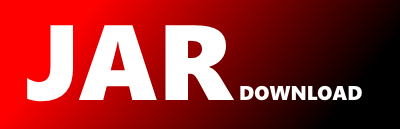
com.aliyun.openservices.log.request.ListTopostoreNodeRequest Maven / Gradle / Ivy
package com.aliyun.openservices.log.request;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Base64;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.alibaba.fastjson.JSONObject;
import com.aliyun.openservices.log.common.Consts;
import com.aliyun.openservices.log.util.Utils;
public class ListTopostoreNodeRequest extends TopostoreRequest {
private String topostoreName;
private List nodeIds = new ArrayList();
private List nodeTypes = new ArrayList();
private String propertyKey;
private String propertyValue;
private Map properties = new HashMap();
public Map getProperties() {
return this.properties;
}
public void setProperties(Map properties) {
this.properties = properties;
}
private Integer offset=0;
private Integer size=200;
public ListTopostoreNodeRequest() {
}
public ListTopostoreNodeRequest(String topostoreName) {
this(topostoreName, new ArrayList(), new ArrayList(), "", "", 0, 200);
}
public ListTopostoreNodeRequest(String topostoreName, List nodeIds) {
this(topostoreName, nodeIds, new ArrayList(), "", "", 0, 200);
}
public ListTopostoreNodeRequest(String topostoreName, List nodeIds, List nodeTypes,
String propertyKey, String propertyValue, Integer offset, Integer size) {
this.topostoreName = topostoreName;
this.nodeIds = nodeIds;
this.nodeTypes = nodeTypes;
this.propertyKey = propertyKey;
this.propertyValue = propertyValue;
this.offset = offset;
this.size = size;
}
public ListTopostoreNodeRequest(String topostoreName,String propertyKey, String propertyValue) {
this(topostoreName, new ArrayList(), new ArrayList(), propertyKey, propertyValue, 0, 200);
}
public String getTopostoreName() {
return this.topostoreName;
}
public void setTopostoreName(String topostoreName) {
this.topostoreName = topostoreName;
}
public List getNodeIds() {
return this.nodeIds;
}
public void setNodeIds(List nodeIds) {
if (nodeIds == null) {
return ;
}
this.nodeIds = nodeIds;
}
public void setNodeIds(String... nodeIds){
this.nodeIds = Arrays.asList(nodeIds);
}
public ListTopostoreNodeRequest addNodeIds(String nodeId){
this.nodeIds.add(nodeId);
return this;
}
public void setNodeTypes(String... nodeTypes){
this.nodeTypes = Arrays.asList(nodeTypes);
}
public List getNodeTypes() {
return this.nodeTypes;
}
public void setNodeTypes(List nodeTypes) {
if(nodeTypes == null ){
return ;
}
this.nodeTypes = nodeTypes;
}
public ListTopostoreNodeRequest addProperties(String key, String value){
this.properties.put(key, value);
return this;
}
public String getPropertyKey() {
return this.propertyKey;
}
public void setPropertyKey(String propertyKey) {
this.propertyKey = propertyKey;
}
public String getPropertyValue() {
return this.propertyValue;
}
public void setPropertyValue(String propertyValue) {
this.propertyValue = propertyValue;
}
public Integer getOffset() {
return this.offset;
}
public void setOffset(Integer offset) {
this.offset = offset;
}
public Integer getSize() {
return this.size;
}
public void setSize(Integer size) {
this.size = size;
}
@Override
public Map GetAllParams() {
if (offset != null) {
SetParam(Consts.CONST_OFFSET, offset.toString());
}
if (size != null) {
SetParam(Consts.CONST_SIZE, size.toString());
}
if(nodeIds != null && !nodeIds.isEmpty()){
SetParam(Consts.TOPOSTORE_NODE_ID_LIST, Utils.join(",", Utils.removeNullItems(nodeIds)));
}
if(nodeTypes != null && !nodeTypes.isEmpty()){
SetParam(Consts.TOPOSTORE_NODE_TYPE_LIST, Utils.join(",", Utils.removeNullItems(nodeTypes)));
}
if(propertyKey!=null && propertyKey.length()>0){
SetParam(Consts.TOPOSTORE_NODE_PROPERTY_KEY, propertyKey);
}
if(propertyValue!= null && propertyValue.length()>0){
SetParam(Consts.TOPOSTORE_NODE_PROPERTY_VALUE, propertyValue);
}
if(properties!=null && !properties.isEmpty()){
JSONObject proObj = new JSONObject();
for(Map.Entry kv : properties.entrySet()){
proObj.put(kv.getKey(), kv.getValue());
}
try{
SetParam(Consts.TOPOSTORE_NODE_PROPERTIES, URLEncoder.encode(new String(
Base64.getEncoder().encodeToString(proObj.toJSONString().getBytes())), "utf-8"));
} catch(UnsupportedEncodingException e){
throw new RuntimeException(e);
}
}
return super.GetAllParams();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy