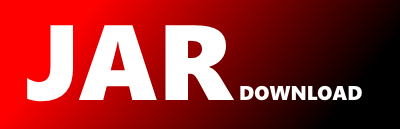
com.aliyun.tair.tairroaring.TairRoaringPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-tairjedis-sdk Show documentation
Show all versions of alibabacloud-tairjedis-sdk Show documentation
Aliyun Tair Redis client for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun.tair.tairroaring;
import com.aliyun.tair.ModuleCommand;
import com.aliyun.tair.util.JoinParameters;
import redis.clients.jedis.BuilderFactory;
import com.aliyun.tair.tairroaring.factory.RoaringBuilderFactory;
import redis.clients.jedis.Pipeline;
import redis.clients.jedis.Response;
import redis.clients.jedis.ScanResult;
import redis.clients.jedis.util.SafeEncoder;
import static redis.clients.jedis.Protocol.toByteArray;
import java.util.ArrayList;
import java.util.List;
public class TairRoaringPipeline extends Pipeline {
/**
* TR.SETBIT TR.SETBIT
* setting the value at the offset in roaringbitmap
*
* @param key roaring key
* @param offset the bit offset
* @param value the bit value
* @return Success: long; Fail: error
*/
public Response trsetbit(final String key, long offset, final String value) {
getClient("").sendCommand(ModuleCommand.TRSETBIT, SafeEncoder.encode(key), toByteArray(offset), SafeEncoder.encode(value));
return getResponse(BuilderFactory.LONG);
}
public Response trsetbit(final String key, long offset, long value) {
getClient("").sendCommand(ModuleCommand.TRSETBIT, SafeEncoder.encode(key), toByteArray(offset), toByteArray(value));
return getResponse(BuilderFactory.LONG);
}
public Response trsetbit(byte[] key, long offset, byte[] value) {
getClient("").sendCommand(ModuleCommand.TRSETBIT, key, toByteArray(offset), value);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.SETBITS TR.SETBITS [ ... ]
* setting the value at the offset in roaringbitmap
*
* @param fields the bit offset
* @param key roaring key
* @return Success: long; Fail: error
*/
public Response trsetbits(final String key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRSETBITS,
JoinParameters.joinParameters(SafeEncoder.encode(key), args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.LONG);
}
public Response trsetbits(byte[] key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRSETBITS,
JoinParameters.joinParameters(key, args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.GETBIT TR.GETBIT
* getting the bit on the offset of roaringbitmap
*
* @param key roaring key
* @param offset the bit offset
* @return Success: long; Fail: error
*/
public Response trgetbit(final String key, long offset) {
getClient("").sendCommand(ModuleCommand.TRGETBIT, SafeEncoder.encode(key), toByteArray(offset));
return getResponse(BuilderFactory.LONG);
}
public Response trgetbit(byte[] key, long offset) {
getClient("").sendCommand(ModuleCommand.TRGETBIT, key, toByteArray(offset));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.GETBITS TR.GETBITS [ ... ]
* get the value at the offset in roaringbitmap
*
* @param key roaring key
* @param fields the bit offset
* @return Success: array long; Fail: error
*/
public Response> trgetbits(final String key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRGETBITS,
JoinParameters.joinParameters(SafeEncoder.encode(key), args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.LONG_LIST);
}
public Response> trgetbits(byte[] key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRGETBITS,
JoinParameters.joinParameters(key, args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.LONG_LIST);
}
/**
* TR.CLEARBITS TR.CLEARBITS [ ... ]
* remove the value at the offset in roaringbitmap
*
* @param key roaring key
* @param fields the bit offset
* @return Success: long; Fail: error
*/
public Response trclearbits(final String key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRCLEARBITS,
JoinParameters.joinParameters(SafeEncoder.encode(key), args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.LONG);
}
public Response trclearbits(byte[] key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRCLEARBITS,
JoinParameters.joinParameters(key, args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.RANGE TR.RANGE
* retrive the setted bit between the closed range, return them with int array
*
* @param key roaring bitmap key
* @param start range start
* @param end range end
* @return Success: array long; Fail: error
*/
public Response> trrange(final String key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRRANGE, SafeEncoder.encode(key), toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG_LIST);
}
public Response> trrange(byte[] key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRRANGE, key, toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG_LIST);
}
/**
* TR.RANGEBITARRAY TR.RANGEBITARRAY
* retrive the setted bit between the closed range, return them by the string bit-array
*
* @param key roaring bitmap key
* @param start range start
* @param end range end
* @return Success: string; Fail: error
*/
public Response trrangebitarray(final String key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRRANGEBITARRAY, SafeEncoder.encode(key), toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.STRING);
}
public Response trrangebitarray(byte[] key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRRANGEBITARRAY, key, toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.STRING);
}
/**
* TR.APPENDBITARRAY TR.APPENDBITARRAY
* append the bit array after the offset in roaringbitmap
*
* @param key roaring key
* @param offset the bit offset
* @param value the bit value
* @return Success: long; Fail: error
*/
public Response trappendbitarray(final String key, long offset, final String value) {
getClient("").sendCommand(ModuleCommand.TRAPPENDBITARRAY, SafeEncoder.encode(key), toByteArray(offset), SafeEncoder.encode(value));
return getResponse(BuilderFactory.LONG);
}
public Response trappendbitarray(final String key, long offset, byte[] value) {
getClient("").sendCommand(ModuleCommand.TRAPPENDBITARRAY, SafeEncoder.encode(key), toByteArray(offset), value);
return getResponse(BuilderFactory.LONG);
}
public Response trappendbitarray(byte[] key, long offset, byte[] value) {
getClient("").sendCommand(ModuleCommand.TRAPPENDBITARRAY, key, toByteArray(offset), value);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.SETRANGE TR.SETRANGE
* set all the elements between min (included) and max (included).
*
* @param key roaring bitmap key
* @param start range start
* @param end range end
* @return Success: long; Fail: error
*/
public Response trsetrange(final String key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRSETRANGE, SafeEncoder.encode(key), toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG);
}
public Response trsetrange(byte[] key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRSETRANGE, key, toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.FLIPRANGE TR.FLIPRANGE
* flip all elements in the roaring bitmap within a specified interval: [range_start, range_end].
*
* @param key roaring bitmap key
* @param start range start
* @param end range end
* @return Success: array long; Fail: error
*/
public Response trfliprange(final String key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRFLIPRANGE, SafeEncoder.encode(key), toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG);
}
public Response trfliprange(byte[] key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRFLIPRANGE, key, toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.BITCOUNT TR.BITCOUNT [ ]
* counting bit set as 1 in the roaringbitmap
* start and end are optional, you can count 1-bit in range by passing start and end
*
* @param key roaring key
* @param start range start
* @param end range end
* @return Success: long; Fail: error
*/
public Response trbitcount(final String key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRBITCOUNT, SafeEncoder.encode(key), toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG);
}
public Response trbitcount(final String key) {
getClient("").sendCommand(ModuleCommand.TRBITCOUNT, SafeEncoder.encode(key));
return getResponse(BuilderFactory.LONG);
}
public Response trbitcount(byte[] key) {
getClient("").sendCommand(ModuleCommand.TRBITCOUNT, key);
return getResponse(BuilderFactory.LONG);
}
public Response trbitcount(byte[] key, long start, long end) {
getClient("").sendCommand(ModuleCommand.TRBITCOUNT, key, toByteArray(start), toByteArray(end));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.MIN TR.MIN
* return the minimum element's offset set in the roaring bitmap
*
* @param key roaring key
* @return Success: long; Fail: error
*/
public Response trmin(final String key) {
getClient("").sendCommand(ModuleCommand.TRMIN, SafeEncoder.encode(key));
return getResponse(BuilderFactory.LONG);
}
public Response trmin(byte[] key) {
getClient("").sendCommand(ModuleCommand.TRMIN, key);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.MAX TR.MAX
* return the maximum element's offset set in the roaring bitmap
*
* @param key roaring key
* @return Success: long; Fail: error
*/
public Response trmax(final String key) {
getClient("").sendCommand(ModuleCommand.TRMAX, SafeEncoder.encode(key));
return getResponse(BuilderFactory.LONG);
}
public Response trmax(byte[] key) {
getClient("").sendCommand(ModuleCommand.TRMAX, key);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.OPTIMIZE TR.OPTIMIZE
* optimize memory usage by trying to use RLE container instead of int array or bitset.
* it will also run shrink_to_fit on bitmap, this may cause memory reallocation.
* optimize will try this function but did not make a guarantee that any change would happen
*
* @param key roaring key
* @return Success: +OK; Fail: error
*/
public Response troptimize(final String key) {
getClient("").sendCommand(ModuleCommand.TROPTIMIZE, SafeEncoder.encode(key));
return getResponse(BuilderFactory.STRING);
}
public Response troptimize(byte[] key) {
getClient("").sendCommand(ModuleCommand.TROPTIMIZE, key);
return getResponse(BuilderFactory.STRING);
}
/**
* TR.STAT TR.STAT
* return roaring bitmap statistic information, you can get JSON formatted result by passing json = true.
*
* @param key roaring key
* @return Success: string; Fail: error
*/
public Response trstat(final String key, boolean json) {
if (json = true) {
getClient("").sendCommand(ModuleCommand.TRSTAT, SafeEncoder.encode(key), SafeEncoder.encode("JSON"));
return getResponse(BuilderFactory.STRING);
}
getClient("").sendCommand(ModuleCommand.TRSTAT, SafeEncoder.encode(key));
return getResponse(BuilderFactory.STRING);
}
public Response trstat(byte[] key, boolean json) {
if (json == true) {
getClient("").sendCommand(ModuleCommand.TRSTAT, key, SafeEncoder.encode("JSON"));
return getResponse(BuilderFactory.STRING);
}
getClient("").sendCommand(ModuleCommand.TRSTAT, key);
return getResponse(BuilderFactory.STRING);
}
/**
* TR.BITPOS TR.BITPOS [counting]
* return the first element set as value at index, where the smallest element is at index 0.
* counting is an optional argument, you can pass positive Counting to indicate the command count for the n-th element from the top
* or pass an negative Counting to count from the n-th element form the bottom.
*
* @param key roaring key
* @param value bit value
* @param count count of the bit, negetive count indecate the reverse iteration
* @return Success: long; Fail: error
*/
public Response trbitpos(final String key, final String value, long count) {
getClient("").sendCommand(ModuleCommand.TRBITPOS, SafeEncoder.encode(key), SafeEncoder.encode(value), toByteArray(count));
return getResponse(BuilderFactory.LONG);
}
public Response trbitpos(final String key, final String value) {
getClient("").sendCommand(ModuleCommand.TRBITPOS, SafeEncoder.encode(key), SafeEncoder.encode(value));
return getResponse(BuilderFactory.LONG);
}
public Response trbitpos(final String key, long value) {
getClient("").sendCommand(ModuleCommand.TRBITPOS, SafeEncoder.encode(key), toByteArray(value));
return getResponse(BuilderFactory.LONG);
}
public Response trbitpos(final String key, long value, long count) {
getClient("").sendCommand(ModuleCommand.TRBITPOS, SafeEncoder.encode(key), toByteArray(value), toByteArray(count));
return getResponse(BuilderFactory.LONG);
}
public Response trbitpos(byte[] key, byte[] value) {
getClient("").sendCommand(ModuleCommand.TRBITPOS, key, value);
return getResponse(BuilderFactory.LONG);
}
public Response trbitpos(byte[] key, byte[] value, byte[] count) {
getClient("").sendCommand(ModuleCommand.TRBITPOS, key, value, count);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.RANK TR.RANK
* rank returns the number of elements that are smaller or equal to offset.
*
* @param key roaring key
* @param offset bit ranking offset
* @return Success: long; Fail: error
*/
public Response trrank(final String key, long offset) {
getClient("").sendCommand(ModuleCommand.TRRANK, SafeEncoder.encode(key), toByteArray(offset));
return getResponse(BuilderFactory.LONG);
}
public Response trrank(byte[] key, byte[] offset) {
getClient("").sendCommand(ModuleCommand.TRRANK, key, offset);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.BITOP TR.BITOP [ ...]
* call bitset computation on given roaring bitmaps, store the result into destkey
* return the cardinality of result.
* operation can be passed to AND OR XOR NOT DIFF.
*
* @param destkey result store int destkey
* @param operation operation type: AND OR NOT XOR DIFF
* @param keys operation joining keys
* @return Success: long; Fail: error
*/
public Response trbitop(final String destkey, final String operation, final String... keys) {
getClient("").sendCommand(ModuleCommand.TRBITOP,
JoinParameters.joinParameters(SafeEncoder.encode(destkey), SafeEncoder.encode(operation), SafeEncoder.encodeMany(keys)));
return getResponse(BuilderFactory.LONG);
}
public Response trbitop(byte[] destkey, byte[] operation, byte[]... keys) {
getClient("").sendCommand(ModuleCommand.TRBITOP, JoinParameters.joinParameters(destkey, operation, keys));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.BITOPCARD TR.BITOPCARD [ ...]
* call bitset computation on given roaring bitmaps, return the cardinality of result.
* operation can be passed to AND OR XOR NOT DIFF.
*
* @param operation operation type: AND OR NOT XOR DIFF
* @param keys operation joining keys
* @return Success: long; Fail: error
*/
public Response trbitopcard(final String operation , final String... keys) {
getClient("").sendCommand(ModuleCommand.TRBITOPCARD,
JoinParameters.joinParameters(SafeEncoder.encode(operation), SafeEncoder.encodeMany(keys)));
return getResponse(BuilderFactory.LONG);
}
public Response trbitopcard(byte[] operation, byte[]... keys) {
getClient("").sendCommand(ModuleCommand.TRBITOPCARD, JoinParameters.joinParameters(operation, keys));
return getResponse(BuilderFactory.LONG);
}
/**
* TR.SCAN TR.SCAN [COUNT ]
* iterating element from cursor, COUNT indecate the max elements count per request
*
* @param key roaring bitmap key
* @param cursor scan cursor, 0 stand for the very first value
* @param count iteration counting by scan
* @return Success: cursor and array long; Fail: error
*/
public Response> trscan(final String key, long cursor, long count) {
getClient("").sendCommand(ModuleCommand.TRSCAN, SafeEncoder.encode(key), toByteArray(cursor), SafeEncoder.encode("COUNT"), toByteArray(count));
return getResponse(RoaringBuilderFactory.TRSCAN_RESULT_LONG);
}
public Response> trscan(final String key, long cursor) {
getClient("").sendCommand(ModuleCommand.TRSCAN, SafeEncoder.encode(key), toByteArray(cursor));
return getResponse(RoaringBuilderFactory.TRSCAN_RESULT_LONG);
}
public Response> trscan(byte[] key, byte[] cursor) {
getClient("").sendCommand(ModuleCommand.TRSCAN, key, cursor);
return getResponse(RoaringBuilderFactory.TRSCAN_RESULT_BYTE);
}
public Response> trscan(byte[] key, byte[] cursor, byte[] count) {
getClient("").sendCommand(ModuleCommand.TRSCAN, key, cursor, SafeEncoder.encode("COUNT"), count);
return getResponse(RoaringBuilderFactory.TRSCAN_RESULT_BYTE);
}
/**
* TR.LOADSTRING TR.LOAD
* Loading string into into a empty roaringbitmap
*
* @param key result store int key
* @param stringkey string, aka origional bitmap
* @return Success: long; Fail: error
*/
public Response trloadstring(final String key, final String stringkey) {
getClient("").sendCommand(ModuleCommand.TRLOADSTRING, SafeEncoder.encode(key), SafeEncoder.encode(stringkey));
return getResponse(BuilderFactory.LONG);
}
public Response trloadstring(byte[] key, byte[] stringkey) {
getClient("").sendCommand(ModuleCommand.TRLOADSTRING, key, stringkey);
return getResponse(BuilderFactory.LONG);
}
/**
* TR.DIFF TR.DIFF
* caculate the difference (andnot) by key1 and key2, store the result into destkey
*
* @param destkey result store int key
* @param key1 operation diff key
* @param key2 operation diff key
* @return Success: OK; Fail: error
*/
public Response trdiff(final String destkey, final String key1, final String key2) {
getClient("").sendCommand(ModuleCommand.TRDIFF, SafeEncoder.encode(destkey),
SafeEncoder.encode(key1), SafeEncoder.encode(key2));
return getResponse(BuilderFactory.STRING);
}
public Response trdiff(byte[] destkey, byte[] key1, byte[] key2) {
getClient("").sendCommand(ModuleCommand.TRDIFF, destkey, key1, key2);
return getResponse(BuilderFactory.STRING);
}
/**
* TR.SETINTARRAY TR.SETINTARRAY [ ... ]
* reset the bitmap by given integer array.
*
* @param key roaring bitmap key
* @param fields bit offset value
* @return Success: +OK; Fail: error
*/
public Response trsetintarray(final String key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRSETINTARRAY,
JoinParameters.joinParameters(SafeEncoder.encode(key), args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.STRING);
}
public Response trsetintarray(byte[] key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRSETINTARRAY,
JoinParameters.joinParameters(key, args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.STRING);
}
/**
* TR.APPENDINTARRAY TR.APPENDINTARRAY [ ... ]
* add elements to the roaring bitmap.
*
* @param key roaring bitmap key
* @param fields bit offset value
* @return Success: +OK; Fail: error
*/
public Response trappendintarray(final String key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRAPPENDINTARRAY,
JoinParameters.joinParameters(SafeEncoder.encode(key), args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.STRING);
}
public Response trappendintarray(byte[] key, long... fields) {
final List args = new ArrayList();
for (long value : fields) {
args.add(toByteArray(value));
}
getClient("").sendCommand(ModuleCommand.TRAPPENDINTARRAY,
JoinParameters.joinParameters(key, args.toArray(new byte[args.size()][])));
return getResponse(BuilderFactory.STRING);
}
/**
* TR.SETBITARRAY TR.SETBITARRAY
* reset the roaring bitmap by given 01-bit string bitset.
*
* @param key roaring bitmap key
* @param value bit offset value
* @return Success: +OK; Fail: error
*/
public Response trsetbitarray(final String key, final String value) {
getClient("").sendCommand(ModuleCommand.TRSETBITARRAY, SafeEncoder.encode(key), SafeEncoder.encode(value));
return getResponse(BuilderFactory.STRING);
}
public Response trsetbitarray(byte[] key, byte[] value) {
getClient("").sendCommand(ModuleCommand.TRSETBITARRAY, key, value);
return getResponse(BuilderFactory.STRING);
}
/**
* TR.JACCARD TR.JACCARD
* caculate roaringbitmap Jaccard index on key1 and key2.
*
* @param key1 operation key
* @param key2 operation key
* @return Success: double; Fail: error
*/
public Response trjaccard(final String key1, final String key2) {
getClient("").sendCommand(ModuleCommand.TRJACCARD, SafeEncoder.encode(key1), SafeEncoder.encode(key2));
return getResponse(BuilderFactory.DOUBLE);
}
public Response trjaccard(byte[] key1, byte[] key2) {
getClient("").sendCommand(ModuleCommand.TRJACCARD, key1, key2);
return getResponse(BuilderFactory.DOUBLE);
}
/**
* TR.CONTAINS TR.CONTAINS
* return wether roaring bitmap key1 is a sub-set of key2
*
* @param key1 operation key
* @param key2 operation key
* @return Success: double; Fail: error
*/
public Response trcontains(final String key1, final String key2) {
getClient("").sendCommand(ModuleCommand.TRCONTAINS, SafeEncoder.encode(key1), SafeEncoder.encode(key2));
return getResponse(BuilderFactory.BOOLEAN);
}
public Response trcontains(byte[] key1, byte[] key2) {
getClient("").sendCommand(ModuleCommand.TRCONTAINS, key1, key2);
return getResponse(BuilderFactory.BOOLEAN);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy