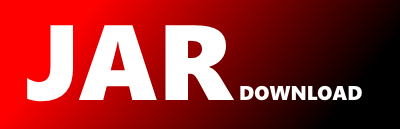
com.aliyun.odps.sqa.QueryInfo Maven / Gradle / Ivy
The newest version!
package com.aliyun.odps.sqa;
import com.aliyun.odps.Instance;
import com.aliyun.odps.sqa.commandapi.CommandInfo;
import com.aliyun.odps.utils.StringUtils;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Created by dongxiao on 2020/3/16.
*/
public class QueryInfo {
// SESSION subquery id
private int id = -1;
private int retry = 0;
private boolean isSelect = true;
private String sql;
private Map hint;
// offline: SQLTASK instance
// online: SQLRT instance
private Instance instance = null;
private ExecuteMode executeMode = ExecuteMode.INTERACTIVE;
private List executionLog = new ArrayList<>();
private CommandInfo commandInfo = null;
public QueryInfo(String sql, Map hint, ExecuteMode executeMode) {
this.sql = sql;
this.hint = hint;
this.executeMode = executeMode;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getRetry() {
synchronized (this) {
return retry;
}
}
public void incRetry() {
synchronized (this) {
this.retry++;
}
}
public String getSql() {
return sql;
}
public Map getHint() {
return hint;
}
public void setHint(Map hint) {
this.hint = hint;
}
public Instance getInstance() {
synchronized (this) {
return instance;
}
}
public ExecuteMode getExecuteMode() {
synchronized (this) {
return executeMode;
}
}
public void setExecuteMode(ExecuteMode mode) {
synchronized (this) {
executeMode = mode;
}
}
public void setInstance(Instance instance, ExecuteMode executeMode, String logview, String rerunMessage) {
synchronized (this) {
this.instance = instance;
this.executeMode = executeMode;
if (!StringUtils.isNullOrEmpty(rerunMessage)) {
// rerun or fallback
executionLog.add("Query failed:" + rerunMessage);
if (executeMode.equals(ExecuteMode.OFFLINE)) {
executionLog.add("Will fallback to offline mode");
} else {
executionLog.add("Will rerun in interactive mode");
}
}
executionLog.add("Running in " + executeMode.toString().toLowerCase()
+ " mode, RetryCount: " + retry + ", QueryId:" + id + "\nLog view:");
executionLog.add(logview);
}
}
public boolean isSelect() {
return isSelect;
}
public void setSelect(boolean select) {
isSelect = select;
}
public List getAndCleanExecutionLog() {
synchronized (this) {
List log = new ArrayList<>();
log.addAll(executionLog);
executionLog.clear();
return log;
}
}
public CommandInfo getCommandInfo() {
return commandInfo;
}
public void setCommandInfo(CommandInfo commandInfo) {
this.commandInfo = commandInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy