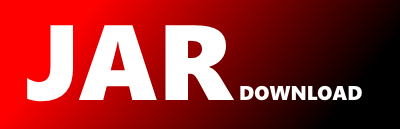
com.aliyun.openservices.log.request.ListTopostoreNodeRelationRequest Maven / Gradle / Ivy
package com.aliyun.openservices.log.request;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.aliyun.openservices.log.common.Consts;
public class ListTopostoreNodeRelationRequest{
private String topostoreName;
// node selection
private List nodeIds = new ArrayList();
private List nodeTypes = new ArrayList();
private Map nodeProperties = new HashMap();
// relation selection
private List relationTypes;
private long relationDepth = 0;
private String relationDirection = Consts.TOPOSTORE_RELATION_DIRECTION_BOTH;
private long from;
private long to;
// extParams;
private Map params = new HashMap();
public ListTopostoreNodeRelationRequest() {
}
public ListTopostoreNodeRelationRequest(String topostoreName) {
this(topostoreName, new ArrayList(), new ArrayList(), new HashMap(), new ArrayList(), 0, Consts.TOPOSTORE_RELATION_DIRECTION_BOTH);
}
public ListTopostoreNodeRelationRequest(String topostoreName, List nodeIds) {
this(topostoreName, nodeIds, new ArrayList(), new HashMap(), new ArrayList(), 0, Consts.TOPOSTORE_RELATION_DIRECTION_BOTH);
}
public ListTopostoreNodeRelationRequest(String topostoreName, List nodeIds, int relationDepth) {
this(topostoreName, nodeIds, new ArrayList(), new HashMap(), new ArrayList(), relationDepth, Consts.TOPOSTORE_RELATION_DIRECTION_BOTH);
}
public ListTopostoreNodeRelationRequest(String topostoreName, List nodeIds, List nodeTypes,
Map nodeProperities, List relationTypes, int relationDepth, String relationDirection) {
this.topostoreName = topostoreName;
if(nodeIds!=null){
this.nodeIds = nodeIds;
}
if(nodeTypes!=null){
this.nodeTypes = nodeTypes;
}
if(nodeProperities!=null){
this.nodeProperties = nodeProperities;
}
if(relationTypes!=null){
this.relationTypes = relationTypes;
}
this.relationDepth = relationDepth;
this.relationDirection = relationDirection;
}
public long getFrom() {
return this.from;
}
public void setFrom(long from) {
this.from = from;
}
public long getTo() {
return this.to;
}
public void setTo(long to) {
this.to = to;
}
public String getTopostoreName() {
return this.topostoreName;
}
public void setTopostoreName(String topostoreName) {
this.topostoreName = topostoreName;
}
public List getNodeIds() {
return this.nodeIds;
}
public void setNodeIds(String... nodeIds ){
this.nodeIds = Arrays.asList(nodeIds);
}
public void setNodeTypes(String... nodeTypes ){
this.nodeTypes = Arrays.asList(nodeTypes);
}
public void setRelationTypes(String... relationTypes ){
this.relationTypes = Arrays.asList(relationTypes);
}
public ListTopostoreNodeRelationRequest addNodeProperty(String key, String value){
this.nodeProperties.put(key, value);
return this;
}
public void setNodeIds(List nodeIds) {
if(nodeIds == null){
return;
}
this.nodeIds = nodeIds;
}
public void SetParam(String key, String value) {
this.params.put(key, value);
}
public Map GetParam() {
return this.params;
}
public List getNodeTypes() {
return this.nodeTypes;
}
public void setNodeTypes(List nodeTypes) {
if(nodeTypes == null){
return;
}
this.nodeTypes = nodeTypes;
}
public Map getNodeProperities() {
return this.nodeProperties;
}
public void setNodeProperities(Map nodeProperities) {
if(nodeProperities == null){
return;
}
this.nodeProperties = nodeProperities;
}
public List getRelationTypes() {
return this.relationTypes;
}
public void setRelationTypes(List relationTypes) {
this.relationTypes = relationTypes;
}
public long getDepth() {
return this.relationDepth;
}
public void setDepth(long depth) {
this.relationDepth = depth;
}
public String getDirection() {
return this.relationDirection;
}
public void setDirection(String direction) {
this.relationDirection = direction;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy