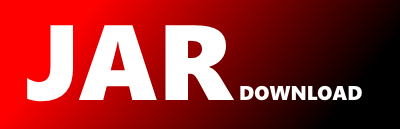
com.aliyun.odps.Sessions Maven / Gradle / Ivy
package com.aliyun.odps;
import com.aliyun.odps.commons.transport.Response;
import com.aliyun.odps.rest.ResourceBuilder;
import com.aliyun.odps.rest.RestClient;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Created by dongxiao on 2019/8/26.
*/
public class Sessions implements Iterable {
private static Gson gson = new GsonBuilder().disableHtmlEscaping().create();
private RestClient client;
private Odps odps;
public Sessions(Odps odps) {
this.odps = odps;
this.client = odps.getRestClient();
}
private class SessionListIterator extends ListIterator {
private boolean inited = false;
@Override
protected List list() {
// TODO add limit for list api, there is no need to do it currently
if (inited) {
return null;
}
List list = new ArrayList<>();
try {
Response resp = client.request(ResourceBuilder.buildSessionsResource(odps.getDefaultProject()), "GET", null, null, null);
Type type = new TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy