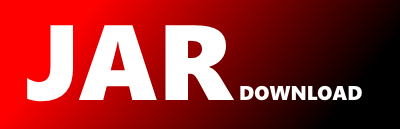
com.aliyun.oss.shade.io.opentelemetry.api.metrics.DoubleGaugeBuilder Maven / Gradle / Ivy
Show all versions of aliyun-sdk-oss-shade Show documentation
/*
* Copyright The OpenTelemetry Authors
* SPDX-License-Identifier: Apache-2.0
*/
package io.opentelemetry.api.metrics;
import java.util.function.Consumer;
/**
* A builder for Gauge metric types. These can only be asynchronously collected.
*
* @since 1.10.0
*/
public interface DoubleGaugeBuilder {
/**
* Sets the description for this instrument.
*
* @param description The description.
* @see Instrument
* Description
*/
DoubleGaugeBuilder setDescription(String description);
/**
* Sets the unit of measure for this instrument.
*
* @param unit The unit. Instrument units must be 63 or fewer ASCII characters.
* @see Instrument
* Unit
*/
DoubleGaugeBuilder setUnit(String unit);
/** Sets the Gauge for recording {@code long} values. */
LongGaugeBuilder ofLongs();
/**
* Builds an Asynchronous Gauge instrument with the given callback.
*
* The callback will be called when the instrument is being observed.
*
*
Callbacks are expected to abide by the following restrictions:
*
*
* - Run in a finite amount of time.
*
- Safe to call repeatedly, across multiple threads.
*
*
* @param callback A callback which observes measurements when invoked.
*/
ObservableDoubleGauge buildWithCallback(Consumer callback);
/**
* Build an observer for this instrument to observe values from a {@link BatchCallback}.
*
* This observer MUST be registered when creating a {@link Meter#batchCallback(Runnable,
* ObservableMeasurement, ObservableMeasurement...) batchCallback}, which records to it. Values
* observed outside registered callbacks are ignored.
*
* @return an observable measurement that batch callbacks use to observe values.
* @since 1.15.0
*/
default ObservableDoubleMeasurement buildObserver() {
return DefaultMeter.getInstance().gaugeBuilder("noop").buildObserver();
}
/**
* Builds and returns a DoubleGauge instrument with the configuration.
*
*
NOTE: This produces a synchronous gauge which records gauge values as they occur. Most users
* will want to instead register an {@link #buildWithCallback(Consumer)} to asynchronously observe
* the value of the gauge when metrics are collected.
*
*
If using the OpenTelemetry SDK, by default gauges use last value aggregation, such that only
* the value of the last recorded measurement is exported.
*
* @return The DoubleGauge instrument.
* @since 1.38.0
*/
default DoubleGauge build() {
return DefaultMeter.getInstance().gaugeBuilder("noop").build();
}
}