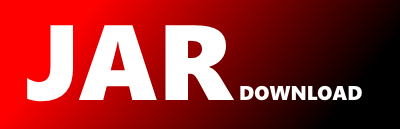
com.aliyun.sdk.service.dataphin_public20230630.models.GetBatchTaskInfoByVersionResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-dataphin_public20230630 Show documentation
Show all versions of alibabacloud-dataphin_public20230630 Show documentation
Alibaba Cloud dataphin-public (20230630) Async SDK for Java
The newest version!
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.dataphin_public20230630.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public class GetBatchTaskInfoByVersionResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Code")
private String code;
@com.aliyun.core.annotation.NameInMap("HttpStatusCode")
private Integer httpStatusCode;
@com.aliyun.core.annotation.NameInMap("Message")
private String message;
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("Success")
private Boolean success;
@com.aliyun.core.annotation.NameInMap("TaskInfo")
private TaskInfo taskInfo;
private GetBatchTaskInfoByVersionResponseBody(Builder builder) {
this.code = builder.code;
this.httpStatusCode = builder.httpStatusCode;
this.message = builder.message;
this.requestId = builder.requestId;
this.success = builder.success;
this.taskInfo = builder.taskInfo;
}
public static Builder builder() {
return new Builder();
}
public static GetBatchTaskInfoByVersionResponseBody create() {
return builder().build();
}
/**
* @return code
*/
public String getCode() {
return this.code;
}
/**
* @return httpStatusCode
*/
public Integer getHttpStatusCode() {
return this.httpStatusCode;
}
/**
* @return message
*/
public String getMessage() {
return this.message;
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return success
*/
public Boolean getSuccess() {
return this.success;
}
/**
* @return taskInfo
*/
public TaskInfo getTaskInfo() {
return this.taskInfo;
}
public static final class Builder {
private String code;
private Integer httpStatusCode;
private String message;
private String requestId;
private Boolean success;
private TaskInfo taskInfo;
/**
* Code.
*/
public Builder code(String code) {
this.code = code;
return this;
}
/**
* HttpStatusCode.
*/
public Builder httpStatusCode(Integer httpStatusCode) {
this.httpStatusCode = httpStatusCode;
return this;
}
/**
* Message.
*/
public Builder message(String message) {
this.message = message;
return this;
}
/**
* RequestId.
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Success.
*/
public Builder success(Boolean success) {
this.success = success;
return this;
}
/**
* TaskInfo.
*/
public Builder taskInfo(TaskInfo taskInfo) {
this.taskInfo = taskInfo;
return this;
}
public GetBatchTaskInfoByVersionResponseBody build() {
return new GetBatchTaskInfoByVersionResponseBody(this);
}
}
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public static class CustomScheduleConfig extends TeaModel {
@com.aliyun.core.annotation.NameInMap("EndTime")
private String endTime;
@com.aliyun.core.annotation.NameInMap("Interval")
private Integer interval;
@com.aliyun.core.annotation.NameInMap("IntervalUnit")
private String intervalUnit;
@com.aliyun.core.annotation.NameInMap("SchedulePeriod")
private String schedulePeriod;
@com.aliyun.core.annotation.NameInMap("StartTime")
private String startTime;
private CustomScheduleConfig(Builder builder) {
this.endTime = builder.endTime;
this.interval = builder.interval;
this.intervalUnit = builder.intervalUnit;
this.schedulePeriod = builder.schedulePeriod;
this.startTime = builder.startTime;
}
public static Builder builder() {
return new Builder();
}
public static CustomScheduleConfig create() {
return builder().build();
}
/**
* @return endTime
*/
public String getEndTime() {
return this.endTime;
}
/**
* @return interval
*/
public Integer getInterval() {
return this.interval;
}
/**
* @return intervalUnit
*/
public String getIntervalUnit() {
return this.intervalUnit;
}
/**
* @return schedulePeriod
*/
public String getSchedulePeriod() {
return this.schedulePeriod;
}
/**
* @return startTime
*/
public String getStartTime() {
return this.startTime;
}
public static final class Builder {
private String endTime;
private Integer interval;
private String intervalUnit;
private String schedulePeriod;
private String startTime;
/**
* EndTime.
*/
public Builder endTime(String endTime) {
this.endTime = endTime;
return this;
}
/**
* Interval.
*/
public Builder interval(Integer interval) {
this.interval = interval;
return this;
}
/**
* IntervalUnit.
*/
public Builder intervalUnit(String intervalUnit) {
this.intervalUnit = intervalUnit;
return this;
}
/**
* SchedulePeriod.
*/
public Builder schedulePeriod(String schedulePeriod) {
this.schedulePeriod = schedulePeriod;
return this;
}
/**
* StartTime.
*/
public Builder startTime(String startTime) {
this.startTime = startTime;
return this;
}
public CustomScheduleConfig build() {
return new CustomScheduleConfig(this);
}
}
}
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public static class ParamList extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Key")
private String key;
@com.aliyun.core.annotation.NameInMap("Value")
private String value;
private ParamList(Builder builder) {
this.key = builder.key;
this.value = builder.value;
}
public static Builder builder() {
return new Builder();
}
public static ParamList create() {
return builder().build();
}
/**
* @return key
*/
public String getKey() {
return this.key;
}
/**
* @return value
*/
public String getValue() {
return this.value;
}
public static final class Builder {
private String key;
private String value;
/**
* Key.
*/
public Builder key(String key) {
this.key = key;
return this;
}
/**
* Value.
*/
public Builder value(String value) {
this.value = value;
return this;
}
public ParamList build() {
return new ParamList(this);
}
}
}
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public static class SparkClientInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("SparkClientVersion")
private String sparkClientVersion;
private SparkClientInfo(Builder builder) {
this.sparkClientVersion = builder.sparkClientVersion;
}
public static Builder builder() {
return new Builder();
}
public static SparkClientInfo create() {
return builder().build();
}
/**
* @return sparkClientVersion
*/
public String getSparkClientVersion() {
return this.sparkClientVersion;
}
public static final class Builder {
private String sparkClientVersion;
/**
* SparkClientVersion.
*/
public Builder sparkClientVersion(String sparkClientVersion) {
this.sparkClientVersion = sparkClientVersion;
return this;
}
public SparkClientInfo build() {
return new SparkClientInfo(this);
}
}
}
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public static class DependPeriod extends TeaModel {
@com.aliyun.core.annotation.NameInMap("PeriodOffset")
private Integer periodOffset;
@com.aliyun.core.annotation.NameInMap("PeriodType")
private String periodType;
private DependPeriod(Builder builder) {
this.periodOffset = builder.periodOffset;
this.periodType = builder.periodType;
}
public static Builder builder() {
return new Builder();
}
public static DependPeriod create() {
return builder().build();
}
/**
* @return periodOffset
*/
public Integer getPeriodOffset() {
return this.periodOffset;
}
/**
* @return periodType
*/
public String getPeriodType() {
return this.periodType;
}
public static final class Builder {
private Integer periodOffset;
private String periodType;
/**
* PeriodOffset.
*/
public Builder periodOffset(Integer periodOffset) {
this.periodOffset = periodOffset;
return this;
}
/**
* PeriodType.
*/
public Builder periodType(String periodType) {
this.periodType = periodType;
return this;
}
public DependPeriod build() {
return new DependPeriod(this);
}
}
}
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public static class UpStreamList extends TeaModel {
@com.aliyun.core.annotation.NameInMap("DependPeriod")
private DependPeriod dependPeriod;
@com.aliyun.core.annotation.NameInMap("DependStrategy")
private String dependStrategy;
@com.aliyun.core.annotation.NameInMap("FieldList")
private java.util.List fieldList;
@com.aliyun.core.annotation.NameInMap("NodeType")
private String nodeType;
@com.aliyun.core.annotation.NameInMap("PeriodDiff")
private Integer periodDiff;
@com.aliyun.core.annotation.NameInMap("SourceNodeEnabled")
private Boolean sourceNodeEnabled;
@com.aliyun.core.annotation.NameInMap("SourceNodeId")
private String sourceNodeId;
@com.aliyun.core.annotation.NameInMap("SourceNodeName")
private String sourceNodeName;
@com.aliyun.core.annotation.NameInMap("SourceNodeOutputName")
private String sourceNodeOutputName;
@com.aliyun.core.annotation.NameInMap("SourceNodeUserName")
private String sourceNodeUserName;
@com.aliyun.core.annotation.NameInMap("SourceTableName")
private String sourceTableName;
private UpStreamList(Builder builder) {
this.dependPeriod = builder.dependPeriod;
this.dependStrategy = builder.dependStrategy;
this.fieldList = builder.fieldList;
this.nodeType = builder.nodeType;
this.periodDiff = builder.periodDiff;
this.sourceNodeEnabled = builder.sourceNodeEnabled;
this.sourceNodeId = builder.sourceNodeId;
this.sourceNodeName = builder.sourceNodeName;
this.sourceNodeOutputName = builder.sourceNodeOutputName;
this.sourceNodeUserName = builder.sourceNodeUserName;
this.sourceTableName = builder.sourceTableName;
}
public static Builder builder() {
return new Builder();
}
public static UpStreamList create() {
return builder().build();
}
/**
* @return dependPeriod
*/
public DependPeriod getDependPeriod() {
return this.dependPeriod;
}
/**
* @return dependStrategy
*/
public String getDependStrategy() {
return this.dependStrategy;
}
/**
* @return fieldList
*/
public java.util.List getFieldList() {
return this.fieldList;
}
/**
* @return nodeType
*/
public String getNodeType() {
return this.nodeType;
}
/**
* @return periodDiff
*/
public Integer getPeriodDiff() {
return this.periodDiff;
}
/**
* @return sourceNodeEnabled
*/
public Boolean getSourceNodeEnabled() {
return this.sourceNodeEnabled;
}
/**
* @return sourceNodeId
*/
public String getSourceNodeId() {
return this.sourceNodeId;
}
/**
* @return sourceNodeName
*/
public String getSourceNodeName() {
return this.sourceNodeName;
}
/**
* @return sourceNodeOutputName
*/
public String getSourceNodeOutputName() {
return this.sourceNodeOutputName;
}
/**
* @return sourceNodeUserName
*/
public String getSourceNodeUserName() {
return this.sourceNodeUserName;
}
/**
* @return sourceTableName
*/
public String getSourceTableName() {
return this.sourceTableName;
}
public static final class Builder {
private DependPeriod dependPeriod;
private String dependStrategy;
private java.util.List fieldList;
private String nodeType;
private Integer periodDiff;
private Boolean sourceNodeEnabled;
private String sourceNodeId;
private String sourceNodeName;
private String sourceNodeOutputName;
private String sourceNodeUserName;
private String sourceTableName;
/**
* DependPeriod.
*/
public Builder dependPeriod(DependPeriod dependPeriod) {
this.dependPeriod = dependPeriod;
return this;
}
/**
* DependStrategy.
*/
public Builder dependStrategy(String dependStrategy) {
this.dependStrategy = dependStrategy;
return this;
}
/**
* FieldList.
*/
public Builder fieldList(java.util.List fieldList) {
this.fieldList = fieldList;
return this;
}
/**
* NodeType.
*/
public Builder nodeType(String nodeType) {
this.nodeType = nodeType;
return this;
}
/**
* PeriodDiff.
*/
public Builder periodDiff(Integer periodDiff) {
this.periodDiff = periodDiff;
return this;
}
/**
* SourceNodeEnabled.
*/
public Builder sourceNodeEnabled(Boolean sourceNodeEnabled) {
this.sourceNodeEnabled = sourceNodeEnabled;
return this;
}
/**
* SourceNodeId.
*/
public Builder sourceNodeId(String sourceNodeId) {
this.sourceNodeId = sourceNodeId;
return this;
}
/**
* SourceNodeName.
*/
public Builder sourceNodeName(String sourceNodeName) {
this.sourceNodeName = sourceNodeName;
return this;
}
/**
* SourceNodeOutputName.
*/
public Builder sourceNodeOutputName(String sourceNodeOutputName) {
this.sourceNodeOutputName = sourceNodeOutputName;
return this;
}
/**
* SourceNodeUserName.
*/
public Builder sourceNodeUserName(String sourceNodeUserName) {
this.sourceNodeUserName = sourceNodeUserName;
return this;
}
/**
* SourceTableName.
*/
public Builder sourceTableName(String sourceTableName) {
this.sourceTableName = sourceTableName;
return this;
}
public UpStreamList build() {
return new UpStreamList(this);
}
}
}
/**
*
* {@link GetBatchTaskInfoByVersionResponseBody} extends {@link TeaModel}
*
* GetBatchTaskInfoByVersionResponseBody
*/
public static class TaskInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Code")
private String code;
@com.aliyun.core.annotation.NameInMap("CronExpression")
private String cronExpression;
@com.aliyun.core.annotation.NameInMap("CustomScheduleConfig")
private CustomScheduleConfig customScheduleConfig;
@com.aliyun.core.annotation.NameInMap("DagId")
private String dagId;
@com.aliyun.core.annotation.NameInMap("DataSourceCatalog")
private String dataSourceCatalog;
@com.aliyun.core.annotation.NameInMap("DataSourceId")
private String dataSourceId;
@com.aliyun.core.annotation.NameInMap("DataSourceSchema")
private String dataSourceSchema;
@com.aliyun.core.annotation.NameInMap("FileId")
private Long fileId;
@com.aliyun.core.annotation.NameInMap("HasDevNode")
private Boolean hasDevNode;
@com.aliyun.core.annotation.NameInMap("Name")
private String name;
@com.aliyun.core.annotation.NameInMap("NeedPublish")
private Boolean needPublish;
@com.aliyun.core.annotation.NameInMap("NodeDescription")
private String nodeDescription;
@com.aliyun.core.annotation.NameInMap("NodeFrom")
private String nodeFrom;
@com.aliyun.core.annotation.NameInMap("NodeId")
private String nodeId;
@com.aliyun.core.annotation.NameInMap("NodeName")
private String nodeName;
@com.aliyun.core.annotation.NameInMap("NodeOutputNameList")
private java.util.List nodeOutputNameList;
@com.aliyun.core.annotation.NameInMap("NodeStatus")
private Integer nodeStatus;
@com.aliyun.core.annotation.NameInMap("OperatorUserId")
private String operatorUserId;
@com.aliyun.core.annotation.NameInMap("OwnerName")
private String ownerName;
@com.aliyun.core.annotation.NameInMap("OwnerUserId")
private String ownerUserId;
@com.aliyun.core.annotation.NameInMap("ParamList")
private java.util.List paramList;
@com.aliyun.core.annotation.NameInMap("Paused")
private Boolean paused;
@com.aliyun.core.annotation.NameInMap("Priority")
private Integer priority;
@com.aliyun.core.annotation.NameInMap("ProjectId")
private Long projectId;
@com.aliyun.core.annotation.NameInMap("Published")
private Boolean published;
@com.aliyun.core.annotation.NameInMap("Remark")
private String remark;
@com.aliyun.core.annotation.NameInMap("Rerunable")
private Boolean rerunable;
@com.aliyun.core.annotation.NameInMap("SchedulePeriod")
private String schedulePeriod;
@com.aliyun.core.annotation.NameInMap("ScheduleType")
private Integer scheduleType;
@com.aliyun.core.annotation.NameInMap("SparkClientInfo")
private SparkClientInfo sparkClientInfo;
@com.aliyun.core.annotation.NameInMap("Status")
private String status;
@com.aliyun.core.annotation.NameInMap("TaskType")
private Integer taskType;
@com.aliyun.core.annotation.NameInMap("UpStreamList")
private java.util.List upStreamList;
private TaskInfo(Builder builder) {
this.code = builder.code;
this.cronExpression = builder.cronExpression;
this.customScheduleConfig = builder.customScheduleConfig;
this.dagId = builder.dagId;
this.dataSourceCatalog = builder.dataSourceCatalog;
this.dataSourceId = builder.dataSourceId;
this.dataSourceSchema = builder.dataSourceSchema;
this.fileId = builder.fileId;
this.hasDevNode = builder.hasDevNode;
this.name = builder.name;
this.needPublish = builder.needPublish;
this.nodeDescription = builder.nodeDescription;
this.nodeFrom = builder.nodeFrom;
this.nodeId = builder.nodeId;
this.nodeName = builder.nodeName;
this.nodeOutputNameList = builder.nodeOutputNameList;
this.nodeStatus = builder.nodeStatus;
this.operatorUserId = builder.operatorUserId;
this.ownerName = builder.ownerName;
this.ownerUserId = builder.ownerUserId;
this.paramList = builder.paramList;
this.paused = builder.paused;
this.priority = builder.priority;
this.projectId = builder.projectId;
this.published = builder.published;
this.remark = builder.remark;
this.rerunable = builder.rerunable;
this.schedulePeriod = builder.schedulePeriod;
this.scheduleType = builder.scheduleType;
this.sparkClientInfo = builder.sparkClientInfo;
this.status = builder.status;
this.taskType = builder.taskType;
this.upStreamList = builder.upStreamList;
}
public static Builder builder() {
return new Builder();
}
public static TaskInfo create() {
return builder().build();
}
/**
* @return code
*/
public String getCode() {
return this.code;
}
/**
* @return cronExpression
*/
public String getCronExpression() {
return this.cronExpression;
}
/**
* @return customScheduleConfig
*/
public CustomScheduleConfig getCustomScheduleConfig() {
return this.customScheduleConfig;
}
/**
* @return dagId
*/
public String getDagId() {
return this.dagId;
}
/**
* @return dataSourceCatalog
*/
public String getDataSourceCatalog() {
return this.dataSourceCatalog;
}
/**
* @return dataSourceId
*/
public String getDataSourceId() {
return this.dataSourceId;
}
/**
* @return dataSourceSchema
*/
public String getDataSourceSchema() {
return this.dataSourceSchema;
}
/**
* @return fileId
*/
public Long getFileId() {
return this.fileId;
}
/**
* @return hasDevNode
*/
public Boolean getHasDevNode() {
return this.hasDevNode;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return needPublish
*/
public Boolean getNeedPublish() {
return this.needPublish;
}
/**
* @return nodeDescription
*/
public String getNodeDescription() {
return this.nodeDescription;
}
/**
* @return nodeFrom
*/
public String getNodeFrom() {
return this.nodeFrom;
}
/**
* @return nodeId
*/
public String getNodeId() {
return this.nodeId;
}
/**
* @return nodeName
*/
public String getNodeName() {
return this.nodeName;
}
/**
* @return nodeOutputNameList
*/
public java.util.List getNodeOutputNameList() {
return this.nodeOutputNameList;
}
/**
* @return nodeStatus
*/
public Integer getNodeStatus() {
return this.nodeStatus;
}
/**
* @return operatorUserId
*/
public String getOperatorUserId() {
return this.operatorUserId;
}
/**
* @return ownerName
*/
public String getOwnerName() {
return this.ownerName;
}
/**
* @return ownerUserId
*/
public String getOwnerUserId() {
return this.ownerUserId;
}
/**
* @return paramList
*/
public java.util.List getParamList() {
return this.paramList;
}
/**
* @return paused
*/
public Boolean getPaused() {
return this.paused;
}
/**
* @return priority
*/
public Integer getPriority() {
return this.priority;
}
/**
* @return projectId
*/
public Long getProjectId() {
return this.projectId;
}
/**
* @return published
*/
public Boolean getPublished() {
return this.published;
}
/**
* @return remark
*/
public String getRemark() {
return this.remark;
}
/**
* @return rerunable
*/
public Boolean getRerunable() {
return this.rerunable;
}
/**
* @return schedulePeriod
*/
public String getSchedulePeriod() {
return this.schedulePeriod;
}
/**
* @return scheduleType
*/
public Integer getScheduleType() {
return this.scheduleType;
}
/**
* @return sparkClientInfo
*/
public SparkClientInfo getSparkClientInfo() {
return this.sparkClientInfo;
}
/**
* @return status
*/
public String getStatus() {
return this.status;
}
/**
* @return taskType
*/
public Integer getTaskType() {
return this.taskType;
}
/**
* @return upStreamList
*/
public java.util.List getUpStreamList() {
return this.upStreamList;
}
public static final class Builder {
private String code;
private String cronExpression;
private CustomScheduleConfig customScheduleConfig;
private String dagId;
private String dataSourceCatalog;
private String dataSourceId;
private String dataSourceSchema;
private Long fileId;
private Boolean hasDevNode;
private String name;
private Boolean needPublish;
private String nodeDescription;
private String nodeFrom;
private String nodeId;
private String nodeName;
private java.util.List nodeOutputNameList;
private Integer nodeStatus;
private String operatorUserId;
private String ownerName;
private String ownerUserId;
private java.util.List paramList;
private Boolean paused;
private Integer priority;
private Long projectId;
private Boolean published;
private String remark;
private Boolean rerunable;
private String schedulePeriod;
private Integer scheduleType;
private SparkClientInfo sparkClientInfo;
private String status;
private Integer taskType;
private java.util.List upStreamList;
/**
* Code.
*/
public Builder code(String code) {
this.code = code;
return this;
}
/**
* CronExpression.
*/
public Builder cronExpression(String cronExpression) {
this.cronExpression = cronExpression;
return this;
}
/**
* CustomScheduleConfig.
*/
public Builder customScheduleConfig(CustomScheduleConfig customScheduleConfig) {
this.customScheduleConfig = customScheduleConfig;
return this;
}
/**
* DagId.
*/
public Builder dagId(String dagId) {
this.dagId = dagId;
return this;
}
/**
* DataSourceCatalog.
*/
public Builder dataSourceCatalog(String dataSourceCatalog) {
this.dataSourceCatalog = dataSourceCatalog;
return this;
}
/**
* DataSourceId.
*/
public Builder dataSourceId(String dataSourceId) {
this.dataSourceId = dataSourceId;
return this;
}
/**
* DataSourceSchema.
*/
public Builder dataSourceSchema(String dataSourceSchema) {
this.dataSourceSchema = dataSourceSchema;
return this;
}
/**
* FileId.
*/
public Builder fileId(Long fileId) {
this.fileId = fileId;
return this;
}
/**
* HasDevNode.
*/
public Builder hasDevNode(Boolean hasDevNode) {
this.hasDevNode = hasDevNode;
return this;
}
/**
* Name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* NeedPublish.
*/
public Builder needPublish(Boolean needPublish) {
this.needPublish = needPublish;
return this;
}
/**
* NodeDescription.
*/
public Builder nodeDescription(String nodeDescription) {
this.nodeDescription = nodeDescription;
return this;
}
/**
* NodeFrom.
*/
public Builder nodeFrom(String nodeFrom) {
this.nodeFrom = nodeFrom;
return this;
}
/**
* NodeId.
*/
public Builder nodeId(String nodeId) {
this.nodeId = nodeId;
return this;
}
/**
* NodeName.
*/
public Builder nodeName(String nodeName) {
this.nodeName = nodeName;
return this;
}
/**
* NodeOutputNameList.
*/
public Builder nodeOutputNameList(java.util.List nodeOutputNameList) {
this.nodeOutputNameList = nodeOutputNameList;
return this;
}
/**
* NodeStatus.
*/
public Builder nodeStatus(Integer nodeStatus) {
this.nodeStatus = nodeStatus;
return this;
}
/**
* OperatorUserId.
*/
public Builder operatorUserId(String operatorUserId) {
this.operatorUserId = operatorUserId;
return this;
}
/**
* OwnerName.
*/
public Builder ownerName(String ownerName) {
this.ownerName = ownerName;
return this;
}
/**
* OwnerUserId.
*/
public Builder ownerUserId(String ownerUserId) {
this.ownerUserId = ownerUserId;
return this;
}
/**
* ParamList.
*/
public Builder paramList(java.util.List paramList) {
this.paramList = paramList;
return this;
}
/**
* Paused.
*/
public Builder paused(Boolean paused) {
this.paused = paused;
return this;
}
/**
* Priority.
*/
public Builder priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* ProjectId.
*/
public Builder projectId(Long projectId) {
this.projectId = projectId;
return this;
}
/**
* Published.
*/
public Builder published(Boolean published) {
this.published = published;
return this;
}
/**
* Remark.
*/
public Builder remark(String remark) {
this.remark = remark;
return this;
}
/**
* Rerunable.
*/
public Builder rerunable(Boolean rerunable) {
this.rerunable = rerunable;
return this;
}
/**
* SchedulePeriod.
*/
public Builder schedulePeriod(String schedulePeriod) {
this.schedulePeriod = schedulePeriod;
return this;
}
/**
* ScheduleType.
*/
public Builder scheduleType(Integer scheduleType) {
this.scheduleType = scheduleType;
return this;
}
/**
* SparkClientInfo.
*/
public Builder sparkClientInfo(SparkClientInfo sparkClientInfo) {
this.sparkClientInfo = sparkClientInfo;
return this;
}
/**
* Status.
*/
public Builder status(String status) {
this.status = status;
return this;
}
/**
* TaskType.
*/
public Builder taskType(Integer taskType) {
this.taskType = taskType;
return this;
}
/**
* UpStreamList.
*/
public Builder upStreamList(java.util.List upStreamList) {
this.upStreamList = upStreamList;
return this;
}
public TaskInfo build() {
return new TaskInfo(this);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy