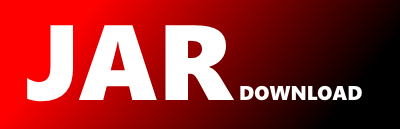
com.aliyun.openservices.shade.io.opentelemetry.api.common.ArrayBackedAttributes Maven / Gradle / Ivy
/*
* Copyright The OpenTelemetry Authors
* SPDX-License-Identifier: Apache-2.0
*/
package com.aliyun.openservices.shade.com.aliyun.openservices.shade.io.opentelemetry.api.common;
import com.aliyun.openservices.shade.com.aliyun.openservices.shade.io.opentelemetry.api.internal.ImmutableKeyValuePairs;
import java.util.ArrayList;
import java.util.Comparator;
import javax.annotation.Nullable;
import javax.annotation.concurrent.Immutable;
@Immutable
final class ArrayBackedAttributes extends ImmutableKeyValuePairs, Object>
implements Attributes {
// We only compare the key name, not type, when constructing, to allow deduping keys with the
// same name but different type.
private static final Comparator> KEY_COMPARATOR_FOR_CONSTRUCTION =
Comparator.comparing(AttributeKey::getKey);
static final Attributes EMPTY = Attributes.builder().build();
private ArrayBackedAttributes(Object[] data, Comparator> keyComparator) {
super(data, keyComparator);
}
/**
* Only use this constructor if you can guarantee that the data has been de-duped, sorted by key
* and contains no null values or null/empty keys.
*
* @param data the raw data
*/
ArrayBackedAttributes(Object[] data) {
super(data);
}
@Override
public AttributesBuilder toBuilder() {
return new ArrayBackedAttributesBuilder(new ArrayList<>(data()));
}
@SuppressWarnings("unchecked")
@Override
@Nullable
public T get(AttributeKey key) {
return (T) super.get(key);
}
static Attributes sortAndFilterToAttributes(Object... data) {
// null out any empty keys or keys with null values
// so they will then be removed by the sortAndFilter method.
for (int i = 0; i < data.length; i += 2) {
AttributeKey> key = (AttributeKey>) data[i];
if (key != null && key.getKey().isEmpty()) {
data[i] = null;
}
}
return new ArrayBackedAttributes(data, KEY_COMPARATOR_FOR_CONSTRUCTION);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy