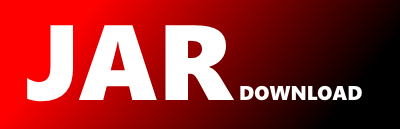
com.aliyun.oss.OSSClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-sdk-oss-shade Show documentation
Show all versions of aliyun-sdk-oss-shade Show documentation
The Aliyun OSS SDK for Java used for accessing Aliyun Object Storage Service, includes all service and dependent JARs.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.aliyun.oss;
import static com.aliyun.oss.common.utils.CodingUtils.assertParameterNotNull;
import static com.aliyun.oss.common.utils.IOUtils.checkFile;
import static com.aliyun.oss.common.utils.LogUtils.logException;
import static com.aliyun.oss.internal.OSSConstants.DEFAULT_CHARSET_NAME;
import static com.aliyun.oss.internal.OSSConstants.DEFAULT_OSS_ENDPOINT;
import static com.aliyun.oss.internal.OSSUtils.OSS_RESOURCE_MANAGER;
import static com.aliyun.oss.internal.OSSUtils.ensureBucketNameValid;
import static com.aliyun.oss.internal.OSSUtils.ensureObjectKeyValidEx;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.InetAddress;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URL;
import java.net.UnknownHostException;
import java.util.Date;
import java.util.List;
import java.util.Map;
import com.aliyun.oss.common.auth.Credentials;
import com.aliyun.oss.common.auth.CredentialsProvider;
import com.aliyun.oss.common.auth.DefaultCredentialProvider;
import com.aliyun.oss.common.auth.ServiceSignature;
import com.aliyun.oss.common.comm.*;
import com.aliyun.oss.common.utils.BinaryUtil;
import com.aliyun.oss.common.utils.DateUtil;
import com.aliyun.oss.internal.*;
import com.aliyun.oss.model.*;
import com.aliyun.oss.model.SetBucketCORSRequest.CORSRule;
import com.aliyun.oss.model.InventoryConfiguration;
/**
* The entry point class of OSS that implements the OSS interface.
*/
public class OSSClient implements OSS {
/* The default credentials provider */
private CredentialsProvider credsProvider;
/* The valid endpoint for accessing to OSS services */
private URI endpoint;
/* The default service client */
private ServiceClient serviceClient;
/* The miscellaneous OSS operations */
private OSSBucketOperation bucketOperation;
private OSSObjectOperation objectOperation;
private OSSMultipartOperation multipartOperation;
private CORSOperation corsOperation;
private OSSUploadOperation uploadOperation;
private OSSDownloadOperation downloadOperation;
private LiveChannelOperation liveChannelOperation;
/**Gets the inner multipartOperation, used for subclass to do implement opreation.
* @return the {@link OSSMultipartOperation} instance.
*/
public OSSMultipartOperation getMultipartOperation() {
return multipartOperation;
}
/**Gets the inner objectOperation, used for subclass to do implement opreation.
* @return the {@link OSSObjectOperation} instance.
*/
public OSSObjectOperation getObjectOperation() {
return objectOperation;
}
/**Sets the inner downloadOperation.
* @param downloadOperation
* the {@link OSSDownloadOperation} instance.
*/
public void setDownloadOperation(OSSDownloadOperation downloadOperation) {
this.downloadOperation = downloadOperation;
}
/**Sets the inner uploadOperation.
* @param uploadOperation
* the {@link OSSUploadOperation} instance.
*/
public void setUploadOperation(OSSUploadOperation uploadOperation) {
this.uploadOperation = uploadOperation;
}
/**
* Uses the default OSS Endpoint(http://oss-cn-hangzhou.aliyuncs.com) and
* Access Id/Access Key to create a new {@link OSSClient} instance.
*
* @param accessKeyId
* Access Key ID.
* @param secretAccessKey
* Secret Access Key.
*/
@Deprecated
public OSSClient(String accessKeyId, String secretAccessKey) {
this(DEFAULT_OSS_ENDPOINT, new DefaultCredentialProvider(accessKeyId, secretAccessKey));
}
/**
* Uses the specified OSS Endpoint and Access Id/Access Key to create a new
* {@link OSSClient} instance.
*
* @param endpoint
* OSS endpoint.
* @param accessKeyId
* Access Key ID.
* @param secretAccessKey
* Secret Access Key.
*/
@Deprecated
public OSSClient(String endpoint, String accessKeyId, String secretAccessKey) {
this(endpoint, new DefaultCredentialProvider(accessKeyId, secretAccessKey), null);
}
/**
* Uses the specified OSS Endpoint、a security token from AliCloud STS and
* Access Id/Access Key to create a new {@link OSSClient} instance.
*
* @param endpoint
* OSS Endpoint.
* @param accessKeyId
* Access Id from STS.
* @param secretAccessKey
* Access Key from STS
* @param securityToken
* Security Token from STS.
*/
@Deprecated
public OSSClient(String endpoint, String accessKeyId, String secretAccessKey, String securityToken) {
this(endpoint, new DefaultCredentialProvider(accessKeyId, secretAccessKey, securityToken), null);
}
/**
* Uses a specified OSS Endpoint、Access Id, Access Key、Client side
* configuration to create a {@link OSSClient} instance.
*
* @param endpoint
* OSS Endpoint。
* @param accessKeyId
* Access Key ID。
* @param secretAccessKey
* Secret Access Key。
* @param config
* A {@link ClientConfiguration} instance. The method would use
* default configuration if it's null.
*/
@Deprecated
public OSSClient(String endpoint, String accessKeyId, String secretAccessKey, ClientConfiguration config) {
this(endpoint, new DefaultCredentialProvider(accessKeyId, secretAccessKey), config);
}
/**
* Uses specified OSS Endpoint, the temporary (Access Id/Access Key/Security
* Token) from STS and the client configuration to create a new
* {@link OSSClient} instance.
*
* @param endpoint
* OSS Endpoint。
* @param accessKeyId
* Access Key Id provided by STS.
* @param secretAccessKey
* Secret Access Key provided by STS.
* @param securityToken
* Security token provided by STS.
* @param config
* A {@link ClientConfiguration} instance. The method would use
* default configuration if it's null.
*/
@Deprecated
public OSSClient(String endpoint, String accessKeyId, String secretAccessKey, String securityToken,
ClientConfiguration config) {
this(endpoint, new DefaultCredentialProvider(accessKeyId, secretAccessKey, securityToken), config);
}
/**
* Uses the specified {@link CredentialsProvider} and OSS Endpoint to create
* a new {@link OSSClient} instance.
*
* @param endpoint
* OSS services Endpoint。
* @param credsProvider
* Credentials provider which has access key Id and access Key
* secret.
*/
@Deprecated
public OSSClient(String endpoint, CredentialsProvider credsProvider) {
this(endpoint, credsProvider, null);
}
/**
* Uses the specified {@link CredentialsProvider}, client configuration and
* OSS endpoint to create a new {@link OSSClient} instance.
*
* @param endpoint
* OSS services Endpoint.
* @param credsProvider
* Credentials provider.
* @param config
* client configuration.
*/
public OSSClient(String endpoint, CredentialsProvider credsProvider, ClientConfiguration config) {
this.credsProvider = credsProvider;
config = config == null ? new ClientConfiguration() : config;
if (config.isRequestTimeoutEnabled()) {
this.serviceClient = new TimeoutServiceClient(config);
} else {
this.serviceClient = new DefaultServiceClient(config);
}
initOperations();
setEndpoint(endpoint);
initDefaultsByEndpoint();
}
/**
* Gets OSS services Endpoint.
*
* @return OSS services Endpoint.
*/
public synchronized URI getEndpoint() {
return URI.create(endpoint.toString());
}
/**
* Sets OSS services endpoint.
*
* @param endpoint
* OSS services endpoint.
*/
public synchronized void setEndpoint(String endpoint) {
URI uri = toURI(endpoint);
this.endpoint = uri;
OSSUtils.ensureEndpointValid(uri.getHost());
if (isIpOrLocalhost(uri)) {
serviceClient.getClientConfiguration().setSLDEnabled(true);
}
this.bucketOperation.setEndpoint(uri);
this.objectOperation.setEndpoint(uri);
this.multipartOperation.setEndpoint(uri);
this.corsOperation.setEndpoint(uri);
this.liveChannelOperation.setEndpoint(uri);
}
/**
* Checks if the uri is an IP or domain. If it's IP or local host, then it
* will use secondary domain of Alibaba cloud. Otherwise, it will use domain
* directly to access the OSS.
*
* @param uri
* URI。
*/
private boolean isIpOrLocalhost(URI uri) {
if (uri.getHost().equals("localhost")) {
return true;
}
InetAddress ia;
try {
ia = InetAddress.getByName(uri.getHost());
} catch (UnknownHostException e) {
return false;
}
if (uri.getHost().equals(ia.getHostAddress())) {
return true;
}
return false;
}
private boolean isCloudBoxEndpointSuffix(URI uri) {
if (uri == null || uri.getHost() == null) {
return false;
}
String host = uri.getHost();
if (host.endsWith("oss-cloudbox.aliyuncs.com") ||
host.endsWith("oss-cloudbox-control.aliyuncs.com")) {
return true;
}
return false;
}
private boolean isVerifyObjectStrict() {
ClientConfiguration conf = serviceClient.getClientConfiguration();
if (conf.signatureVersion == null || SignVersion.V1.equals(conf.signatureVersion)) {
return conf.isVerifyObjectStrict();
}
return false;
}
private URI toURI(String endpoint) throws IllegalArgumentException {
return OSSUtils.toEndpointURI(endpoint, this.serviceClient.getClientConfiguration().getProtocol().toString());
}
private void initOperations() {
this.bucketOperation = new OSSBucketOperation(this.serviceClient, this.credsProvider);
this.objectOperation = new OSSObjectOperation(this.serviceClient, this.credsProvider);
this.multipartOperation = new OSSMultipartOperation(this.serviceClient, this.credsProvider);
this.corsOperation = new CORSOperation(this.serviceClient, this.credsProvider);
this.uploadOperation = new OSSUploadOperation(this.multipartOperation);
this.downloadOperation = new OSSDownloadOperation(objectOperation);
this.liveChannelOperation = new LiveChannelOperation(this.serviceClient, this.credsProvider);
}
private void initDefaultsByEndpoint() {
if (!this.serviceClient.getClientConfiguration().isExtractSettingFromEndpointEnable()) {
return;
}
//cloud box endpoint pattern: cloudbox-id.region.oss-cloudbox[-control].aliyuncs.com
//cloudbox-id start with cb-
if (isCloudBoxEndpointSuffix(this.endpoint)) {
String host = this.endpoint.getHost();
String[] keys = host.split("\\.");
if (keys != null && keys.length == 5) {
if (keys[0].startsWith("cb-")) {
this.setCloudBoxId(keys[0]);
this.setRegion(keys[1]);
this.setProduct(OSSConstants.PRODUCT_CLOUD_BOX);
if (SignVersion.V4.compareTo(this.serviceClient.getClientConfiguration().getSignatureVersion()) > 0) {
this.setSignatureVersionInner(SignVersion.V4);
}
}
}
}
}
private void setSignatureVersionInner(SignVersion version) {
this.bucketOperation.setSignVersion(version);
this.objectOperation.setSignVersion(version);
this.multipartOperation.setSignVersion(version);
this.corsOperation.setSignVersion(version);
this.liveChannelOperation.setSignVersion(version);
}
/**
* Sets the product name.
*
* @param product
* the product name, ex oss.
*/
public void setProduct(String product) {
this.bucketOperation.setProduct(product);
this.objectOperation.setProduct(product);
this.multipartOperation.setProduct(product);
this.corsOperation.setProduct(product);
this.liveChannelOperation.setProduct(product);
}
/**
* Sets OSS services Region.
*
* @param region
* OSS services Region.
*/
public void setRegion(String region) {
this.bucketOperation.setRegion(region);
this.objectOperation.setRegion(region);
this.multipartOperation.setRegion(region);
this.corsOperation.setRegion(region);
this.liveChannelOperation.setRegion(region);
}
/**
* Sets OSS cloud box id.
*
* @param cloudBoxId OSS cloud box id.
*/
public void setCloudBoxId(String cloudBoxId) {
this.bucketOperation.setCloudBoxId(cloudBoxId);
this.objectOperation.setCloudBoxId(cloudBoxId);
this.multipartOperation.setCloudBoxId(cloudBoxId);
this.corsOperation.setCloudBoxId(cloudBoxId);
this.liveChannelOperation.setCloudBoxId(cloudBoxId);
}
@Override
public void switchCredentials(Credentials creds) {
if (creds == null) {
throw new IllegalArgumentException("creds should not be null.");
}
this.credsProvider.setCredentials(creds);
}
@Override
public void switchSignatureVersion(SignVersion signatureVersion) {
if (signatureVersion == null) {
throw new IllegalArgumentException("signatureVersion should not be null.");
}
this.getClientConfiguration().setSignatureVersion(signatureVersion);
}
public CredentialsProvider getCredentialsProvider() {
return this.credsProvider;
}
public ClientConfiguration getClientConfiguration() {
return serviceClient.getClientConfiguration();
}
@Override
public Bucket createBucket(String bucketName) throws OSSException, ClientException {
return this.createBucket(new CreateBucketRequest(bucketName));
}
@Override
public Bucket createBucket(CreateBucketRequest createBucketRequest) throws OSSException, ClientException {
return bucketOperation.createBucket(createBucketRequest);
}
@Override
public VoidResult deleteBucket(String bucketName) throws OSSException, ClientException {
return this.deleteBucket(new GenericRequest(bucketName));
}
@Override
public VoidResult deleteBucket(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.deleteBucket(genericRequest);
}
@Override
public List listBuckets() throws OSSException, ClientException {
return bucketOperation.listBuckets();
}
@Override
public BucketList listBuckets(ListBucketsRequest listBucketsRequest) throws OSSException, ClientException {
return bucketOperation.listBuckets(listBucketsRequest);
}
@Override
public BucketList listBuckets(String prefix, String marker, Integer maxKeys) throws OSSException, ClientException {
return bucketOperation.listBuckets(new ListBucketsRequest(prefix, marker, maxKeys));
}
@Override
public VoidResult setBucketAcl(String bucketName, CannedAccessControlList cannedACL)
throws OSSException, ClientException {
return this.setBucketAcl(new SetBucketAclRequest(bucketName, cannedACL));
}
@Override
public VoidResult setBucketAcl(SetBucketAclRequest setBucketAclRequest) throws OSSException, ClientException {
return bucketOperation.setBucketAcl(setBucketAclRequest);
}
@Override
public AccessControlList getBucketAcl(String bucketName) throws OSSException, ClientException {
return this.getBucketAcl(new GenericRequest(bucketName));
}
@Override
public AccessControlList getBucketAcl(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.getBucketAcl(genericRequest);
}
@Override
public BucketMetadata getBucketMetadata(String bucketName) throws OSSException, ClientException {
return this.getBucketMetadata(new GenericRequest(bucketName));
}
@Override
public BucketMetadata getBucketMetadata(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.getBucketMetadata(genericRequest);
}
@Override
public VoidResult setBucketReferer(String bucketName, BucketReferer referer) throws OSSException, ClientException {
return this.setBucketReferer(new SetBucketRefererRequest(bucketName, referer));
}
@Override
public VoidResult setBucketReferer(SetBucketRefererRequest setBucketRefererRequest) throws OSSException, ClientException {
return bucketOperation.setBucketReferer(setBucketRefererRequest);
}
@Override
public BucketReferer getBucketReferer(String bucketName) throws OSSException, ClientException {
return this.getBucketReferer(new GenericRequest(bucketName));
}
@Override
public BucketReferer getBucketReferer(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.getBucketReferer(genericRequest);
}
@Override
public String getBucketLocation(String bucketName) throws OSSException, ClientException {
return this.getBucketLocation(new GenericRequest(bucketName));
}
@Override
public String getBucketLocation(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.getBucketLocation(genericRequest);
}
@Override
public boolean doesBucketExist(String bucketName) throws OSSException, ClientException {
return this.doesBucketExist(new GenericRequest(bucketName));
}
@Override
public boolean doesBucketExist(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.doesBucketExists(genericRequest);
}
@Deprecated
public boolean isBucketExist(String bucketName) throws OSSException, ClientException {
return this.doesBucketExist(bucketName);
}
@Override
public ObjectListing listObjects(String bucketName) throws OSSException, ClientException {
return listObjects(new ListObjectsRequest(bucketName, null, null, null, null));
}
@Override
public ObjectListing listObjects(String bucketName, String prefix) throws OSSException, ClientException {
return listObjects(new ListObjectsRequest(bucketName, prefix, null, null, null));
}
@Override
public ObjectListing listObjects(ListObjectsRequest listObjectsRequest) throws OSSException, ClientException {
return bucketOperation.listObjects(listObjectsRequest);
}
@Override
public ListObjectsV2Result listObjectsV2(ListObjectsV2Request listObjectsV2Request) throws OSSException, ClientException {
return bucketOperation.listObjectsV2(listObjectsV2Request);
}
@Override
public ListObjectsV2Result listObjectsV2(String bucketName) throws OSSException, ClientException {
return bucketOperation.listObjectsV2(new ListObjectsV2Request(bucketName));
}
@Override
public ListObjectsV2Result listObjectsV2(String bucketName, String prefix) throws OSSException, ClientException {
return bucketOperation.listObjectsV2(new ListObjectsV2Request(bucketName, prefix));
}
@Override
public ListObjectsV2Result listObjectsV2(String bucketName, String prefix, String continuationToken,
String startAfter, String delimiter, Integer maxKeys,
String encodingType, boolean fetchOwner) throws OSSException, ClientException {
return bucketOperation.listObjectsV2(new ListObjectsV2Request(bucketName, prefix, continuationToken, startAfter,
delimiter, maxKeys, encodingType, fetchOwner));
}
@Override
public VersionListing listVersions(String bucketName, String prefix) throws OSSException, ClientException {
return listVersions(new ListVersionsRequest(bucketName, prefix, null, null, null, null));
}
@Override
public VersionListing listVersions(String bucketName, String prefix, String keyMarker, String versionIdMarker,
String delimiter, Integer maxResults) throws OSSException, ClientException {
ListVersionsRequest request = new ListVersionsRequest()
.withBucketName(bucketName)
.withPrefix(prefix)
.withDelimiter(delimiter)
.withKeyMarker(keyMarker)
.withVersionIdMarker(versionIdMarker)
.withMaxResults(maxResults);
return listVersions(request);
}
@Override
public VersionListing listVersions(ListVersionsRequest listVersionsRequest) throws OSSException, ClientException {
return bucketOperation.listVersions(listVersionsRequest);
}
@Override
public PutObjectResult putObject(String bucketName, String key, InputStream input)
throws OSSException, ClientException {
return putObject(bucketName, key, input, null);
}
@Override
public PutObjectResult putObject(String bucketName, String key, InputStream input, ObjectMetadata metadata)
throws OSSException, ClientException {
return putObject(new PutObjectRequest(bucketName, key, input, metadata));
}
@Override
public PutObjectResult putObject(String bucketName, String key, File file, ObjectMetadata metadata)
throws OSSException, ClientException {
return putObject(new PutObjectRequest(bucketName, key, file, metadata));
}
@Override
public PutObjectResult putObject(String bucketName, String key, File file) throws OSSException, ClientException {
return putObject(bucketName, key, file, null);
}
@Override
public PutObjectResult putObject(PutObjectRequest putObjectRequest) throws OSSException, ClientException {
return objectOperation.putObject(putObjectRequest);
}
@Override
public PutObjectResult putObject(URL signedUrl, String filePath, Map requestHeaders)
throws OSSException, ClientException {
return putObject(signedUrl, filePath, requestHeaders, false);
}
@Override
public PutObjectResult putObject(URL signedUrl, String filePath, Map requestHeaders,
boolean useChunkEncoding) throws OSSException, ClientException {
FileInputStream requestContent = null;
try {
File toUpload = new File(filePath);
if (!checkFile(toUpload)) {
throw new IllegalArgumentException("Illegal file path: " + filePath);
}
long fileSize = toUpload.length();
requestContent = new FileInputStream(toUpload);
return putObject(signedUrl, requestContent, fileSize, requestHeaders, useChunkEncoding);
} catch (FileNotFoundException e) {
throw new ClientException(e);
} finally {
if (requestContent != null) {
try {
requestContent.close();
} catch (IOException e) {
}
}
}
}
@Override
public PutObjectResult putObject(URL signedUrl, InputStream requestContent, long contentLength,
Map requestHeaders) throws OSSException, ClientException {
return putObject(signedUrl, requestContent, contentLength, requestHeaders, false);
}
@Override
public PutObjectResult putObject(URL signedUrl, InputStream requestContent, long contentLength,
Map requestHeaders, boolean useChunkEncoding) throws OSSException, ClientException {
return objectOperation.putObject(signedUrl, requestContent, contentLength, requestHeaders, useChunkEncoding);
}
@Override
public CopyObjectResult copyObject(String sourceBucketName, String sourceKey, String destinationBucketName,
String destinationKey) throws OSSException, ClientException {
return copyObject(new CopyObjectRequest(sourceBucketName, sourceKey, destinationBucketName, destinationKey));
}
@Override
public CopyObjectResult copyObject(CopyObjectRequest copyObjectRequest) throws OSSException, ClientException {
return objectOperation.copyObject(copyObjectRequest);
}
@Override
public OSSObject getObject(String bucketName, String key) throws OSSException, ClientException {
return this.getObject(new GetObjectRequest(bucketName, key));
}
@Override
public ObjectMetadata getObject(GetObjectRequest getObjectRequest, File file) throws OSSException, ClientException {
return objectOperation.getObject(getObjectRequest, file);
}
@Override
public OSSObject getObject(GetObjectRequest getObjectRequest) throws OSSException, ClientException {
return objectOperation.getObject(getObjectRequest);
}
@Override
public OSSObject getObject(URL signedUrl, Map requestHeaders) throws OSSException, ClientException {
GetObjectRequest getObjectRequest = new GetObjectRequest(signedUrl, requestHeaders);
return objectOperation.getObject(getObjectRequest);
}
@Override
public OSSObject selectObject(SelectObjectRequest selectObjectRequest) throws OSSException, ClientException {
return objectOperation.selectObject(selectObjectRequest);
}
@Override
public SimplifiedObjectMeta getSimplifiedObjectMeta(String bucketName, String key)
throws OSSException, ClientException {
return this.getSimplifiedObjectMeta(new GenericRequest(bucketName, key));
}
@Override
public SimplifiedObjectMeta getSimplifiedObjectMeta(GenericRequest genericRequest)
throws OSSException, ClientException {
return this.objectOperation.getSimplifiedObjectMeta(genericRequest);
}
@Override
public ObjectMetadata getObjectMetadata(String bucketName, String key) throws OSSException, ClientException {
return this.getObjectMetadata(new GenericRequest(bucketName, key));
}
@Override
public SelectObjectMetadata createSelectObjectMetadata(CreateSelectObjectMetadataRequest createSelectObjectMetadataRequest) throws OSSException, ClientException {
return objectOperation.createSelectObjectMetadata(createSelectObjectMetadataRequest);
}
@Override
public ObjectMetadata getObjectMetadata(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.getObjectMetadata(genericRequest);
}
@Override
public ObjectMetadata headObject(String bucketName, String key) throws OSSException, ClientException {
return this.headObject(new HeadObjectRequest(bucketName, key));
}
@Override
public ObjectMetadata headObject(HeadObjectRequest headObjectRequest) throws OSSException, ClientException {
return objectOperation.headObject(headObjectRequest);
}
@Override
public AppendObjectResult appendObject(AppendObjectRequest appendObjectRequest)
throws OSSException, ClientException {
return objectOperation.appendObject(appendObjectRequest);
}
@Override
public VoidResult deleteObject(String bucketName, String key) throws OSSException, ClientException {
return this.deleteObject(new GenericRequest(bucketName, key));
}
@Override
public VoidResult deleteObject(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.deleteObject(genericRequest);
}
@Override
public VoidResult deleteVersion(String bucketName, String key, String versionId) throws OSSException, ClientException {
return deleteVersion(new DeleteVersionRequest(bucketName, key, versionId));
}
@Override
public VoidResult deleteVersion(DeleteVersionRequest deleteVersionRequest) throws OSSException, ClientException {
return objectOperation.deleteVersion(deleteVersionRequest);
}
@Override
public DeleteObjectsResult deleteObjects(DeleteObjectsRequest deleteObjectsRequest)
throws OSSException, ClientException {
return objectOperation.deleteObjects(deleteObjectsRequest);
}
@Override
public DeleteVersionsResult deleteVersions(DeleteVersionsRequest deleteVersionsRequest)
throws OSSException, ClientException {
return objectOperation.deleteVersions(deleteVersionsRequest);
}
@Override
public boolean doesObjectExist(String bucketName, String key) throws OSSException, ClientException {
return doesObjectExist(new GenericRequest(bucketName, key));
}
@Override
public boolean doesObjectExist(String bucketName, String key, boolean isOnlyInOSS) {
if (isOnlyInOSS) {
return doesObjectExist(bucketName, key);
} else {
return objectOperation.doesObjectExistWithRedirect(new GenericRequest(bucketName, key));
}
}
@Deprecated
@Override
public boolean doesObjectExist(HeadObjectRequest headObjectRequest) throws OSSException, ClientException {
return doesObjectExist(new GenericRequest(headObjectRequest.getBucketName(), headObjectRequest.getKey()));
}
@Override
public boolean doesObjectExist(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.doesObjectExist(genericRequest);
}
@Override
public boolean doesObjectExist(GenericRequest genericRequest, boolean isOnlyInOSS) throws OSSException, ClientException {
if (isOnlyInOSS) {
return objectOperation.doesObjectExist(genericRequest);
} else {
return objectOperation.doesObjectExistWithRedirect(genericRequest);
}
}
@Override
public VoidResult setObjectAcl(String bucketName, String key, CannedAccessControlList cannedACL)
throws OSSException, ClientException {
return this.setObjectAcl(new SetObjectAclRequest(bucketName, key, cannedACL));
}
@Override
public VoidResult setObjectAcl(SetObjectAclRequest setObjectAclRequest) throws OSSException, ClientException {
return objectOperation.setObjectAcl(setObjectAclRequest);
}
@Override
public ObjectAcl getObjectAcl(String bucketName, String key) throws OSSException, ClientException {
return this.getObjectAcl(new GenericRequest(bucketName, key));
}
@Override
public ObjectAcl getObjectAcl(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.getObjectAcl(genericRequest);
}
@Override
public RestoreObjectResult restoreObject(String bucketName, String key) throws OSSException, ClientException {
return this.restoreObject(new GenericRequest(bucketName, key));
}
@Override
public RestoreObjectResult restoreObject(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.restoreObject(genericRequest);
}
@Override
public RestoreObjectResult restoreObject(String bucketName, String key, RestoreConfiguration restoreConfiguration)
throws OSSException, ClientException {
return this.restoreObject(new RestoreObjectRequest(bucketName, key, restoreConfiguration));
}
@Override
public RestoreObjectResult restoreObject(RestoreObjectRequest restoreObjectRequest)
throws OSSException, ClientException {
return objectOperation.restoreObject(restoreObjectRequest);
}
@Override
public VoidResult setObjectTagging(String bucketName, String key, Map tags)
throws OSSException, ClientException {
return this.setObjectTagging(new SetObjectTaggingRequest(bucketName, key, tags));
}
@Override
public VoidResult setObjectTagging(String bucketName, String key, TagSet tagSet) throws OSSException, ClientException {
return this.setObjectTagging(new SetObjectTaggingRequest(bucketName, key, tagSet));
}
@Override
public VoidResult setObjectTagging(SetObjectTaggingRequest setObjectTaggingRequest) throws OSSException, ClientException {
return objectOperation.setObjectTagging(setObjectTaggingRequest);
}
@Override
public TagSet getObjectTagging(String bucketName, String key) throws OSSException, ClientException {
return this.getObjectTagging(new GenericRequest(bucketName, key));
}
@Override
public TagSet getObjectTagging(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.getObjectTagging(genericRequest);
}
@Override
public VoidResult deleteObjectTagging(String bucketName, String key) throws OSSException, ClientException {
return this.deleteObjectTagging(new GenericRequest(bucketName, key));
}
@Override
public VoidResult deleteObjectTagging(GenericRequest genericRequest) throws OSSException, ClientException {
return objectOperation.deleteObjectTagging(genericRequest);
}
@Override
public URL generatePresignedUrl(String bucketName, String key, Date expiration) throws ClientException {
return generatePresignedUrl(bucketName, key, expiration, HttpMethod.GET);
}
@Override
public URL generatePresignedUrl(String bucketName, String key, Date expiration, HttpMethod method)
throws ClientException {
GeneratePresignedUrlRequest request = new GeneratePresignedUrlRequest(bucketName, key);
request.setExpiration(expiration);
request.setMethod(method);
return generatePresignedUrl(request);
}
@Override
public URL generatePresignedUrl(GeneratePresignedUrlRequest request) throws ClientException {
assertParameterNotNull(request, "request");
if (request.getBucketName() == null) {
throw new IllegalArgumentException(OSS_RESOURCE_MANAGER.getString("MustSetBucketName"));
}
ensureBucketNameValid(request.getBucketName());
assertParameterNotNull(request.getKey(), "key");
ensureObjectKeyValidEx(request.getKey(), isVerifyObjectStrict());
if (request.getExpiration() == null) {
throw new IllegalArgumentException(OSS_RESOURCE_MANAGER.getString("MustSetExpiration"));
}
String url;
if (SignVersion.V1.equals(serviceClient.getClientConfiguration().getSignatureVersion())) {
url = SignUtils.buildSignedURL(request, credsProvider.getCredentials(), serviceClient.getClientConfiguration(), endpoint);
} else if (SignVersion.V2.equals(serviceClient.getClientConfiguration().getSignatureVersion())) {
url = SignV2Utils.buildSignedURL(request, credsProvider.getCredentials(), serviceClient.getClientConfiguration(), endpoint);
} else {
return objectOperation.generatePresignedUrl(request);
}
try {
return new URL(url);
} catch (MalformedURLException e) {
throw new ClientException(e);
}
}
@Override
public VoidResult abortMultipartUpload(AbortMultipartUploadRequest request) throws OSSException, ClientException {
return multipartOperation.abortMultipartUpload(request);
}
@Override
public CompleteMultipartUploadResult completeMultipartUpload(CompleteMultipartUploadRequest request)
throws OSSException, ClientException {
return multipartOperation.completeMultipartUpload(request);
}
@Override
public InitiateMultipartUploadResult initiateMultipartUpload(InitiateMultipartUploadRequest request)
throws OSSException, ClientException {
return multipartOperation.initiateMultipartUpload(request);
}
@Override
public MultipartUploadListing listMultipartUploads(ListMultipartUploadsRequest request)
throws OSSException, ClientException {
return multipartOperation.listMultipartUploads(request);
}
@Override
public PartListing listParts(ListPartsRequest request) throws OSSException, ClientException {
return multipartOperation.listParts(request);
}
@Override
public UploadPartResult uploadPart(UploadPartRequest request) throws OSSException, ClientException {
return multipartOperation.uploadPart(request);
}
@Override
public UploadPartCopyResult uploadPartCopy(UploadPartCopyRequest request) throws OSSException, ClientException {
return multipartOperation.uploadPartCopy(request);
}
@Override
public VoidResult setBucketCORS(SetBucketCORSRequest request) throws OSSException, ClientException {
return corsOperation.setBucketCORS(request);
}
@Override
public List getBucketCORSRules(String bucketName) throws OSSException, ClientException {
return this.getBucketCORSRules(new GenericRequest(bucketName));
}
@Override
public List getBucketCORSRules(GenericRequest genericRequest) throws OSSException, ClientException {
return this.getBucketCORS(genericRequest).getCorsRules();
}
@Override
public CORSConfiguration getBucketCORS(GenericRequest genericRequest) throws OSSException, ClientException {
return corsOperation.getBucketCORS(genericRequest);
}
@Override
public VoidResult deleteBucketCORSRules(String bucketName) throws OSSException, ClientException {
return this.deleteBucketCORSRules(new GenericRequest(bucketName));
}
@Override
public VoidResult deleteBucketCORSRules(GenericRequest genericRequest) throws OSSException, ClientException {
return corsOperation.deleteBucketCORS(genericRequest);
}
@Override
public ResponseMessage optionsObject(OptionsRequest request) throws OSSException, ClientException {
return corsOperation.optionsObject(request);
}
@Override
public VoidResult setBucketLogging(SetBucketLoggingRequest request) throws OSSException, ClientException {
return bucketOperation.setBucketLogging(request);
}
@Override
public BucketLoggingResult getBucketLogging(String bucketName) throws OSSException, ClientException {
return this.getBucketLogging(new GenericRequest(bucketName));
}
@Override
public BucketLoggingResult getBucketLogging(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.getBucketLogging(genericRequest);
}
@Override
public VoidResult deleteBucketLogging(String bucketName) throws OSSException, ClientException {
return this.deleteBucketLogging(new GenericRequest(bucketName));
}
@Override
public VoidResult deleteBucketLogging(GenericRequest genericRequest) throws OSSException, ClientException {
return bucketOperation.deleteBucketLogging(genericRequest);
}
@Override
public VoidResult putBucketImage(PutBucketImageRequest request) throws OSSException, ClientException {
return bucketOperation.putBucketImage(request);
}
@Override
public GetBucketImageResult getBucketImage(String bucketName) throws OSSException, ClientException {
return bucketOperation.getBucketImage(bucketName, new GenericRequest());
}
@Override
public GetBucketImageResult getBucketImage(String bucketName, GenericRequest genericRequest)
throws OSSException, ClientException {
return bucketOperation.getBucketImage(bucketName, genericRequest);
}
@Override
public VoidResult deleteBucketImage(String bucketName) throws OSSException, ClientException {
return bucketOperation.deleteBucketImage(bucketName, new GenericRequest());
}
@Override
public VoidResult deleteBucketImage(String bucketName, GenericRequest genericRequest)
throws OSSException, ClientException {
return bucketOperation.deleteBucketImage(bucketName, genericRequest);
}
@Override
public VoidResult putImageStyle(PutImageStyleRequest putImageStyleRequest) throws OSSException, ClientException {
return bucketOperation.putImageStyle(putImageStyleRequest);
}
@Override
public VoidResult deleteImageStyle(String bucketName, String styleName) throws OSSException, ClientException {
return bucketOperation.deleteImageStyle(bucketName, styleName, new GenericRequest());
}
@Override
public VoidResult deleteImageStyle(String bucketName, String styleName, GenericRequest genericRequest)
throws OSSException, ClientException {
return bucketOperation.deleteImageStyle(bucketName, styleName, genericRequest);
}
@Override
public GetImageStyleResult getImageStyle(String bucketName, String styleName) throws OSSException, ClientException {
return bucketOperation.getImageStyle(bucketName, styleName, new GenericRequest());
}
@Override
public GetImageStyleResult getImageStyle(String bucketName, String styleName, GenericRequest genericRequest)
throws OSSException, ClientException {
return bucketOperation.getImageStyle(bucketName, styleName, genericRequest);
}
@Override
public List