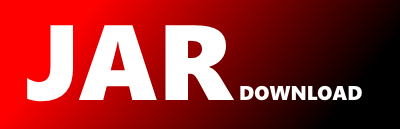
com.aliyun.tair.tairsearch.TairSearchPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-tairjedis-sdk Show documentation
Show all versions of alibabacloud-tairjedis-sdk Show documentation
Aliyun Tair Redis client for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun.tair.tairsearch;
import java.util.Map;
import com.aliyun.tair.ModuleCommand;
import com.aliyun.tair.tairsearch.params.TFTAddDocParams;
import com.aliyun.tair.tairsearch.params.TFTDelDocParams;
import com.aliyun.tair.util.JoinParameters;
import redis.clients.jedis.BuilderFactory;
import redis.clients.jedis.Pipeline;
import redis.clients.jedis.Response;
import redis.clients.jedis.util.SafeEncoder;
import static redis.clients.jedis.Protocol.toByteArray;
public class TairSearchPipeline extends Pipeline {
public Response tftmappingindex(String key, String request) {
return tftmappingindex(SafeEncoder.encode(key), SafeEncoder.encode(request));
}
public Response tftmappingindex(byte[] key, byte[] request) {
getClient("").sendCommand(ModuleCommand.TFTMAPPINGINDEX, key, request);
return getResponse(BuilderFactory.STRING);
}
public Response tftcreateindex(String key, String request) {
return tftcreateindex(SafeEncoder.encode(key), SafeEncoder.encode(request));
}
public Response tftcreateindex(byte[] key, byte[] request) {
getClient("").sendCommand(ModuleCommand.TFTCREATEINDEX, key, request);
return getResponse(BuilderFactory.STRING);
}
public Response tftupdateindex(String index, String request) {
return tftupdateindex(SafeEncoder.encode(index), SafeEncoder.encode(request));
}
public Response tftupdateindex(byte[] index, byte[] request) {
getClient("").sendCommand(ModuleCommand.TFTUPDATEINDEX, index, request);
return getResponse(BuilderFactory.STRING);
}
public Response tftgetindexmappings(String key) {
return tftgetindexmappings(SafeEncoder.encode(key));
}
public Response tftgetindexmappings(byte[] key) {
getClient("").sendCommand(ModuleCommand.TFTGETINDEX, key, SafeEncoder.encode("mappings"));
return getResponse(BuilderFactory.STRING);
}
public Response tftadddoc(String key, String request) {
return tftadddoc(SafeEncoder.encode(key), SafeEncoder.encode(request));
}
public Response tftadddoc(byte[] key, byte[] request) {
getClient("").sendCommand(ModuleCommand.TFTADDDOC, key, request);
return getResponse(BuilderFactory.STRING);
}
public Response tftadddoc(String key, String request, String docId) {
return tftadddoc(SafeEncoder.encode(key), SafeEncoder.encode(request), SafeEncoder.encode(docId));
}
public Response tftadddoc(byte[] key, byte[] request, byte[] docId) {
getClient("").sendCommand(ModuleCommand.TFTADDDOC, key, request, SafeEncoder.encode("WITH_ID"), docId);
return getResponse(BuilderFactory.STRING);
}
public Response tftmadddoc(String key, Map docs) {
TFTAddDocParams params = new TFTAddDocParams();
getClient("").sendCommand(ModuleCommand.TFTMADDDOC, params.getByteParams(key, docs));
return getResponse(BuilderFactory.STRING);
}
public Response tftmadddoc(byte[] key, Map docs) {
TFTAddDocParams params = new TFTAddDocParams();
getClient("").sendCommand(ModuleCommand.TFTMADDDOC, params.getByteParams(key, docs));
return getResponse(BuilderFactory.STRING);
}
@Deprecated
public Response tftupdatedoc(String index, String docId, String docContent) {
return tftupdatedoc(SafeEncoder.encode(index), SafeEncoder.encode(docId), SafeEncoder.encode(docContent));
}
@Deprecated
public Response tftupdatedoc(byte[] index, byte[] docId, byte[] docContent) {
getClient("").sendCommand(ModuleCommand.TFTUPDATEDOC, index, docId, docContent);
return getResponse(BuilderFactory.STRING);
}
public Response tftupdatedocfield(String index, String docId, String docContent) {
return tftupdatedoc(SafeEncoder.encode(index), SafeEncoder.encode(docId), SafeEncoder.encode(docContent));
}
public Response tftupdatedocfield(byte[] index, byte[] docId, byte[] docContent) {
getClient("").sendCommand(ModuleCommand.TFTUPDATEDOCFIELD, index, docId, docContent);
return getResponse(BuilderFactory.STRING);
}
public Response tftincrlongdocfield(String index, String docId, final String field, final long value) {
return tftincrlongdocfield(SafeEncoder.encode(index), SafeEncoder.encode(docId), SafeEncoder.encode(field), value);
}
public Response tftincrlongdocfield(byte[] index, byte[] docId, byte[] field, long value) {
getClient("").sendCommand(ModuleCommand.TFTINCRLONGDOCFIELD, index, docId, field, toByteArray(value));
return getResponse(BuilderFactory.LONG);
}
public Response tftincrfloatdocfield(String index, String docId, final String field, final double value) {
return tftincrfloatdocfield(SafeEncoder.encode(index), SafeEncoder.encode(docId), SafeEncoder.encode(field), value);
}
public Response tftincrfloatdocfield(byte[] index, byte[] docId, byte[] field, double value) {
getClient("").sendCommand(ModuleCommand.TFTINCRFLOATDOCFIELD, index, docId, field, toByteArray(value));
return getResponse(BuilderFactory.DOUBLE);
}
public Response tftdeldocfield(String index, String docId, final String... field) {
return tftdeldocfield(SafeEncoder.encode(index), SafeEncoder.encode(docId), SafeEncoder.encodeMany(field));
}
public Response tftdeldocfield(byte[] index, byte[] docId, byte[]... field) {
getClient("").sendCommand(ModuleCommand.TFTDELDOCFIELD, JoinParameters.joinParameters(index, docId, field));
return getResponse(BuilderFactory.LONG);
}
public Response tftgetdoc(String key, String docId) {
return tftgetdoc(SafeEncoder.encode(key), SafeEncoder.encode(docId));
}
public Response tftgetdoc(byte[] key, byte[] docId) {
getClient("").sendCommand(ModuleCommand.TFTGETDOC, key, docId);
return getResponse(BuilderFactory.STRING);
}
public Response tftgetdoc(String key, String docId, String request) {
return tftgetdoc(SafeEncoder.encode(key), SafeEncoder.encode(docId), SafeEncoder.encode(request));
}
public Response tftgetdoc(byte[] key, byte[] docId, byte[] request) {
getClient("").sendCommand(ModuleCommand.TFTGETDOC, key, docId, request);
return getResponse(BuilderFactory.STRING);
}
public Response tftdeldoc(String key, String... docId) {
TFTDelDocParams params = new TFTDelDocParams();
getClient("").sendCommand(ModuleCommand.TFTDELDOC, params.getByteParams(key, docId));
return getResponse(BuilderFactory.STRING);
}
public Response tftdeldoc(byte[] key, byte[]... docId) {
TFTDelDocParams params = new TFTDelDocParams();
getClient("").sendCommand(ModuleCommand.TFTDELDOC, params.getByteParams(key, docId));
return getResponse(BuilderFactory.STRING);
}
public Response tftdelall(String index) {
return tftdelall(SafeEncoder.encode(index));
}
public Response tftdelall(byte[] index) {
getClient("").sendCommand(ModuleCommand.TFTDELALL, index);
return getResponse(BuilderFactory.STRING);
}
public Response tftsearch(String key, String request) {
return tftsearch(SafeEncoder.encode(key), SafeEncoder.encode(request));
}
public Response tftsearch(byte[] key, byte[] request) {
getClient("").sendCommand(ModuleCommand.TFTSEARCH, key, request);
return getResponse(BuilderFactory.STRING);
}
public Response tftsearch(String key, String request, boolean use_cache) {
return tftsearch(SafeEncoder.encode(key), SafeEncoder.encode(request), use_cache);
}
public Response tftsearch(byte[] key, byte[] request, boolean use_cache) {
if (use_cache) {
getClient("").sendCommand(ModuleCommand.TFTSEARCH, key, request, SafeEncoder.encode("use_cache"));
} else {
getClient("").sendCommand(ModuleCommand.TFTSEARCH, key, request);
}
return getResponse(BuilderFactory.STRING);
}
public Response tftexists(String index, String docId) {
return tftexists(SafeEncoder.encode(index), SafeEncoder.encode(docId));
}
public Response tftexists(byte[] index, byte[] docId) {
getClient("").sendCommand(ModuleCommand.TFTEXISTS, index, docId);
return getResponse(BuilderFactory.LONG);
}
public Response tftdocnum(String index) {
return tftdocnum(SafeEncoder.encode(index));
}
public Response tftdocnum(byte[] index) {
getClient("").sendCommand(ModuleCommand.TFTDOCNUM, index);
return getResponse(BuilderFactory.LONG);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy