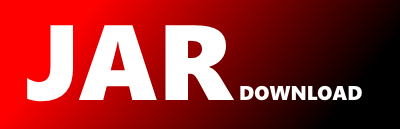
com.aliyun.sdk.service.apig20240327.models.HttpApiApiInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-apig20240327 Show documentation
Show all versions of alibabacloud-apig20240327 Show documentation
Alibaba Cloud APIG (20240327) Async SDK for Java
The newest version!
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.apig20240327.models;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public class HttpApiApiInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("aiProtocols")
private java.util.List < String > aiProtocols;
@com.aliyun.core.annotation.NameInMap("basePath")
private String basePath;
@com.aliyun.core.annotation.NameInMap("deployConfigs")
private java.util.List < HttpApiDeployConfig > deployConfigs;
@com.aliyun.core.annotation.NameInMap("description")
private String description;
@com.aliyun.core.annotation.NameInMap("environments")
private java.util.List < Environments> environments;
@com.aliyun.core.annotation.NameInMap("httpApiId")
private String httpApiId;
@com.aliyun.core.annotation.NameInMap("ingressInfo")
private IngressInfo ingressInfo;
@com.aliyun.core.annotation.NameInMap("name")
private String name;
@com.aliyun.core.annotation.NameInMap("protocols")
private java.util.List < String > protocols;
@com.aliyun.core.annotation.NameInMap("resourceGroupId")
private String resourceGroupId;
@com.aliyun.core.annotation.NameInMap("type")
private String type;
@com.aliyun.core.annotation.NameInMap("versionInfo")
private HttpApiVersionInfo versionInfo;
private HttpApiApiInfo(Builder builder) {
this.aiProtocols = builder.aiProtocols;
this.basePath = builder.basePath;
this.deployConfigs = builder.deployConfigs;
this.description = builder.description;
this.environments = builder.environments;
this.httpApiId = builder.httpApiId;
this.ingressInfo = builder.ingressInfo;
this.name = builder.name;
this.protocols = builder.protocols;
this.resourceGroupId = builder.resourceGroupId;
this.type = builder.type;
this.versionInfo = builder.versionInfo;
}
public static Builder builder() {
return new Builder();
}
public static HttpApiApiInfo create() {
return builder().build();
}
/**
* @return aiProtocols
*/
public java.util.List < String > getAiProtocols() {
return this.aiProtocols;
}
/**
* @return basePath
*/
public String getBasePath() {
return this.basePath;
}
/**
* @return deployConfigs
*/
public java.util.List < HttpApiDeployConfig > getDeployConfigs() {
return this.deployConfigs;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return environments
*/
public java.util.List < Environments> getEnvironments() {
return this.environments;
}
/**
* @return httpApiId
*/
public String getHttpApiId() {
return this.httpApiId;
}
/**
* @return ingressInfo
*/
public IngressInfo getIngressInfo() {
return this.ingressInfo;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return protocols
*/
public java.util.List < String > getProtocols() {
return this.protocols;
}
/**
* @return resourceGroupId
*/
public String getResourceGroupId() {
return this.resourceGroupId;
}
/**
* @return type
*/
public String getType() {
return this.type;
}
/**
* @return versionInfo
*/
public HttpApiVersionInfo getVersionInfo() {
return this.versionInfo;
}
public static final class Builder {
private java.util.List < String > aiProtocols;
private String basePath;
private java.util.List < HttpApiDeployConfig > deployConfigs;
private String description;
private java.util.List < Environments> environments;
private String httpApiId;
private IngressInfo ingressInfo;
private String name;
private java.util.List < String > protocols;
private String resourceGroupId;
private String type;
private HttpApiVersionInfo versionInfo;
/**
* aiProtocols.
*/
public Builder aiProtocols(java.util.List < String > aiProtocols) {
this.aiProtocols = aiProtocols;
return this;
}
/**
* basePath.
*/
public Builder basePath(String basePath) {
this.basePath = basePath;
return this;
}
/**
* deployConfigs.
*/
public Builder deployConfigs(java.util.List < HttpApiDeployConfig > deployConfigs) {
this.deployConfigs = deployConfigs;
return this;
}
/**
* description.
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* environments.
*/
public Builder environments(java.util.List < Environments> environments) {
this.environments = environments;
return this;
}
/**
* httpApiId.
*/
public Builder httpApiId(String httpApiId) {
this.httpApiId = httpApiId;
return this;
}
/**
* ingressInfo.
*/
public Builder ingressInfo(IngressInfo ingressInfo) {
this.ingressInfo = ingressInfo;
return this;
}
/**
* name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* protocols.
*/
public Builder protocols(java.util.List < String > protocols) {
this.protocols = protocols;
return this;
}
/**
* resourceGroupId.
*/
public Builder resourceGroupId(String resourceGroupId) {
this.resourceGroupId = resourceGroupId;
return this;
}
/**
* type.
*/
public Builder type(String type) {
this.type = type;
return this;
}
/**
* versionInfo.
*/
public Builder versionInfo(HttpApiVersionInfo versionInfo) {
this.versionInfo = versionInfo;
return this;
}
public HttpApiApiInfo build() {
return new HttpApiApiInfo(this);
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class GatewayInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("gatewayId")
private String gatewayId;
@com.aliyun.core.annotation.NameInMap("name")
private String name;
private GatewayInfo(Builder builder) {
this.gatewayId = builder.gatewayId;
this.name = builder.name;
}
public static Builder builder() {
return new Builder();
}
public static GatewayInfo create() {
return builder().build();
}
/**
* @return gatewayId
*/
public String getGatewayId() {
return this.gatewayId;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
public static final class Builder {
private String gatewayId;
private String name;
/**
* gatewayId.
*/
public Builder gatewayId(String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
/**
* name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
public GatewayInfo build() {
return new GatewayInfo(this);
}
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class ServiceConfigs extends TeaModel {
@com.aliyun.core.annotation.NameInMap("gatewayServiceId")
private String gatewayServiceId;
@com.aliyun.core.annotation.NameInMap("match")
private HttpApiBackendMatchConditions match;
@com.aliyun.core.annotation.NameInMap("name")
private String name;
@com.aliyun.core.annotation.NameInMap("port")
private String port;
@com.aliyun.core.annotation.NameInMap("protocol")
private String protocol;
@com.aliyun.core.annotation.NameInMap("serviceId")
private String serviceId;
@com.aliyun.core.annotation.NameInMap("version")
private String version;
@com.aliyun.core.annotation.NameInMap("weight")
private Integer weight;
private ServiceConfigs(Builder builder) {
this.gatewayServiceId = builder.gatewayServiceId;
this.match = builder.match;
this.name = builder.name;
this.port = builder.port;
this.protocol = builder.protocol;
this.serviceId = builder.serviceId;
this.version = builder.version;
this.weight = builder.weight;
}
public static Builder builder() {
return new Builder();
}
public static ServiceConfigs create() {
return builder().build();
}
/**
* @return gatewayServiceId
*/
public String getGatewayServiceId() {
return this.gatewayServiceId;
}
/**
* @return match
*/
public HttpApiBackendMatchConditions getMatch() {
return this.match;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return port
*/
public String getPort() {
return this.port;
}
/**
* @return protocol
*/
public String getProtocol() {
return this.protocol;
}
/**
* @return serviceId
*/
public String getServiceId() {
return this.serviceId;
}
/**
* @return version
*/
public String getVersion() {
return this.version;
}
/**
* @return weight
*/
public Integer getWeight() {
return this.weight;
}
public static final class Builder {
private String gatewayServiceId;
private HttpApiBackendMatchConditions match;
private String name;
private String port;
private String protocol;
private String serviceId;
private String version;
private Integer weight;
/**
* gatewayServiceId.
*/
public Builder gatewayServiceId(String gatewayServiceId) {
this.gatewayServiceId = gatewayServiceId;
return this;
}
/**
* match.
*/
public Builder match(HttpApiBackendMatchConditions match) {
this.match = match;
return this;
}
/**
* name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* port.
*/
public Builder port(String port) {
this.port = port;
return this;
}
/**
* protocol.
*/
public Builder protocol(String protocol) {
this.protocol = protocol;
return this;
}
/**
* serviceId.
*/
public Builder serviceId(String serviceId) {
this.serviceId = serviceId;
return this;
}
/**
* version.
*/
public Builder version(String version) {
this.version = version;
return this;
}
/**
* weight.
*/
public Builder weight(Integer weight) {
this.weight = weight;
return this;
}
public ServiceConfigs build() {
return new ServiceConfigs(this);
}
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class SubDomains extends TeaModel {
@com.aliyun.core.annotation.NameInMap("domainId")
private String domainId;
@com.aliyun.core.annotation.NameInMap("name")
private String name;
@com.aliyun.core.annotation.NameInMap("networkType")
private String networkType;
@com.aliyun.core.annotation.NameInMap("protocol")
private String protocol;
private SubDomains(Builder builder) {
this.domainId = builder.domainId;
this.name = builder.name;
this.networkType = builder.networkType;
this.protocol = builder.protocol;
}
public static Builder builder() {
return new Builder();
}
public static SubDomains create() {
return builder().build();
}
/**
* @return domainId
*/
public String getDomainId() {
return this.domainId;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return networkType
*/
public String getNetworkType() {
return this.networkType;
}
/**
* @return protocol
*/
public String getProtocol() {
return this.protocol;
}
public static final class Builder {
private String domainId;
private String name;
private String networkType;
private String protocol;
/**
* domainId.
*/
public Builder domainId(String domainId) {
this.domainId = domainId;
return this;
}
/**
* name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* networkType.
*/
public Builder networkType(String networkType) {
this.networkType = networkType;
return this;
}
/**
* protocol.
*/
public Builder protocol(String protocol) {
this.protocol = protocol;
return this;
}
public SubDomains build() {
return new SubDomains(this);
}
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class Environments extends TeaModel {
@com.aliyun.core.annotation.NameInMap("alias")
private String alias;
@com.aliyun.core.annotation.NameInMap("backendScene")
private String backendScene;
@com.aliyun.core.annotation.NameInMap("backendType")
private String backendType;
@com.aliyun.core.annotation.NameInMap("customDomains")
private java.util.List < HttpApiDomainInfo > customDomains;
@com.aliyun.core.annotation.NameInMap("deployStatus")
private String deployStatus;
@com.aliyun.core.annotation.NameInMap("environmentId")
private String environmentId;
@com.aliyun.core.annotation.NameInMap("gatewayInfo")
private GatewayInfo gatewayInfo;
@com.aliyun.core.annotation.NameInMap("name")
private String name;
@com.aliyun.core.annotation.NameInMap("serviceConfigs")
private java.util.List < ServiceConfigs> serviceConfigs;
@com.aliyun.core.annotation.NameInMap("subDomains")
private java.util.List < SubDomains> subDomains;
private Environments(Builder builder) {
this.alias = builder.alias;
this.backendScene = builder.backendScene;
this.backendType = builder.backendType;
this.customDomains = builder.customDomains;
this.deployStatus = builder.deployStatus;
this.environmentId = builder.environmentId;
this.gatewayInfo = builder.gatewayInfo;
this.name = builder.name;
this.serviceConfigs = builder.serviceConfigs;
this.subDomains = builder.subDomains;
}
public static Builder builder() {
return new Builder();
}
public static Environments create() {
return builder().build();
}
/**
* @return alias
*/
public String getAlias() {
return this.alias;
}
/**
* @return backendScene
*/
public String getBackendScene() {
return this.backendScene;
}
/**
* @return backendType
*/
public String getBackendType() {
return this.backendType;
}
/**
* @return customDomains
*/
public java.util.List < HttpApiDomainInfo > getCustomDomains() {
return this.customDomains;
}
/**
* @return deployStatus
*/
public String getDeployStatus() {
return this.deployStatus;
}
/**
* @return environmentId
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
* @return gatewayInfo
*/
public GatewayInfo getGatewayInfo() {
return this.gatewayInfo;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return serviceConfigs
*/
public java.util.List < ServiceConfigs> getServiceConfigs() {
return this.serviceConfigs;
}
/**
* @return subDomains
*/
public java.util.List < SubDomains> getSubDomains() {
return this.subDomains;
}
public static final class Builder {
private String alias;
private String backendScene;
private String backendType;
private java.util.List < HttpApiDomainInfo > customDomains;
private String deployStatus;
private String environmentId;
private GatewayInfo gatewayInfo;
private String name;
private java.util.List < ServiceConfigs> serviceConfigs;
private java.util.List < SubDomains> subDomains;
/**
* alias.
*/
public Builder alias(String alias) {
this.alias = alias;
return this;
}
/**
* backendScene.
*/
public Builder backendScene(String backendScene) {
this.backendScene = backendScene;
return this;
}
/**
* backendType.
*/
public Builder backendType(String backendType) {
this.backendType = backendType;
return this;
}
/**
* customDomains.
*/
public Builder customDomains(java.util.List < HttpApiDomainInfo > customDomains) {
this.customDomains = customDomains;
return this;
}
/**
* deployStatus.
*/
public Builder deployStatus(String deployStatus) {
this.deployStatus = deployStatus;
return this;
}
/**
* environmentId.
*/
public Builder environmentId(String environmentId) {
this.environmentId = environmentId;
return this;
}
/**
* gatewayInfo.
*/
public Builder gatewayInfo(GatewayInfo gatewayInfo) {
this.gatewayInfo = gatewayInfo;
return this;
}
/**
* name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* serviceConfigs.
*/
public Builder serviceConfigs(java.util.List < ServiceConfigs> serviceConfigs) {
this.serviceConfigs = serviceConfigs;
return this;
}
/**
* subDomains.
*/
public Builder subDomains(java.util.List < SubDomains> subDomains) {
this.subDomains = subDomains;
return this;
}
public Environments build() {
return new Environments(this);
}
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class EnvironmentInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("environmentId")
private String environmentId;
private EnvironmentInfo(Builder builder) {
this.environmentId = builder.environmentId;
}
public static Builder builder() {
return new Builder();
}
public static EnvironmentInfo create() {
return builder().build();
}
/**
* @return environmentId
*/
public String getEnvironmentId() {
return this.environmentId;
}
public static final class Builder {
private String environmentId;
/**
* environmentId.
*/
public Builder environmentId(String environmentId) {
this.environmentId = environmentId;
return this;
}
public EnvironmentInfo build() {
return new EnvironmentInfo(this);
}
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class K8sClusterInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("clusterId")
private String clusterId;
private K8sClusterInfo(Builder builder) {
this.clusterId = builder.clusterId;
}
public static Builder builder() {
return new Builder();
}
public static K8sClusterInfo create() {
return builder().build();
}
/**
* @return clusterId
*/
public String getClusterId() {
return this.clusterId;
}
public static final class Builder {
private String clusterId;
/**
* clusterId.
*/
public Builder clusterId(String clusterId) {
this.clusterId = clusterId;
return this;
}
public K8sClusterInfo build() {
return new K8sClusterInfo(this);
}
}
}
/**
*
* {@link HttpApiApiInfo} extends {@link TeaModel}
*
* HttpApiApiInfo
*/
public static class IngressInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("environmentInfo")
private EnvironmentInfo environmentInfo;
@com.aliyun.core.annotation.NameInMap("ingressClass")
private String ingressClass;
@com.aliyun.core.annotation.NameInMap("k8sClusterInfo")
private K8sClusterInfo k8sClusterInfo;
@com.aliyun.core.annotation.NameInMap("overrideIngressIp")
private Boolean overrideIngressIp;
@com.aliyun.core.annotation.NameInMap("sourceId")
private String sourceId;
@com.aliyun.core.annotation.NameInMap("watchNamespace")
private String watchNamespace;
private IngressInfo(Builder builder) {
this.environmentInfo = builder.environmentInfo;
this.ingressClass = builder.ingressClass;
this.k8sClusterInfo = builder.k8sClusterInfo;
this.overrideIngressIp = builder.overrideIngressIp;
this.sourceId = builder.sourceId;
this.watchNamespace = builder.watchNamespace;
}
public static Builder builder() {
return new Builder();
}
public static IngressInfo create() {
return builder().build();
}
/**
* @return environmentInfo
*/
public EnvironmentInfo getEnvironmentInfo() {
return this.environmentInfo;
}
/**
* @return ingressClass
*/
public String getIngressClass() {
return this.ingressClass;
}
/**
* @return k8sClusterInfo
*/
public K8sClusterInfo getK8sClusterInfo() {
return this.k8sClusterInfo;
}
/**
* @return overrideIngressIp
*/
public Boolean getOverrideIngressIp() {
return this.overrideIngressIp;
}
/**
* @return sourceId
*/
public String getSourceId() {
return this.sourceId;
}
/**
* @return watchNamespace
*/
public String getWatchNamespace() {
return this.watchNamespace;
}
public static final class Builder {
private EnvironmentInfo environmentInfo;
private String ingressClass;
private K8sClusterInfo k8sClusterInfo;
private Boolean overrideIngressIp;
private String sourceId;
private String watchNamespace;
/**
* environmentInfo.
*/
public Builder environmentInfo(EnvironmentInfo environmentInfo) {
this.environmentInfo = environmentInfo;
return this;
}
/**
* ingressClass.
*/
public Builder ingressClass(String ingressClass) {
this.ingressClass = ingressClass;
return this;
}
/**
* k8sClusterInfo.
*/
public Builder k8sClusterInfo(K8sClusterInfo k8sClusterInfo) {
this.k8sClusterInfo = k8sClusterInfo;
return this;
}
/**
* overrideIngressIp.
*/
public Builder overrideIngressIp(Boolean overrideIngressIp) {
this.overrideIngressIp = overrideIngressIp;
return this;
}
/**
* sourceId.
*/
public Builder sourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
/**
* watchNamespace.
*/
public Builder watchNamespace(String watchNamespace) {
this.watchNamespace = watchNamespace;
return this;
}
public IngressInfo build() {
return new IngressInfo(this);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy