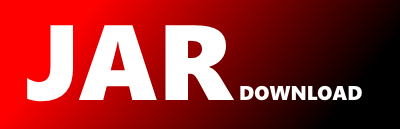
com.aliyun.openservices.log.request.ListTopostoreRelationRequest Maven / Gradle / Ivy
package com.aliyun.openservices.log.request;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Base64;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.alibaba.fastjson.JSONObject;
import com.aliyun.openservices.log.common.Consts;
import com.aliyun.openservices.log.util.Utils;
public class ListTopostoreRelationRequest extends TopostoreRequest {
private String topostoreName;
private List relationIds = new ArrayList();
private List relationTypes = new ArrayList();
private List srcNodeIds = new ArrayList();
private List dstNodeIds = new ArrayList();
private String propertyKey = "";
private String propertyValue = "";
private Integer offset=0;
private Integer size=200;
private Map properties = new HashMap();
public Map getProperties() {
return this.properties;
}
public void setProperties(Map properties) {
if(properties==null){
return;
}
this.properties = properties;
}
public ListTopostoreRelationRequest() {
}
public ListTopostoreRelationRequest(String topostoreName) {
this(topostoreName, new ArrayList(),new ArrayList(), "", "", 0, 200 );
}
public ListTopostoreRelationRequest(String topostoreName, List relationIds) {
this(topostoreName, relationIds,new ArrayList(), "", "", 0, 200 );
}
public ListTopostoreRelationRequest(String topostoreName, List relationIds, List relationTypes,
String propertyKey, String propertyValue, Integer offset, Integer size) {
this.topostoreName = topostoreName;
if(relationIds != null ){
this.relationIds = relationIds;
}
if(relationTypes!=null){
this.relationTypes = relationTypes;
}
this.propertyKey = propertyKey;
this.propertyValue = propertyValue;
this.offset = offset;
this.size = size;
}
public ListTopostoreRelationRequest(String topostoreName,String propertyKey, String propertyValue) {
this(topostoreName, new ArrayList(),new ArrayList(), propertyKey, propertyValue, 0, 200 );
}
public String getTopostoreName() {
return this.topostoreName;
}
public void setTopostoreName(String topostoreName) {
this.topostoreName = topostoreName;
}
public List getRelationIds() {
return this.relationIds;
}
public void setRelationIds(List relationIds) {
if(relationIds == null ) {
return ;
}
this.relationIds = relationIds;
}
public ListTopostoreRelationRequest addProperties(String key, String value){
this.properties.put(key, value);
return this;
}
public void setRelationIds(String... relationIds){
this.relationIds = Arrays.asList(relationIds);
}
public ListTopostoreRelationRequest addRelationId(String relationId){
this.relationIds.add(relationId);
return this;
}
public void setRelationTypes(String... relationTypes){
this.relationTypes = Arrays.asList(relationTypes);
}
public ListTopostoreRelationRequest addRelationType(String relationType){
this.relationTypes.add(relationType);
return this;
}
public void setSrcNodeIds(String... srcNodeIds){
this.srcNodeIds = Arrays.asList(srcNodeIds);
}
public ListTopostoreRelationRequest addSrcNodeId(String srcNodeId){
this.srcNodeIds.add(srcNodeId);
return this;
}
public void setDstNodeIds(String... dstNodeIds){
this.dstNodeIds = Arrays.asList(dstNodeIds);
}
public ListTopostoreRelationRequest addDstNodeId(String dstNodeId){
this.dstNodeIds.add(dstNodeId);
return this;
}
public List getRelationTypes() {
return this.relationTypes;
}
public void setRelationTypes(List relationTypes) {
if(relationTypes == null ) {
return ;
}
this.relationTypes = relationTypes;
}
public String getPropertyKey() {
return this.propertyKey;
}
public void setPropertyKey(String propertyKey) {
this.propertyKey = propertyKey;
}
public String getPropertyValue() {
return this.propertyValue;
}
public void setPropertyValue(String propertyValue) {
this.propertyValue = propertyValue;
}
public Integer getOffset() {
return this.offset;
}
public void setOffset(Integer offset) {
this.offset = offset;
}
public Integer getSize() {
return this.size;
}
public void setSize(Integer size) {
this.size = size;
}
public List getSrcNodeIds() {
return this.srcNodeIds;
}
public void setSrcNodeIds(List srcNodeIds) {
if(srcNodeIds == null ) {
return ;
}
this.srcNodeIds = srcNodeIds;
}
public List getDstNodeIds() {
return this.dstNodeIds;
}
public void setDstNodeIds(List dstNodeIds) {
if(dstNodeIds == null ) {
return ;
}
this.dstNodeIds = dstNodeIds;
}
@Override
public Map GetAllParams() {
if (offset != null) {
SetParam(Consts.CONST_OFFSET, offset.toString());
}
if (size != null) {
SetParam(Consts.CONST_SIZE, size.toString());
}
if(relationIds != null && !relationIds.isEmpty()){
SetParam(Consts.TOPOSTORE_RELATION_ID_LIST, Utils.join(",", Utils.removeNullItems(relationIds)));
}
if(relationTypes != null && !relationTypes.isEmpty()){
SetParam(Consts.TOPOSTORE_RELATION_TYPE_LIST, Utils.join(",", Utils.removeNullItems(relationTypes)));
}
if(srcNodeIds != null && !srcNodeIds.isEmpty()){
SetParam(Consts.TOPOSTORE_RELATION_SRC_NODE_ID_LIST, Utils.join(",", Utils.removeNullItems(srcNodeIds)));
}
if(dstNodeIds != null && !dstNodeIds.isEmpty()){
SetParam(Consts.TOPOSTORE_RELATION_DST_NODE_ID_LIST, Utils.join(",", Utils.removeNullItems(dstNodeIds)));
}
if(propertyKey!=null&&propertyKey.length()>0){
SetParam(Consts.TOPOSTORE_RELATION_PROPERTY_KEY, propertyKey);
}
if(propertyValue!=null&&propertyValue.length()>0){
SetParam(Consts.TOPOSTORE_RELATION_PROPERTY_VALUE, propertyValue);
}
if(properties!=null && !properties.isEmpty()){
JSONObject proObj = new JSONObject();
for(Map.Entry kv : properties.entrySet()){
proObj.put(kv.getKey(), kv.getValue());
}
try{
SetParam(Consts.TOPOSTORE_RELATION_PROPERTIES, URLEncoder.encode(new String(
Base64.getEncoder().encodeToString(proObj.toJSONString().getBytes())), "utf-8"));
} catch(UnsupportedEncodingException e){
throw new RuntimeException(e);
}
}
return super.GetAllParams();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy