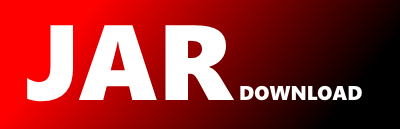
org.apache.phoenix.execute.SingleKeyQueryPlan Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.phoenix.execute;
import java.sql.ParameterMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.htrace.TraceScope;
import org.apache.phoenix.compile.*;
import org.apache.phoenix.expression.Expression;
import org.apache.phoenix.iterate.*;
import org.apache.phoenix.jdbc.PhoenixConnection;
import org.apache.phoenix.jdbc.PhoenixStatement;
import org.apache.phoenix.parse.FilterableStatement;
import org.apache.phoenix.query.KeyRange;
import org.apache.phoenix.query.QueryServices;
import org.apache.phoenix.query.QueryServicesOptions;
import org.apache.phoenix.schema.TableRef;
import org.apache.phoenix.trace.TracingIterator;
import org.apache.phoenix.trace.util.Tracing;
import org.apache.phoenix.util.LogUtil;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
public class SingleKeyQueryPlan implements QueryPlan {
private static final Log LOG = LogFactory.getLog(SingleKeyQueryPlan.class);
private final StatementContext context;
private final FilterableStatement statement;
private final TableRef table;
private final RowProjector projector;
private final Integer limit;
private final Integer offset;
private final Expression where;
private final OrderByCompiler.OrderBy orderBy;
private final PhoenixConnection connection;
public SingleKeyQueryPlan(StatementContext context, FilterableStatement statement, TableRef table,
RowProjector projector, Integer limit, Integer offset, Expression where, OrderByCompiler.OrderBy orderBy) {
this.context = context;
this.statement = statement;
this.table = table;
this.projector = projector;
this.limit = limit;
this.offset = offset;
this.where = where;
this.orderBy = orderBy;
this.connection = context.getConnection();
}
@Override
public StatementContext getContext() {
return context;
}
@Override
public ParameterMetaData getParameterMetaData() {
return context.getBindManager().getParameterMetaData();
}
@Override
public ExplainPlan getExplainPlan() throws SQLException {
List steps = new ArrayList<>();
ResultIterator iterator = iterator();
iterator.explain(steps);
return new ExplainPlan(steps);
}
@Override
public Set getSourceRefs() {
return ImmutableSet.of(table);
}
@Override
public PhoenixStatement.Operation getOperation() {
return PhoenixStatement.Operation.QUERY;
}
@Override
public Long getEstimatedRowsToScan() {
return null;
}
@Override
public Long getEstimatedBytesToScan() {
return null;
}
@Override
public Long getEstimateInfoTimestamp() {
return null;
}
@Override
public final ResultIterator iterator() throws SQLException {
return iterator(DefaultParallelScanGrouper.getInstance());
}
@Override
public final ResultIterator iterator(ParallelScanGrouper scanGrouper) throws SQLException {
return iterator(scanGrouper, null);
}
@Override
public final ResultIterator iterator(ParallelScanGrouper scanGrouper, Scan scan) throws SQLException {
if (LOG.isDebugEnabled()) {
LOG.debug(LogUtil.addCustomAnnotations("Gets ready for iteration: " + scan, connection));
}
ResultIterator iterator = new GettingResultIterator(context, where);
if (!orderBy.getOrderByExpressions().isEmpty()) { // TopN
int thresholdBytes = context.getConnection().getQueryServices().getProps().getInt(
QueryServices.SPOOL_THRESHOLD_BYTES_ATTRIB, QueryServicesOptions.DEFAULT_SPOOL_THRESHOLD_BYTES);
iterator = new OrderedResultIterator(iterator, orderBy.getOrderByExpressions(), thresholdBytes, limit,
offset, projector.getEstimatedRowByteSize());
} else {
if (offset != null) {
iterator = new OffsetResultIterator(iterator, offset);
}
if (limit != null) {
iterator = new LimitingResultIterator(iterator, limit);
}
}
if (context.getSequenceManager().getSequenceCount() > 0) {
iterator = new SequenceResultIterator(iterator, context.getSequenceManager());
}
if (LOG.isDebugEnabled()) {
LOG.debug(LogUtil.addCustomAnnotations("Iterator ready: " + iterator, connection));
}
TraceScope scope = Tracing.startNewSpan(context.getConnection(),
"Creating basic query for " + getPlanSteps(iterator));
return (scope.getSpan() != null) ? new TracingIterator(scope, iterator) : iterator;
}
private List getPlanSteps(ResultIterator iterator){
List planSteps = Lists.newArrayListWithExpectedSize(5);
iterator.explain(planSteps);
return planSteps;
}
@Override
public long getEstimatedSize() {
return 0;
}
@Override
public TableRef getTableRef() {
return table;
}
@Override
public RowProjector getProjector() {
return projector;
}
@Override
public Integer getLimit() {
return limit;
}
@Override
public Integer getOffset() {
return offset;
}
@Override
public OrderByCompiler.OrderBy getOrderBy() {
return orderBy;
}
@Override
public GroupByCompiler.GroupBy getGroupBy() {
return null;
}
@Override
public List getSplits() {
return Collections.EMPTY_LIST;
}
@Override
public List> getScans() {
return Collections.EMPTY_LIST;
}
@Override
public FilterableStatement getStatement() {
return statement;
}
@Override
public boolean isDegenerate() {
return false;
}
@Override
public boolean isRowKeyOrdered() {
return false;
}
@Override
public boolean useRoundRobinIterator() throws SQLException {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy