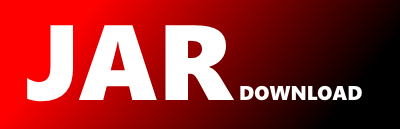
com.aliyun.sdk.service.dataphin_public20230630.models.GetSupplementDagrunInstanceResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-dataphin_public20230630 Show documentation
Show all versions of alibabacloud-dataphin_public20230630 Show documentation
Alibaba Cloud dataphin-public (20230630) Async SDK for Java
The newest version!
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.dataphin_public20230630.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link GetSupplementDagrunInstanceResponseBody} extends {@link TeaModel}
*
* GetSupplementDagrunInstanceResponseBody
*/
public class GetSupplementDagrunInstanceResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Code")
private String code;
@com.aliyun.core.annotation.NameInMap("HttpStatusCode")
private Integer httpStatusCode;
@com.aliyun.core.annotation.NameInMap("InstanceList")
private java.util.List instanceList;
@com.aliyun.core.annotation.NameInMap("Message")
private String message;
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("Success")
private Boolean success;
private GetSupplementDagrunInstanceResponseBody(Builder builder) {
this.code = builder.code;
this.httpStatusCode = builder.httpStatusCode;
this.instanceList = builder.instanceList;
this.message = builder.message;
this.requestId = builder.requestId;
this.success = builder.success;
}
public static Builder builder() {
return new Builder();
}
public static GetSupplementDagrunInstanceResponseBody create() {
return builder().build();
}
/**
* @return code
*/
public String getCode() {
return this.code;
}
/**
* @return httpStatusCode
*/
public Integer getHttpStatusCode() {
return this.httpStatusCode;
}
/**
* @return instanceList
*/
public java.util.List getInstanceList() {
return this.instanceList;
}
/**
* @return message
*/
public String getMessage() {
return this.message;
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return success
*/
public Boolean getSuccess() {
return this.success;
}
public static final class Builder {
private String code;
private Integer httpStatusCode;
private java.util.List instanceList;
private String message;
private String requestId;
private Boolean success;
/**
* Code.
*/
public Builder code(String code) {
this.code = code;
return this;
}
/**
* HttpStatusCode.
*/
public Builder httpStatusCode(Integer httpStatusCode) {
this.httpStatusCode = httpStatusCode;
return this;
}
/**
* InstanceList.
*/
public Builder instanceList(java.util.List instanceList) {
this.instanceList = instanceList;
return this;
}
/**
* Message.
*/
public Builder message(String message) {
this.message = message;
return this;
}
/**
* RequestId.
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Success.
*/
public Builder success(Boolean success) {
this.success = success;
return this;
}
public GetSupplementDagrunInstanceResponseBody build() {
return new GetSupplementDagrunInstanceResponseBody(this);
}
}
/**
*
* {@link GetSupplementDagrunInstanceResponseBody} extends {@link TeaModel}
*
* GetSupplementDagrunInstanceResponseBody
*/
public static class Creator extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Id")
private String id;
@com.aliyun.core.annotation.NameInMap("Name")
private String name;
private Creator(Builder builder) {
this.id = builder.id;
this.name = builder.name;
}
public static Builder builder() {
return new Builder();
}
public static Creator create() {
return builder().build();
}
/**
* @return id
*/
public String getId() {
return this.id;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
public static final class Builder {
private String id;
private String name;
/**
* Id.
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* Name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
public Creator build() {
return new Creator(this);
}
}
}
/**
*
* {@link GetSupplementDagrunInstanceResponseBody} extends {@link TeaModel}
*
* GetSupplementDagrunInstanceResponseBody
*/
public static class Modifier extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Id")
private String id;
@com.aliyun.core.annotation.NameInMap("Name")
private String name;
private Modifier(Builder builder) {
this.id = builder.id;
this.name = builder.name;
}
public static Builder builder() {
return new Builder();
}
public static Modifier create() {
return builder().build();
}
/**
* @return id
*/
public String getId() {
return this.id;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
public static final class Builder {
private String id;
private String name;
/**
* Id.
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* Name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
public Modifier build() {
return new Modifier(this);
}
}
}
/**
*
* {@link GetSupplementDagrunInstanceResponseBody} extends {@link TeaModel}
*
* GetSupplementDagrunInstanceResponseBody
*/
public static class OwnerList extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Id")
private String id;
@com.aliyun.core.annotation.NameInMap("Name")
private String name;
private OwnerList(Builder builder) {
this.id = builder.id;
this.name = builder.name;
}
public static Builder builder() {
return new Builder();
}
public static OwnerList create() {
return builder().build();
}
/**
* @return id
*/
public String getId() {
return this.id;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
public static final class Builder {
private String id;
private String name;
/**
* Id.
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* Name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
public OwnerList build() {
return new OwnerList(this);
}
}
}
/**
*
* {@link GetSupplementDagrunInstanceResponseBody} extends {@link TeaModel}
*
* GetSupplementDagrunInstanceResponseBody
*/
public static class NodeInfo extends TeaModel {
@com.aliyun.core.annotation.NameInMap("BizUnitName")
private String bizUnitName;
@com.aliyun.core.annotation.NameInMap("CreateTime")
private String createTime;
@com.aliyun.core.annotation.NameInMap("Creator")
private Creator creator;
@com.aliyun.core.annotation.NameInMap("Description")
private String description;
@com.aliyun.core.annotation.NameInMap("DryRun")
private Boolean dryRun;
@com.aliyun.core.annotation.NameInMap("From")
private String from;
@com.aliyun.core.annotation.NameInMap("HasDev")
private Boolean hasDev;
@com.aliyun.core.annotation.NameInMap("HasProd")
private Boolean hasProd;
@com.aliyun.core.annotation.NameInMap("Id")
private String id;
@com.aliyun.core.annotation.NameInMap("LastModifiedTime")
private String lastModifiedTime;
@com.aliyun.core.annotation.NameInMap("Modifier")
private Modifier modifier;
@com.aliyun.core.annotation.NameInMap("Name")
private String name;
@com.aliyun.core.annotation.NameInMap("OwnerList")
private java.util.List ownerList;
@com.aliyun.core.annotation.NameInMap("PriorityList")
private java.util.List priorityList;
@com.aliyun.core.annotation.NameInMap("ResourceGroupList")
private java.util.List resourceGroupList;
@com.aliyun.core.annotation.NameInMap("SchedulePaused")
private Boolean schedulePaused;
@com.aliyun.core.annotation.NameInMap("SchedulePeriodList")
private java.util.List schedulePeriodList;
@com.aliyun.core.annotation.NameInMap("SubDetailType")
private String subDetailType;
@com.aliyun.core.annotation.NameInMap("Type")
private String type;
private NodeInfo(Builder builder) {
this.bizUnitName = builder.bizUnitName;
this.createTime = builder.createTime;
this.creator = builder.creator;
this.description = builder.description;
this.dryRun = builder.dryRun;
this.from = builder.from;
this.hasDev = builder.hasDev;
this.hasProd = builder.hasProd;
this.id = builder.id;
this.lastModifiedTime = builder.lastModifiedTime;
this.modifier = builder.modifier;
this.name = builder.name;
this.ownerList = builder.ownerList;
this.priorityList = builder.priorityList;
this.resourceGroupList = builder.resourceGroupList;
this.schedulePaused = builder.schedulePaused;
this.schedulePeriodList = builder.schedulePeriodList;
this.subDetailType = builder.subDetailType;
this.type = builder.type;
}
public static Builder builder() {
return new Builder();
}
public static NodeInfo create() {
return builder().build();
}
/**
* @return bizUnitName
*/
public String getBizUnitName() {
return this.bizUnitName;
}
/**
* @return createTime
*/
public String getCreateTime() {
return this.createTime;
}
/**
* @return creator
*/
public Creator getCreator() {
return this.creator;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return dryRun
*/
public Boolean getDryRun() {
return this.dryRun;
}
/**
* @return from
*/
public String getFrom() {
return this.from;
}
/**
* @return hasDev
*/
public Boolean getHasDev() {
return this.hasDev;
}
/**
* @return hasProd
*/
public Boolean getHasProd() {
return this.hasProd;
}
/**
* @return id
*/
public String getId() {
return this.id;
}
/**
* @return lastModifiedTime
*/
public String getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
* @return modifier
*/
public Modifier getModifier() {
return this.modifier;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return ownerList
*/
public java.util.List getOwnerList() {
return this.ownerList;
}
/**
* @return priorityList
*/
public java.util.List getPriorityList() {
return this.priorityList;
}
/**
* @return resourceGroupList
*/
public java.util.List getResourceGroupList() {
return this.resourceGroupList;
}
/**
* @return schedulePaused
*/
public Boolean getSchedulePaused() {
return this.schedulePaused;
}
/**
* @return schedulePeriodList
*/
public java.util.List getSchedulePeriodList() {
return this.schedulePeriodList;
}
/**
* @return subDetailType
*/
public String getSubDetailType() {
return this.subDetailType;
}
/**
* @return type
*/
public String getType() {
return this.type;
}
public static final class Builder {
private String bizUnitName;
private String createTime;
private Creator creator;
private String description;
private Boolean dryRun;
private String from;
private Boolean hasDev;
private Boolean hasProd;
private String id;
private String lastModifiedTime;
private Modifier modifier;
private String name;
private java.util.List ownerList;
private java.util.List priorityList;
private java.util.List resourceGroupList;
private Boolean schedulePaused;
private java.util.List schedulePeriodList;
private String subDetailType;
private String type;
/**
* BizUnitName.
*/
public Builder bizUnitName(String bizUnitName) {
this.bizUnitName = bizUnitName;
return this;
}
/**
* CreateTime.
*/
public Builder createTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Creator.
*/
public Builder creator(Creator creator) {
this.creator = creator;
return this;
}
/**
* Description.
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* DryRun.
*/
public Builder dryRun(Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
/**
* From.
*/
public Builder from(String from) {
this.from = from;
return this;
}
/**
* HasDev.
*/
public Builder hasDev(Boolean hasDev) {
this.hasDev = hasDev;
return this;
}
/**
* HasProd.
*/
public Builder hasProd(Boolean hasProd) {
this.hasProd = hasProd;
return this;
}
/**
* Id.
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* LastModifiedTime.
*/
public Builder lastModifiedTime(String lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
return this;
}
/**
* Modifier.
*/
public Builder modifier(Modifier modifier) {
this.modifier = modifier;
return this;
}
/**
* Name.
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* OwnerList.
*/
public Builder ownerList(java.util.List ownerList) {
this.ownerList = ownerList;
return this;
}
/**
* PriorityList.
*/
public Builder priorityList(java.util.List priorityList) {
this.priorityList = priorityList;
return this;
}
/**
* ResourceGroupList.
*/
public Builder resourceGroupList(java.util.List resourceGroupList) {
this.resourceGroupList = resourceGroupList;
return this;
}
/**
* SchedulePaused.
*/
public Builder schedulePaused(Boolean schedulePaused) {
this.schedulePaused = schedulePaused;
return this;
}
/**
* SchedulePeriodList.
*/
public Builder schedulePeriodList(java.util.List schedulePeriodList) {
this.schedulePeriodList = schedulePeriodList;
return this;
}
/**
* SubDetailType.
*/
public Builder subDetailType(String subDetailType) {
this.subDetailType = subDetailType;
return this;
}
/**
* Type.
*/
public Builder type(String type) {
this.type = type;
return this;
}
public NodeInfo build() {
return new NodeInfo(this);
}
}
}
/**
*
* {@link GetSupplementDagrunInstanceResponseBody} extends {@link TeaModel}
*
* GetSupplementDagrunInstanceResponseBody
*/
public static class InstanceList extends TeaModel {
@com.aliyun.core.annotation.NameInMap("BizDate")
private Long bizDate;
@com.aliyun.core.annotation.NameInMap("DueTime")
private Long dueTime;
@com.aliyun.core.annotation.NameInMap("Duration")
private String duration;
@com.aliyun.core.annotation.NameInMap("EndExecuteTime")
private Long endExecuteTime;
@com.aliyun.core.annotation.NameInMap("ExtendInfo")
private String extendInfo;
@com.aliyun.core.annotation.NameInMap("Id")
private String id;
@com.aliyun.core.annotation.NameInMap("Index")
private Integer index;
@com.aliyun.core.annotation.NameInMap("NodeInfo")
private NodeInfo nodeInfo;
@com.aliyun.core.annotation.NameInMap("StartExecuteTime")
private Long startExecuteTime;
@com.aliyun.core.annotation.NameInMap("StatusList")
private java.util.List statusList;
@com.aliyun.core.annotation.NameInMap("Type")
private String type;
private InstanceList(Builder builder) {
this.bizDate = builder.bizDate;
this.dueTime = builder.dueTime;
this.duration = builder.duration;
this.endExecuteTime = builder.endExecuteTime;
this.extendInfo = builder.extendInfo;
this.id = builder.id;
this.index = builder.index;
this.nodeInfo = builder.nodeInfo;
this.startExecuteTime = builder.startExecuteTime;
this.statusList = builder.statusList;
this.type = builder.type;
}
public static Builder builder() {
return new Builder();
}
public static InstanceList create() {
return builder().build();
}
/**
* @return bizDate
*/
public Long getBizDate() {
return this.bizDate;
}
/**
* @return dueTime
*/
public Long getDueTime() {
return this.dueTime;
}
/**
* @return duration
*/
public String getDuration() {
return this.duration;
}
/**
* @return endExecuteTime
*/
public Long getEndExecuteTime() {
return this.endExecuteTime;
}
/**
* @return extendInfo
*/
public String getExtendInfo() {
return this.extendInfo;
}
/**
* @return id
*/
public String getId() {
return this.id;
}
/**
* @return index
*/
public Integer getIndex() {
return this.index;
}
/**
* @return nodeInfo
*/
public NodeInfo getNodeInfo() {
return this.nodeInfo;
}
/**
* @return startExecuteTime
*/
public Long getStartExecuteTime() {
return this.startExecuteTime;
}
/**
* @return statusList
*/
public java.util.List getStatusList() {
return this.statusList;
}
/**
* @return type
*/
public String getType() {
return this.type;
}
public static final class Builder {
private Long bizDate;
private Long dueTime;
private String duration;
private Long endExecuteTime;
private String extendInfo;
private String id;
private Integer index;
private NodeInfo nodeInfo;
private Long startExecuteTime;
private java.util.List statusList;
private String type;
/**
* BizDate.
*/
public Builder bizDate(Long bizDate) {
this.bizDate = bizDate;
return this;
}
/**
* DueTime.
*/
public Builder dueTime(Long dueTime) {
this.dueTime = dueTime;
return this;
}
/**
* Duration.
*/
public Builder duration(String duration) {
this.duration = duration;
return this;
}
/**
* EndExecuteTime.
*/
public Builder endExecuteTime(Long endExecuteTime) {
this.endExecuteTime = endExecuteTime;
return this;
}
/**
* ExtendInfo.
*/
public Builder extendInfo(String extendInfo) {
this.extendInfo = extendInfo;
return this;
}
/**
* Id.
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* Index.
*/
public Builder index(Integer index) {
this.index = index;
return this;
}
/**
* NodeInfo.
*/
public Builder nodeInfo(NodeInfo nodeInfo) {
this.nodeInfo = nodeInfo;
return this;
}
/**
* StartExecuteTime.
*/
public Builder startExecuteTime(Long startExecuteTime) {
this.startExecuteTime = startExecuteTime;
return this;
}
/**
* StatusList.
*/
public Builder statusList(java.util.List statusList) {
this.statusList = statusList;
return this;
}
/**
* Type.
*/
public Builder type(String type) {
this.type = type;
return this;
}
public InstanceList build() {
return new InstanceList(this);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy