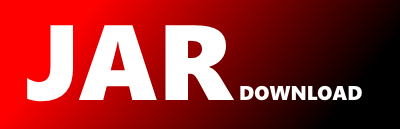
com.aliyun.sdk.service.apig20240327.DefaultAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-apig20240327 Show documentation
Show all versions of alibabacloud-apig20240327 Show documentation
Alibaba Cloud APIG (20240327) Async SDK for Java
The newest version!
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.apig20240327;
import com.aliyun.core.http.*;
import com.aliyun.sdk.service.apig20240327.models.*;
import darabonba.core.utils.*;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import java.util.concurrent.CompletableFuture;
/**
* Main client.
*/
public final class DefaultAsyncClient implements AsyncClient {
protected final String product;
protected final String version;
protected final String endpointRule;
protected final java.util.Map endpointMap;
protected final TeaRequest REQUEST;
protected final TeaAsyncHandler handler;
protected DefaultAsyncClient(ClientConfiguration configuration) {
this.handler = new TeaAsyncHandler(configuration);
this.product = "APIG";
this.version = "2024-03-27";
this.endpointRule = "";
this.endpointMap = new java.util.HashMap<>();
this.REQUEST = TeaRequest.create().setProduct(product).setEndpointRule(endpointRule).setEndpointMap(endpointMap).setVersion(version);
}
@Override
public void close() {
this.handler.close();
}
/**
* @param request the request parameters of AddGatewaySecurityGroupRule AddGatewaySecurityGroupRuleRequest
* @return AddGatewaySecurityGroupRuleResponse
*/
@Override
public CompletableFuture addGatewaySecurityGroupRule(AddGatewaySecurityGroupRuleRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("AddGatewaySecurityGroupRule").setMethod(HttpMethod.POST).setPathRegex("/v1/gateways/{gatewayId}/security-group-rules").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(AddGatewaySecurityGroupRuleResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Create Domain.
*
* @param request the request parameters of CreateDomain CreateDomainRequest
* @return CreateDomainResponse
*/
@Override
public CompletableFuture createDomain(CreateDomainRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("CreateDomain").setMethod(HttpMethod.POST).setPathRegex("/v1/domains").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CreateDomainResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Create environment.
*
* @param request the request parameters of CreateEnvironment CreateEnvironmentRequest
* @return CreateEnvironmentResponse
*/
@Override
public CompletableFuture createEnvironment(CreateEnvironmentRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("CreateEnvironment").setMethod(HttpMethod.POST).setPathRegex("/v1/environments").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CreateEnvironmentResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of CreateHttpApi CreateHttpApiRequest
* @return CreateHttpApiResponse
*/
@Override
public CompletableFuture createHttpApi(CreateHttpApiRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("CreateHttpApi").setMethod(HttpMethod.POST).setPathRegex("/v1/http-apis").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CreateHttpApiResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of CreateHttpApiOperation CreateHttpApiOperationRequest
* @return CreateHttpApiOperationResponse
*/
@Override
public CompletableFuture createHttpApiOperation(CreateHttpApiOperationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("CreateHttpApiOperation").setMethod(HttpMethod.POST).setPathRegex("/v1/http-apis/{httpApiId}/operations").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CreateHttpApiOperationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DeleteDomain DeleteDomainRequest
* @return DeleteDomainResponse
*/
@Override
public CompletableFuture deleteDomain(DeleteDomainRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("DeleteDomain").setMethod(HttpMethod.DELETE).setPathRegex("/v1/domains/{domainId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DeleteDomainResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DeleteEnvironment DeleteEnvironmentRequest
* @return DeleteEnvironmentResponse
*/
@Override
public CompletableFuture deleteEnvironment(DeleteEnvironmentRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("DeleteEnvironment").setMethod(HttpMethod.DELETE).setPathRegex("/v1/environments/{environmentId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DeleteEnvironmentResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DeleteGateway DeleteGatewayRequest
* @return DeleteGatewayResponse
*/
@Override
public CompletableFuture deleteGateway(DeleteGatewayRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("DeleteGateway").setMethod(HttpMethod.DELETE).setPathRegex("/v1/gateways/{gatewayId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DeleteGatewayResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DeleteHttpApi DeleteHttpApiRequest
* @return DeleteHttpApiResponse
*/
@Override
public CompletableFuture deleteHttpApi(DeleteHttpApiRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("DeleteHttpApi").setMethod(HttpMethod.DELETE).setPathRegex("/v1/http-apis/{httpApiId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DeleteHttpApiResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DeleteHttpApiOperation DeleteHttpApiOperationRequest
* @return DeleteHttpApiOperationResponse
*/
@Override
public CompletableFuture deleteHttpApiOperation(DeleteHttpApiOperationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("DeleteHttpApiOperation").setMethod(HttpMethod.DELETE).setPathRegex("/v1/http-apis/{httpApiId}/operations/{operationId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DeleteHttpApiOperationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetDomain GetDomainRequest
* @return GetDomainResponse
*/
@Override
public CompletableFuture getDomain(GetDomainRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("GetDomain").setMethod(HttpMethod.GET).setPathRegex("/v1/domains/{domainId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetDomainResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetEnvironment GetEnvironmentRequest
* @return GetEnvironmentResponse
*/
@Override
public CompletableFuture getEnvironment(GetEnvironmentRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("GetEnvironment").setMethod(HttpMethod.GET).setPathRegex("/v1/environments/{environmentId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetEnvironmentResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetGateway GetGatewayRequest
* @return GetGatewayResponse
*/
@Override
public CompletableFuture getGateway(GetGatewayRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("GetGateway").setMethod(HttpMethod.GET).setPathRegex("/v1/gateways/{gatewayId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetGatewayResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetHttpApi GetHttpApiRequest
* @return GetHttpApiResponse
*/
@Override
public CompletableFuture getHttpApi(GetHttpApiRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("GetHttpApi").setMethod(HttpMethod.GET).setPathRegex("/v1/http-apis/{httpApiId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetHttpApiResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetHttpApiOperation GetHttpApiOperationRequest
* @return GetHttpApiOperationResponse
*/
@Override
public CompletableFuture getHttpApiOperation(GetHttpApiOperationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("GetHttpApiOperation").setMethod(HttpMethod.GET).setPathRegex("/v1/http-apis/{httpApiId}/operations/{operationId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetHttpApiOperationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetHttpApiRoute GetHttpApiRouteRequest
* @return GetHttpApiRouteResponse
*/
@Override
public CompletableFuture getHttpApiRoute(GetHttpApiRouteRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("GetHttpApiRoute").setMethod(HttpMethod.GET).setPathRegex("/v1/http-apis/{httpApiId}/routes/{routeId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetHttpApiRouteResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of ListDomains ListDomainsRequest
* @return ListDomainsResponse
*/
@Override
public CompletableFuture listDomains(ListDomainsRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("ListDomains").setMethod(HttpMethod.GET).setPathRegex("/v1/domains").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(ListDomainsResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of ListEnvironments ListEnvironmentsRequest
* @return ListEnvironmentsResponse
*/
@Override
public CompletableFuture listEnvironments(ListEnvironmentsRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("ListEnvironments").setMethod(HttpMethod.GET).setPathRegex("/v1/environments").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(ListEnvironmentsResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of ListGateways ListGatewaysRequest
* @return ListGatewaysResponse
*/
@Override
public CompletableFuture listGateways(ListGatewaysRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("ListGateways").setMethod(HttpMethod.GET).setPathRegex("/v1/gateways").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(ListGatewaysResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of ListHttpApiOperations ListHttpApiOperationsRequest
* @return ListHttpApiOperationsResponse
*/
@Override
public CompletableFuture listHttpApiOperations(ListHttpApiOperationsRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("ListHttpApiOperations").setMethod(HttpMethod.GET).setPathRegex("/v1/http-apis/{httpApiId}/operations").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(ListHttpApiOperationsResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of ListHttpApis ListHttpApisRequest
* @return ListHttpApisResponse
*/
@Override
public CompletableFuture listHttpApis(ListHttpApisRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("ListHttpApis").setMethod(HttpMethod.GET).setPathRegex("/v1/http-apis").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(ListHttpApisResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UpdateDomain UpdateDomainRequest
* @return UpdateDomainResponse
*/
@Override
public CompletableFuture updateDomain(UpdateDomainRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("UpdateDomain").setMethod(HttpMethod.PUT).setPathRegex("/v1/domains/{domainId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UpdateDomainResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UpdateEnvironment UpdateEnvironmentRequest
* @return UpdateEnvironmentResponse
*/
@Override
public CompletableFuture updateEnvironment(UpdateEnvironmentRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("UpdateEnvironment").setMethod(HttpMethod.PUT).setPathRegex("/v1/environments/{environmentId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UpdateEnvironmentResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UpdateHttpApi UpdateHttpApiRequest
* @return UpdateHttpApiResponse
*/
@Override
public CompletableFuture updateHttpApi(UpdateHttpApiRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("UpdateHttpApi").setMethod(HttpMethod.PUT).setPathRegex("/v1/http-apis/{httpApiId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UpdateHttpApiResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UpdateHttpApiOperation UpdateHttpApiOperationRequest
* @return UpdateHttpApiOperationResponse
*/
@Override
public CompletableFuture updateHttpApiOperation(UpdateHttpApiOperationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RESTFUL).setAction("UpdateHttpApiOperation").setMethod(HttpMethod.POST).setPathRegex("/v1/http-apis/{httpApiId}/operations/{operationId}").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UpdateHttpApiOperationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy