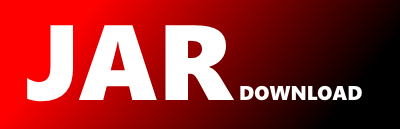
com.aliyun.oss.model.RoutingRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-sdk-oss-shade Show documentation
Show all versions of aliyun-sdk-oss-shade Show documentation
The Aliyun OSS SDK for Java used for accessing Aliyun Object Storage Service, includes all service and dependent JARs.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.aliyun.oss.model;
import com.aliyun.oss.ClientException;
import com.aliyun.oss.OSSErrorCode;
import java.text.MessageFormat;
import java.util.List;
import java.util.Map;
/**
* A rule that identifies a condition and the redirect that is applied when the
* condition is met.
*
*/
public class RoutingRule {
/**
* Container for describing a condition that must be met for the specified
* redirect to be applied. If the routing rule does not include a condition,
* the rule is applied to all requests.
*/
public static class Condition {
public String getKeyPrefixEquals() {
return keyPrefixEquals;
}
public void setKeyPrefixEquals(String keyPrefixEquals) {
this.keyPrefixEquals = keyPrefixEquals;
}
public Integer getHttpErrorCodeReturnedEquals() {
return httpErrorCodeReturnedEquals;
}
public void setHttpErrorCodeReturnedEquals(Integer httpErrorCodeReturnedEquals) {
if (httpErrorCodeReturnedEquals == null) {
return;
}
if (httpErrorCodeReturnedEquals <= 0) {
throw new IllegalArgumentException(MessageFormat.format("HttpErrorCodeReturnedEqualsInvalid",
"HttpErrorCodeReturnedEquals should be greater than 0"));
}
this.httpErrorCodeReturnedEquals = httpErrorCodeReturnedEquals;
}
public void ensureConditionValid() {
}
public String getKeySuffixEquals() {
return keySuffixEquals;
}
public void setKeySuffixEquals(String keySuffixEquals) {
this.keySuffixEquals = keySuffixEquals;
}
public List getIncludeHeaders() {
return includeHeaders;
}
public void setIncludeHeaders(List includeHeaders) {
this.includeHeaders = includeHeaders;
}
/**
* The object key name prefix from which requests will be redirected.
*/
private String keyPrefixEquals;
/**
* The object key name prefix from which requests will be redirected.
*/
private String keySuffixEquals;
/**
* The HTTP error code that must match for the redirect to apply. In the
* event of an error, if the error code meets this value, then specified
* redirect applies.
*/
private Integer httpErrorCodeReturnedEquals;
private List includeHeaders;
}
public static class IncludeHeader {
/**
* name of header
*/
private String key;
/**
* key should be equal to the given value
*/
private String equals;
/**
* key should be start with the given value
*/
private String startsWith;
/**
* key should be end with the given value
*/
private String endsWith;
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public String getEquals() {
return equals;
}
public void setEquals(String equals) {
this.equals = equals;
}
public String getStartsWith() {
return startsWith;
}
public void setStartsWith(String startsWith) {
this.startsWith = startsWith;
}
public String getEndsWith() {
return endsWith;
}
public void setEndsWith(String endsWith) {
this.endsWith = endsWith;
}
}
public static enum RedirectType {
/**
* Internal mode is not supported yet.
*/
Internal("Internal"),
/**
* 302 redirect.
*/
External("External"),
/**
* AliCDN
*/
AliCDN("AliCDN"),
/**
* Means OSS would read the source data on user's behalf and store it in
* OSS for later access.
*/
Mirror("Mirror");
private String redirectTypeString;
private RedirectType(String redirectTypeString) {
this.redirectTypeString = redirectTypeString;
}
@Override
public String toString() {
return this.redirectTypeString;
}
public static RedirectType parse(String redirectTypeString) {
for (RedirectType rt : RedirectType.values()) {
if (rt.toString().equals(redirectTypeString)) {
return rt;
}
}
throw new IllegalArgumentException("Unable to parse " + redirectTypeString);
}
}
public static enum Protocol {
Http("http"), Https("https");
private String protocolString;
private Protocol(String protocolString) {
this.protocolString = protocolString;
}
@Override
public String toString() {
return this.protocolString;
}
public static Protocol parse(String protocolString) {
for (Protocol protocol : Protocol.values()) {
if (protocol.toString().equals(protocolString)) {
return protocol;
}
}
throw new IllegalArgumentException("Unable to parse " + protocolString);
}
}
public static class MirrorHeaders{
/**
* Flags of passing all headers to source site.
*/
private boolean passAll;
/**
* Only headers include in list can be passed to source site.
*/
private List pass;
/**
* Headers include in list cannot be passed to source site.
*/
private List remove;
/**
* Define the value for some headers.
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy