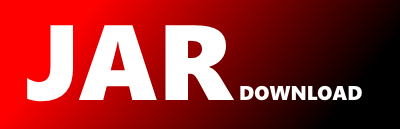
com.aliyun.sdk.service.dytnsapi20200217.DefaultAsyncClient Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.dytnsapi20200217;
import com.aliyun.core.http.*;
import com.aliyun.sdk.service.dytnsapi20200217.models.*;
import darabonba.core.utils.*;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import java.util.concurrent.CompletableFuture;
/**
* Main client.
*/
public final class DefaultAsyncClient implements AsyncClient {
protected final String product;
protected final String version;
protected final String endpointRule;
protected final java.util.Map endpointMap;
protected final TeaRequest REQUEST;
protected final TeaAsyncHandler handler;
protected DefaultAsyncClient(ClientConfiguration configuration) {
this.handler = new TeaAsyncHandler(configuration);
this.product = "Dytnsapi";
this.version = "2020-02-17";
this.endpointRule = "central";
this.endpointMap = new java.util.HashMap<>();
this.REQUEST = TeaRequest.create().setProduct(product).setEndpointRule(endpointRule).setEndpointMap(endpointMap).setVersion(version);
}
@Override
public void close() {
this.handler.close();
}
/**
* @param request the request parameters of CertNoThreeElementVerification CertNoThreeElementVerificationRequest
* @return CertNoThreeElementVerificationResponse
*/
@Override
public CompletableFuture certNoThreeElementVerification(CertNoThreeElementVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("CertNoThreeElementVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CertNoThreeElementVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of CertNoTwoElementVerification CertNoTwoElementVerificationRequest
* @return CertNoTwoElementVerificationResponse
*/
@Override
public CompletableFuture certNoTwoElementVerification(CertNoTwoElementVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("CertNoTwoElementVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CertNoTwoElementVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of services related to four-element verification for enterprises. For more information, see Billing.
*
* - You are charged only if the value of VerifyResult is true or false and the value of ReasonCode is 0, 1, or 2.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
*
* @param request the request parameters of CompanyFourElementsVerification CompanyFourElementsVerificationRequest
* @return CompanyFourElementsVerificationResponse
*/
@Override
public CompletableFuture companyFourElementsVerification(CompanyFourElementsVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("CompanyFourElementsVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CompanyFourElementsVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of services related to three-element verification for enterprises. For more information, see Billing.
*
* - You are charged only if the value of VerifyResult is true or false and the value of ReasonCode is 0, 1, or 2.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
*
* @param request the request parameters of CompanyThreeElementsVerification CompanyThreeElementsVerificationRequest
* @return CompanyThreeElementsVerificationResponse
*/
@Override
public CompletableFuture companyThreeElementsVerification(CompanyThreeElementsVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("CompanyThreeElementsVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CompanyThreeElementsVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of services related to two-element verification for enterprises. For more information, see Billing.
*
* - You are charged only if the value of VerifyResult is true or false and the value of ReasonCode is 0 or 1.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
*
* @param request the request parameters of CompanyTwoElementsVerification CompanyTwoElementsVerificationRequest
* @return CompanyTwoElementsVerificationResponse
*/
@Override
public CompletableFuture companyTwoElementsVerification(CompanyTwoElementsVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("CompanyTwoElementsVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(CompanyTwoElementsVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* You can call this operation to verify whether a phone number is a nonexistent number. When you call this operation to verify a number, the system charges you CNY 0.01 per verification based on the number of verifications. Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - You are charged only if the value of Code is OK and the value of Status is not UNKNOWN.
* - The prediction is not strictly accurate because Cell Phone Number Service predicts the nonexistent number probability by using AI algorithms. The accuracy rate of the prediction and the recall rate of empty numbers are about 95%. Pay attention to this point when you call this operation.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 100 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
* Authorization information
* By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
*
* @param request the request parameters of DescribeEmptyNumber DescribeEmptyNumberRequest
* @return DescribeEmptyNumberResponse
*/
@Override
public CompletableFuture describeEmptyNumber(DescribeEmptyNumberRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribeEmptyNumber").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribeEmptyNumberResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribeMobileOperatorAttribute DescribeMobileOperatorAttributeRequest
* @return DescribeMobileOperatorAttributeResponse
*/
@Override
public CompletableFuture describeMobileOperatorAttribute(DescribeMobileOperatorAttributeRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribeMobileOperatorAttribute").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribeMobileOperatorAttributeResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribePhoneNumberAnalysis DescribePhoneNumberAnalysisRequest
* @return DescribePhoneNumberAnalysisResponse
*/
@Override
public CompletableFuture describePhoneNumberAnalysis(DescribePhoneNumberAnalysisRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberAnalysis").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberAnalysisResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label. Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* @param request the request parameters of DescribePhoneNumberAnalysisAI DescribePhoneNumberAnalysisAIRequest
* @return DescribePhoneNumberAnalysisAIResponse
*/
@Override
public CompletableFuture describePhoneNumberAnalysisAI(DescribePhoneNumberAnalysisAIRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberAnalysisAI").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberAnalysisAIResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribePhoneNumberAnalysisPai DescribePhoneNumberAnalysisPaiRequest
* @return DescribePhoneNumberAnalysisPaiResponse
*/
@Override
public CompletableFuture describePhoneNumberAnalysisPai(DescribePhoneNumberAnalysisPaiRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberAnalysisPai").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberAnalysisPaiResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribePhoneNumberAnalysisTransparent DescribePhoneNumberAnalysisTransparentRequest
* @return DescribePhoneNumberAnalysisTransparentResponse
*/
@Override
public CompletableFuture describePhoneNumberAnalysisTransparent(DescribePhoneNumberAnalysisTransparentRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberAnalysisTransparent").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberAnalysisTransparentResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @deprecated OpenAPI DescribePhoneNumberAttribute is deprecated, please use Dytnsapi::2020-02-17::DescribePhoneNumberOperatorAttribute instead. * @description * Before you call this operation, make sure that you are familiar with the [billing](https://help.aliyun.com/document_detail/154751.html) of Cell Phone Number Service.
* * By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see [Grant permissions to RAM users](https://help.aliyun.com/document_detail/154006.html).
* ### [](#qps)QPS limits
* You can call this operation up to 2,000 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of DescribePhoneNumberAttribute DescribePhoneNumberAttributeRequest
* @return DescribePhoneNumberAttributeResponse
*/
@Deprecated
@Override
public CompletableFuture describePhoneNumberAttribute(DescribePhoneNumberAttributeRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberAttribute").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberAttributeResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* - Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* QPS limits
* You can call this operation up to 200 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of DescribePhoneNumberOnlineTime DescribePhoneNumberOnlineTimeRequest
* @return DescribePhoneNumberOnlineTimeResponse
*/
@Override
public CompletableFuture describePhoneNumberOnlineTime(DescribePhoneNumberOnlineTimeRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberOnlineTime").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberOnlineTimeResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - You can call this operation to obtain the carrier, registration location, and mobile number portability information about a phone number. You can query phone numbers in plaintext and phone numbers that are encrypted by using MD5 and SHA256.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
*
* @param request the request parameters of DescribePhoneNumberOperatorAttribute DescribePhoneNumberOperatorAttributeRequest
* @return DescribePhoneNumberOperatorAttributeResponse
*/
@Override
public CompletableFuture describePhoneNumberOperatorAttribute(DescribePhoneNumberOperatorAttributeRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberOperatorAttribute").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberOperatorAttributeResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribePhoneNumberOperatorAttributeAnnual DescribePhoneNumberOperatorAttributeAnnualRequest
* @return DescribePhoneNumberOperatorAttributeAnnualResponse
*/
@Override
public CompletableFuture describePhoneNumberOperatorAttributeAnnual(DescribePhoneNumberOperatorAttributeAnnualRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberOperatorAttributeAnnual").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberOperatorAttributeAnnualResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribePhoneNumberOperatorAttributeAnnualUse DescribePhoneNumberOperatorAttributeAnnualUseRequest
* @return DescribePhoneNumberOperatorAttributeAnnualUseResponse
*/
@Override
public CompletableFuture describePhoneNumberOperatorAttributeAnnualUse(DescribePhoneNumberOperatorAttributeAnnualUseRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberOperatorAttributeAnnualUse").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberOperatorAttributeAnnualUseResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of DescribePhoneNumberRisk DescribePhoneNumberRiskRequest
* @return DescribePhoneNumberRiskResponse
*/
@Override
public CompletableFuture describePhoneNumberRisk(DescribePhoneNumberRiskRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneNumberRisk").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneNumberRiskResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - You are charged for phone number verifications only if the value of Code is OK and the value of VerifyResult is not 0.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 100 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
* Authorization information
* By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
*
* @param request the request parameters of DescribePhoneTwiceTelVerify DescribePhoneTwiceTelVerifyRequest
* @return DescribePhoneTwiceTelVerifyResponse
*/
@Override
public CompletableFuture describePhoneTwiceTelVerify(DescribePhoneTwiceTelVerifyRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("DescribePhoneTwiceTelVerify").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(DescribePhoneTwiceTelVerifyResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetUAIDApplyTokenSign GetUAIDApplyTokenSignRequest
* @return GetUAIDApplyTokenSignResponse
*/
@Override
public CompletableFuture getUAIDApplyTokenSign(GetUAIDApplyTokenSignRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("GetUAIDApplyTokenSign").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetUAIDApplyTokenSignResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of GetUAIDConversionSign GetUAIDConversionSignRequest
* @return GetUAIDConversionSignResponse
*/
@Override
public CompletableFuture getUAIDConversionSign(GetUAIDConversionSignRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("GetUAIDConversionSign").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(GetUAIDConversionSignResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
* QPS limits
* You can call this operation up to 1,000 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of InvalidPhoneNumberFilter InvalidPhoneNumberFilterRequest
* @return InvalidPhoneNumberFilterResponse
*/
@Override
public CompletableFuture invalidPhoneNumberFilter(InvalidPhoneNumberFilterRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("InvalidPhoneNumberFilter").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(InvalidPhoneNumberFilterResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of PhoneNumberConvertService PhoneNumberConvertServiceRequest
* @return PhoneNumberConvertServiceResponse
*/
@Override
public CompletableFuture phoneNumberConvertService(PhoneNumberConvertServiceRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberConvertService").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberConvertServiceResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
* QPS limits
* You can call this operation up to 1,000 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberEncrypt PhoneNumberEncryptRequest
* @return PhoneNumberEncryptResponse
*/
@Override
public CompletableFuture phoneNumberEncrypt(PhoneNumberEncryptRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberEncrypt").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberEncryptResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 300 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberStatusForAccount PhoneNumberStatusForAccountRequest
* @return PhoneNumberStatusForAccountResponse
*/
@Override
public CompletableFuture phoneNumberStatusForAccount(PhoneNumberStatusForAccountRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberStatusForAccount").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberStatusForAccountResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 300 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberStatusForPublic PhoneNumberStatusForPublicRequest
* @return PhoneNumberStatusForPublicResponse
*/
@Override
public CompletableFuture phoneNumberStatusForPublic(PhoneNumberStatusForPublicRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberStatusForPublic").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberStatusForPublicResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 300 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberStatusForReal PhoneNumberStatusForRealRequest
* @return PhoneNumberStatusForRealResponse
*/
@Override
public CompletableFuture phoneNumberStatusForReal(PhoneNumberStatusForRealRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberStatusForReal").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberStatusForRealResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 300 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberStatusForSms PhoneNumberStatusForSmsRequest
* @return PhoneNumberStatusForSmsResponse
*/
@Override
public CompletableFuture phoneNumberStatusForSms(PhoneNumberStatusForSmsRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberStatusForSms").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberStatusForSmsResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - You are charged only if the value of Code is OK and the value of IsPrivacyNumber is true or false.
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 300 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberStatusForVirtual PhoneNumberStatusForVirtualRequest
* @return PhoneNumberStatusForVirtualResponse
*/
@Override
public CompletableFuture phoneNumberStatusForVirtual(PhoneNumberStatusForVirtualRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberStatusForVirtual").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberStatusForVirtualResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - By default, only Alibaba Cloud accounts can call this operation. RAM users can call this operation only after the RAM users are granted the related permissions. For more information, see Grant permissions to RAM users.
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
*
* QPS limits
* You can call this operation up to 300 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of PhoneNumberStatusForVoice PhoneNumberStatusForVoiceRequest
* @return PhoneNumberStatusForVoiceResponse
*/
@Override
public CompletableFuture phoneNumberStatusForVoice(PhoneNumberStatusForVoiceRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("PhoneNumberStatusForVoice").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(PhoneNumberStatusForVoiceResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryAvailableAuthCode QueryAvailableAuthCodeRequest
* @return QueryAvailableAuthCodeResponse
*/
@Override
public CompletableFuture queryAvailableAuthCode(QueryAvailableAuthCodeRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryAvailableAuthCode").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryAvailableAuthCodeResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryPhoneNumberOnlineTime QueryPhoneNumberOnlineTimeRequest
* @return QueryPhoneNumberOnlineTimeResponse
*/
@Override
public CompletableFuture queryPhoneNumberOnlineTime(QueryPhoneNumberOnlineTimeRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryPhoneNumberOnlineTime").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryPhoneNumberOnlineTimeResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryPhoneTwiceTelVerify QueryPhoneTwiceTelVerifyRequest
* @return QueryPhoneTwiceTelVerifyResponse
*/
@Override
public CompletableFuture queryPhoneTwiceTelVerify(QueryPhoneTwiceTelVerifyRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryPhoneTwiceTelVerify").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryPhoneTwiceTelVerifyResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryTagApplyRule QueryTagApplyRuleRequest
* @return QueryTagApplyRuleResponse
*/
@Override
public CompletableFuture queryTagApplyRule(QueryTagApplyRuleRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryTagApplyRule").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryTagApplyRuleResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryTagInfoBySelection QueryTagInfoBySelectionRequest
* @return QueryTagInfoBySelectionResponse
*/
@Override
public CompletableFuture queryTagInfoBySelection(QueryTagInfoBySelectionRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryTagInfoBySelection").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryTagInfoBySelectionResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryTagListPage QueryTagListPageRequest
* @return QueryTagListPageResponse
*/
@Override
public CompletableFuture queryTagListPage(QueryTagListPageRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryTagListPage").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryTagListPageResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of QueryUsageStatisticsByTagId QueryUsageStatisticsByTagIdRequest
* @return QueryUsageStatisticsByTagIdResponse
*/
@Override
public CompletableFuture queryUsageStatisticsByTagId(QueryUsageStatisticsByTagIdRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("QueryUsageStatisticsByTagId").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(QueryUsageStatisticsByTagIdResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
* - You are charged only if the value of Code is OK and the value of IsConsistent is not 2.
*
* QPS limits
* You can call this operation up to 200 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of ThreeElementsVerification ThreeElementsVerificationRequest
* @return ThreeElementsVerificationResponse
*/
@Override
public CompletableFuture threeElementsVerification(ThreeElementsVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("ThreeElementsVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(ThreeElementsVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* description :
* Before you call this operation, make sure that you are familiar with the billing of Cell Phone Number Service.
*
* - Before you call this operation, perform the following operations: Log on to the Cell Phone Number Service console. On the Labels page, find the label that you want to use, click Activate Now, enter the required information, and then submit your application. After your application is approved, you can use the label.
* - You are charged only if the value of Code is OK and the value of IsConsistent is not 2.
*
* QPS limits
* You can call this operation up to 200 times per second per account. If the number of calls per second exceeds the limit, throttling is triggered. As a result, your business may be affected. We recommend that you take note of the limit when you call this operation.
*
* @param request the request parameters of TwoElementsVerification TwoElementsVerificationRequest
* @return TwoElementsVerificationResponse
*/
@Override
public CompletableFuture twoElementsVerification(TwoElementsVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("TwoElementsVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(TwoElementsVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UAIDCollection UAIDCollectionRequest
* @return UAIDCollectionResponse
*/
@Override
public CompletableFuture uAIDCollection(UAIDCollectionRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("UAIDCollection").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UAIDCollectionResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UAIDConversion UAIDConversionRequest
* @return UAIDConversionResponse
*/
@Override
public CompletableFuture uAIDConversion(UAIDConversionRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("UAIDConversion").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UAIDConversionResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
/**
* @param request the request parameters of UAIDVerification UAIDVerificationRequest
* @return UAIDVerificationResponse
*/
@Override
public CompletableFuture uAIDVerification(UAIDVerificationRequest request) {
try {
this.handler.validateRequestModel(request);
TeaRequest teaRequest = REQUEST.copy().setStyle(RequestStyle.RPC).setAction("UAIDVerification").setMethod(HttpMethod.POST).setPathRegex("/").setBodyType(BodyType.JSON).setBodyIsForm(false).setReqBodyType(BodyType.JSON).formModel(request);
ClientExecutionParams params = new ClientExecutionParams().withInput(request).withRequest(teaRequest).withOutput(UAIDVerificationResponse.create());
return this.handler.execute(params);
} catch (Exception e) {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(e);
return future;
}
}
}