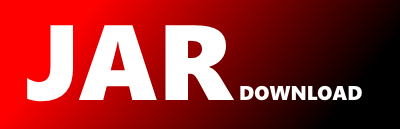
com.aliyun.tair.tairstring.TairStringPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-tairjedis-sdk Show documentation
Show all versions of alibabacloud-tairjedis-sdk Show documentation
Aliyun Tair Redis client for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
package com.aliyun.tair.tairstring;
import com.aliyun.tair.ModuleCommand;
import com.aliyun.tair.tairstring.params.CasParams;
import com.aliyun.tair.tairstring.params.ExincrbyFloatParams;
import com.aliyun.tair.tairstring.params.ExincrbyParams;
import com.aliyun.tair.tairstring.params.ExsetParams;
import com.aliyun.tair.tairstring.results.ExcasResult;
import com.aliyun.tair.tairstring.results.ExgetResult;
import com.aliyun.tair.tairstring.factory.StringBuilderFactory;
import com.aliyun.tair.tairstring.results.ExincrbyVersionResult;
import redis.clients.jedis.BuilderFactory;
import redis.clients.jedis.Pipeline;
import redis.clients.jedis.Response;
import redis.clients.jedis.util.SafeEncoder;
import static redis.clients.jedis.Protocol.toByteArray;
public class TairStringPipeline extends Pipeline {
public Response cas(String key, String oldvalue, String newvalue) {
return cas(SafeEncoder.encode(key), SafeEncoder.encode(oldvalue), SafeEncoder.encode(newvalue));
}
public Response cas(byte[] key, byte[] oldvalue, byte[] newvalue) {
getClient("").sendCommand(ModuleCommand.CAS, key, oldvalue, newvalue);
return getResponse(BuilderFactory.LONG);
}
public Response cas(String key, String oldvalue, String newvalue, CasParams params) {
return cas(SafeEncoder.encode(key), SafeEncoder.encode(oldvalue), SafeEncoder.encode(newvalue), params);
}
public Response cas(byte[] key, byte[] oldvalue, byte[] newvalue, CasParams params) {
getClient("").sendCommand(ModuleCommand.CAS, params.getByteParams(key, oldvalue, newvalue));
return getResponse(BuilderFactory.LONG);
}
public Response cad(String key, String value) {
return cad(SafeEncoder.encode(key), SafeEncoder.encode(value));
}
public Response cad(byte[] key, byte[] value) {
getClient("").sendCommand(ModuleCommand.CAD, key, value);
return getResponse(BuilderFactory.LONG);
}
public Response exset(String key, String value) {
getClient("").sendCommand(ModuleCommand.EXSET, key, value);
return getResponse(BuilderFactory.STRING);
}
public Response exset(byte[] key, byte[] value) {
getClient("").sendCommand(ModuleCommand.EXSET, key, value);
return getResponse(BuilderFactory.STRING);
}
public Response exset(String key, String value, ExsetParams params) {
getClient("").sendCommand(ModuleCommand.EXSET, params.getByteParams(key, value));
return getResponse(BuilderFactory.STRING);
}
public Response exset(byte[] key, byte[] value, ExsetParams params) {
getClient("").sendCommand(ModuleCommand.EXSET, params.getByteParams(key, value));
return getResponse(BuilderFactory.STRING);
}
public Response exsetVersion(String key, String value, ExsetParams params) {
getClient("").sendCommand(ModuleCommand.EXSET,
params.getByteParams(key, value, "WITHVERSION"));
return getResponse(BuilderFactory.LONG);
}
public Response exsetVersion(byte[] key, byte[] value, ExsetParams params) {
getClient("").sendCommand(ModuleCommand.EXSET,
params.getByteParams(key, value, "WITHVERSION".getBytes()));
return getResponse(BuilderFactory.LONG);
}
public Response> exget(String key) {
getClient("").sendCommand(ModuleCommand.EXGET, key);
return getResponse(StringBuilderFactory.EXGET_RESULT_STRING);
}
public Response> exget(byte[] key) {
getClient("").sendCommand(ModuleCommand.EXGET, key);
return getResponse(StringBuilderFactory.EXGET_RESULT_BYTE);
}
public Response> exgetFlags(String key) {
getClient("").sendCommand(ModuleCommand.EXGET, key, "WITHFLAGS");
return getResponse(StringBuilderFactory.EXGET_RESULT_STRING);
}
public Response> exgetFlags(byte[] key) {
getClient("").sendCommand(ModuleCommand.EXGET, key, "WITHFLAGS".getBytes());
return getResponse(StringBuilderFactory.EXGET_RESULT_BYTE);
}
public Response exsetver(String key, long version) {
return exsetver(SafeEncoder.encode(key), version);
}
public Response exsetver(byte[] key, long version) {
getClient("").sendCommand(ModuleCommand.EXSETVER, key, toByteArray(version));
return getResponse(BuilderFactory.LONG);
}
public Response exincrBy(String key, long incr) {
return exincrBy(SafeEncoder.encode(key), incr);
}
public Response exincrBy(byte[] key, long incr) {
getClient("").sendCommand(ModuleCommand.EXINCRBY, key, toByteArray(incr));
return getResponse(BuilderFactory.LONG);
}
public Response exincrBy(String key, long incr, ExincrbyParams params) {
return exincrBy(SafeEncoder.encode(key), incr, params);
}
public Response exincrBy(byte[] key, long incr, ExincrbyParams params) {
getClient("").sendCommand(ModuleCommand.EXINCRBY, params.getByteParams(key, toByteArray(incr)));
return getResponse(BuilderFactory.LONG);
}
public Response exincrByVersion(String key, long incr, ExincrbyParams params) {
return exincrByVersion(SafeEncoder.encode(key), incr, params);
}
public Response exincrByVersion(byte[] key, long incr, ExincrbyParams params) {
getClient("").sendCommand(ModuleCommand.EXINCRBY,
params.getByteParams(key, toByteArray(incr), "WITHVERSION".getBytes()));
return getResponse(StringBuilderFactory.EXINCRBY_VERSION_RESULT_STRING);
}
public Response exincrByFloat(String key, Double incr) {
return exincrByFloat(SafeEncoder.encode(key), incr);
}
public Response exincrByFloat(byte[] key, Double incr) {
getClient("").sendCommand(ModuleCommand.EXINCRBYFLOAT, key, toByteArray(incr));
return getResponse(BuilderFactory.DOUBLE);
}
public Response exincrByFloat(String key, Double incr, ExincrbyFloatParams params) {
return exincrByFloat(SafeEncoder.encode(key), incr, params);
}
public Response exincrByFloat(byte[] key, Double incr, ExincrbyFloatParams params) {
getClient("").sendCommand(ModuleCommand.EXINCRBYFLOAT, params.getByteParams(key, toByteArray(incr)));
return getResponse(BuilderFactory.DOUBLE);
}
public Response> excas(String key, String value, long version) {
getClient("").sendCommand(ModuleCommand.EXCAS, key, value, String.valueOf(version));
return getResponse(StringBuilderFactory.EXCAS_RESULT_STRING);
}
public Response> excas(byte[] key, byte[] value, long version) {
getClient("").sendCommand(ModuleCommand.EXCAS, key, value, toByteArray(version));
return getResponse(StringBuilderFactory.EXCAS_RESULT_BYTE);
}
public Response excad(String key, long version) {
return excad(SafeEncoder.encode(key), version);
}
public Response excad(byte[] key, long version) {
getClient("").sendCommand(ModuleCommand.EXCAD, key, toByteArray(version));
return getResponse(BuilderFactory.LONG);
}
public Response exappend(String key, String value, String nxxx, String verabs, long version) {
return exappend(SafeEncoder.encode(key), SafeEncoder.encode(value), nxxx, verabs, version);
}
public Response exappend(byte[] key, byte[] value, String nxxx, String verabs, long version) {
getClient("").sendCommand(ModuleCommand.EXAPPEND, key, value, SafeEncoder.encode(nxxx),
SafeEncoder.encode(verabs), toByteArray(version));
return getResponse(BuilderFactory.LONG);
}
public Response exprepend(String key, String value, String nxxx, String verabs, long version) {
return exprepend(SafeEncoder.encode(key), SafeEncoder.encode(value), nxxx, verabs, version);
}
public Response exprepend(byte[] key, byte[] value, String nxxx, String verabs, long version) {
getClient("").sendCommand(ModuleCommand.EXPREPEND, key, value, SafeEncoder.encode(nxxx),
SafeEncoder.encode(verabs), toByteArray(version));
return getResponse(BuilderFactory.LONG);
}
public Response> exgae(String key, String expxwithat, long time) {
getClient("").sendCommand(ModuleCommand.EXGAE, key, expxwithat, Long.toString(time));
return getResponse(StringBuilderFactory.EXGET_RESULT_STRING);
}
public Response> exgae(byte[] key, String expxwithat, long time) {
getClient("").sendCommand(ModuleCommand.EXGAE, key, SafeEncoder.encode(expxwithat),
toByteArray(time));
return getResponse(StringBuilderFactory.EXGET_RESULT_BYTE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy