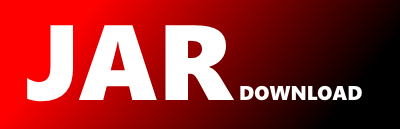
com.aliyun.openservices.ons.api.exactlyonce.aop.proxy.MQTransactionScaner Maven / Gradle / Ivy
package com.aliyun.openservices.ons.api.exactlyonce.aop.proxy;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.HashSet;
import com.aliyun.openservices.ons.api.exactlyonce.aop.annotation.MQTransaction;
import com.aliyun.openservices.ons.api.exactlyonce.manager.util.AopUtil;
import org.springframework.aop.Advisor;
import org.springframework.aop.TargetSource;
import org.springframework.aop.framework.AdvisedSupport;
import org.springframework.aop.support.AopUtils;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationEvent;
import org.springframework.context.event.ContextRefreshedEvent;
import static com.aliyun.openservices.ons.api.exactlyonce.manager.util.AopUtil.findTargetClass;
import static com.aliyun.openservices.ons.api.exactlyonce.manager.util.AopUtil.getAdvisedSupport;
/**
* @author gongshi
*/
public class MQTransactionScaner extends AbstractMQTransactionScanner {
private boolean enableTransaction = true;
private MQTransactionInterceptor interceptor;
private HashSet proxyMethodSet = new HashSet();
private boolean isEnableTransaction = true;
private void init() {
// TransactionManager.start();
}
@Override
protected Object wrapIfNecessary(Object bean, String beanName, Object cacheKey) {
synchronized (proxyMethodSet) {
if (proxyMethodSet.contains(beanName)) {
return bean;
}
proxyMethodSet.add(beanName);
}
try {
Class> targetInterface = findTargetClass(bean);
Method[] methods = targetInterface.getMethods();
HashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy