
public Builder removeResources(int index) {
if (resourcesBuilder_ == null) {
ensureResourcesIsMutable();
resources_.remove(index);
onChanged();
} else {
resourcesBuilder_.remove(index);
}
return this;
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder getResourcesBuilder(
int index) {
return getResourcesFieldBuilder().getBuilder(index);
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder getResourcesOrBuilder(
int index) {
if (resourcesBuilder_ == null) {
return resources_.get(index); } else {
return resourcesBuilder_.getMessageOrBuilder(index);
}
}
public java.util.List extends apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder>
getResourcesOrBuilderList() {
if (resourcesBuilder_ != null) {
return resourcesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(resources_);
}
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder addResourcesBuilder() {
return getResourcesFieldBuilder().addBuilder(
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.getDefaultInstance());
}
public apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder addResourcesBuilder(
int index) {
return getResourcesFieldBuilder().addBuilder(
index, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.getDefaultInstance());
}
public java.util.List
getResourcesBuilderList() {
return getResourcesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder, apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder>
getResourcesFieldBuilder() {
if (resourcesBuilder_ == null) {
resourcesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources, apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder, apsara.odps.lot.PythonTransformProtos.PythonTransform.ResourcesOrBuilder>(
resources_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
resources_ = null;
}
return resourcesBuilder_;
}
// required string ClassName = 2;
private java.lang.Object className_ = "";
public boolean hasClassName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getClassName() {
java.lang.Object ref = className_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
className_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setClassName(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
className_ = value;
onChanged();
return this;
}
public Builder clearClassName() {
bitField0_ = (bitField0_ & ~0x00000002);
className_ = getDefaultInstance().getClassName();
onChanged();
return this;
}
void setClassName(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
className_ = value;
onChanged();
}
// repeated .apsara.odps.ReferencedURI URIs = 3;
private java.util.List uRIs_ =
java.util.Collections.emptyList();
private void ensureURIsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
uRIs_ = new java.util.ArrayList(uRIs_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
apsara.odps.ReferencedURIProtos.ReferencedURI, apsara.odps.ReferencedURIProtos.ReferencedURI.Builder, apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder> uRIsBuilder_;
public java.util.List getURIsList() {
if (uRIsBuilder_ == null) {
return java.util.Collections.unmodifiableList(uRIs_);
} else {
return uRIsBuilder_.getMessageList();
}
}
public int getURIsCount() {
if (uRIsBuilder_ == null) {
return uRIs_.size();
} else {
return uRIsBuilder_.getCount();
}
}
public apsara.odps.ReferencedURIProtos.ReferencedURI getURIs(int index) {
if (uRIsBuilder_ == null) {
return uRIs_.get(index);
} else {
return uRIsBuilder_.getMessage(index);
}
}
public Builder setURIs(
int index, apsara.odps.ReferencedURIProtos.ReferencedURI value) {
if (uRIsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureURIsIsMutable();
uRIs_.set(index, value);
onChanged();
} else {
uRIsBuilder_.setMessage(index, value);
}
return this;
}
public Builder setURIs(
int index, apsara.odps.ReferencedURIProtos.ReferencedURI.Builder builderForValue) {
if (uRIsBuilder_ == null) {
ensureURIsIsMutable();
uRIs_.set(index, builderForValue.build());
onChanged();
} else {
uRIsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
public Builder addURIs(apsara.odps.ReferencedURIProtos.ReferencedURI value) {
if (uRIsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureURIsIsMutable();
uRIs_.add(value);
onChanged();
} else {
uRIsBuilder_.addMessage(value);
}
return this;
}
public Builder addURIs(
int index, apsara.odps.ReferencedURIProtos.ReferencedURI value) {
if (uRIsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureURIsIsMutable();
uRIs_.add(index, value);
onChanged();
} else {
uRIsBuilder_.addMessage(index, value);
}
return this;
}
public Builder addURIs(
apsara.odps.ReferencedURIProtos.ReferencedURI.Builder builderForValue) {
if (uRIsBuilder_ == null) {
ensureURIsIsMutable();
uRIs_.add(builderForValue.build());
onChanged();
} else {
uRIsBuilder_.addMessage(builderForValue.build());
}
return this;
}
public Builder addURIs(
int index, apsara.odps.ReferencedURIProtos.ReferencedURI.Builder builderForValue) {
if (uRIsBuilder_ == null) {
ensureURIsIsMutable();
uRIs_.add(index, builderForValue.build());
onChanged();
} else {
uRIsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
public Builder addAllURIs(
java.lang.Iterable extends apsara.odps.ReferencedURIProtos.ReferencedURI> values) {
if (uRIsBuilder_ == null) {
ensureURIsIsMutable();
super.addAll(values, uRIs_);
onChanged();
} else {
uRIsBuilder_.addAllMessages(values);
}
return this;
}
public Builder clearURIs() {
if (uRIsBuilder_ == null) {
uRIs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
uRIsBuilder_.clear();
}
return this;
}
public Builder removeURIs(int index) {
if (uRIsBuilder_ == null) {
ensureURIsIsMutable();
uRIs_.remove(index);
onChanged();
} else {
uRIsBuilder_.remove(index);
}
return this;
}
public apsara.odps.ReferencedURIProtos.ReferencedURI.Builder getURIsBuilder(
int index) {
return getURIsFieldBuilder().getBuilder(index);
}
public apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder getURIsOrBuilder(
int index) {
if (uRIsBuilder_ == null) {
return uRIs_.get(index); } else {
return uRIsBuilder_.getMessageOrBuilder(index);
}
}
public java.util.List extends apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder>
getURIsOrBuilderList() {
if (uRIsBuilder_ != null) {
return uRIsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(uRIs_);
}
}
public apsara.odps.ReferencedURIProtos.ReferencedURI.Builder addURIsBuilder() {
return getURIsFieldBuilder().addBuilder(
apsara.odps.ReferencedURIProtos.ReferencedURI.getDefaultInstance());
}
public apsara.odps.ReferencedURIProtos.ReferencedURI.Builder addURIsBuilder(
int index) {
return getURIsFieldBuilder().addBuilder(
index, apsara.odps.ReferencedURIProtos.ReferencedURI.getDefaultInstance());
}
public java.util.List
getURIsBuilderList() {
return getURIsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
apsara.odps.ReferencedURIProtos.ReferencedURI, apsara.odps.ReferencedURIProtos.ReferencedURI.Builder, apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder>
getURIsFieldBuilder() {
if (uRIsBuilder_ == null) {
uRIsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
apsara.odps.ReferencedURIProtos.ReferencedURI, apsara.odps.ReferencedURIProtos.ReferencedURI.Builder, apsara.odps.ReferencedURIProtos.ReferencedURIOrBuilder>(
uRIs_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
uRIs_ = null;
}
return uRIsBuilder_;
}
// @@protoc_insertion_point(builder_scope:apsara.odps.lot.PythonTransform)
}
static {
defaultInstance = new PythonTransform(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:apsara.odps.lot.PythonTransform)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_apsara_odps_lot_PythonTransform_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_apsara_odps_lot_PythonTransform_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_apsara_odps_lot_PythonTransform_Resources_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_apsara_odps_lot_PythonTransform_Resources_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\031lot/pythontransform.proto\022\017apsara.odps" +
".lot\032\032common/referenceduri.proto\"\301\001\n\017Pyt" +
"honTransform\022=\n\tresources\030\001 \003(\n2*.apsara" +
".odps.lot.PythonTransform.Resources\022\021\n\tC" +
"lassName\030\002 \002(\t\022(\n\004URIs\030\003 \003(\0132\032.apsara.od" +
"ps.ReferencedURI\0322\n\tResources\022\017\n\007Project" +
"\030\001 \002(\t\022\024\n\014ResourceName\030\002 \002(\tB\027B\025PythonTr" +
"ansformProtos"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_apsara_odps_lot_PythonTransform_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_apsara_odps_lot_PythonTransform_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_apsara_odps_lot_PythonTransform_descriptor,
new java.lang.String[] { "Resources", "ClassName", "URIs", },
apsara.odps.lot.PythonTransformProtos.PythonTransform.class,
apsara.odps.lot.PythonTransformProtos.PythonTransform.Builder.class);
internal_static_apsara_odps_lot_PythonTransform_Resources_descriptor =
internal_static_apsara_odps_lot_PythonTransform_descriptor.getNestedTypes().get(0);
internal_static_apsara_odps_lot_PythonTransform_Resources_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_apsara_odps_lot_PythonTransform_Resources_descriptor,
new java.lang.String[] { "Project", "ResourceName", },
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.class,
apsara.odps.lot.PythonTransformProtos.PythonTransform.Resources.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
apsara.odps.ReferencedURIProtos.getDescriptor(),
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy