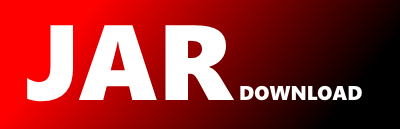
com.aliyun.sdk.service.ecs20140526.models.AllocateDedicatedHostsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-ecs20140526 Show documentation
Show all versions of alibabacloud-ecs20140526 Show documentation
Alibaba Cloud Ecs (20140526) Async SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import com.aliyun.core.annotation.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
* {@link AllocateDedicatedHostsRequest} extends {@link RequestModel}
*
* AllocateDedicatedHostsRequest
*/
public class AllocateDedicatedHostsRequest extends Request {
@Query
@NameInMap("NetworkAttributes")
private NetworkAttributes networkAttributes;
@Host
@NameInMap("SourceRegionId")
private String sourceRegionId;
@Query
@NameInMap("ActionOnMaintenance")
private String actionOnMaintenance;
@Query
@NameInMap("AutoPlacement")
private String autoPlacement;
@Query
@NameInMap("AutoReleaseTime")
private String autoReleaseTime;
@Query
@NameInMap("AutoRenew")
private Boolean autoRenew;
@Query
@NameInMap("AutoRenewPeriod")
private Integer autoRenewPeriod;
@Query
@NameInMap("ChargeType")
private String chargeType;
@Query
@NameInMap("ClientToken")
private String clientToken;
@Query
@NameInMap("CpuOverCommitRatio")
private Float cpuOverCommitRatio;
@Query
@NameInMap("DedicatedHostClusterId")
private String dedicatedHostClusterId;
@Query
@NameInMap("DedicatedHostName")
private String dedicatedHostName;
@Query
@NameInMap("DedicatedHostType")
@Validation(required = true)
private String dedicatedHostType;
@Query
@NameInMap("Description")
private String description;
@Query
@NameInMap("MinQuantity")
private Integer minQuantity;
@Query
@NameInMap("OwnerAccount")
private String ownerAccount;
@Query
@NameInMap("OwnerId")
private Long ownerId;
@Query
@NameInMap("Period")
private Integer period;
@Query
@NameInMap("PeriodUnit")
private String periodUnit;
@Query
@NameInMap("Quantity")
private Integer quantity;
@Query
@NameInMap("RegionId")
@Validation(required = true)
private String regionId;
@Query
@NameInMap("ResourceGroupId")
private String resourceGroupId;
@Query
@NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@Query
@NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@Query
@NameInMap("Tag")
private java.util.List < Tag> tag;
@Query
@NameInMap("ZoneId")
private String zoneId;
private AllocateDedicatedHostsRequest(Builder builder) {
super(builder);
this.networkAttributes = builder.networkAttributes;
this.sourceRegionId = builder.sourceRegionId;
this.actionOnMaintenance = builder.actionOnMaintenance;
this.autoPlacement = builder.autoPlacement;
this.autoReleaseTime = builder.autoReleaseTime;
this.autoRenew = builder.autoRenew;
this.autoRenewPeriod = builder.autoRenewPeriod;
this.chargeType = builder.chargeType;
this.clientToken = builder.clientToken;
this.cpuOverCommitRatio = builder.cpuOverCommitRatio;
this.dedicatedHostClusterId = builder.dedicatedHostClusterId;
this.dedicatedHostName = builder.dedicatedHostName;
this.dedicatedHostType = builder.dedicatedHostType;
this.description = builder.description;
this.minQuantity = builder.minQuantity;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.period = builder.period;
this.periodUnit = builder.periodUnit;
this.quantity = builder.quantity;
this.regionId = builder.regionId;
this.resourceGroupId = builder.resourceGroupId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.tag = builder.tag;
this.zoneId = builder.zoneId;
}
public static Builder builder() {
return new Builder();
}
public static AllocateDedicatedHostsRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return networkAttributes
*/
public NetworkAttributes getNetworkAttributes() {
return this.networkAttributes;
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return actionOnMaintenance
*/
public String getActionOnMaintenance() {
return this.actionOnMaintenance;
}
/**
* @return autoPlacement
*/
public String getAutoPlacement() {
return this.autoPlacement;
}
/**
* @return autoReleaseTime
*/
public String getAutoReleaseTime() {
return this.autoReleaseTime;
}
/**
* @return autoRenew
*/
public Boolean getAutoRenew() {
return this.autoRenew;
}
/**
* @return autoRenewPeriod
*/
public Integer getAutoRenewPeriod() {
return this.autoRenewPeriod;
}
/**
* @return chargeType
*/
public String getChargeType() {
return this.chargeType;
}
/**
* @return clientToken
*/
public String getClientToken() {
return this.clientToken;
}
/**
* @return cpuOverCommitRatio
*/
public Float getCpuOverCommitRatio() {
return this.cpuOverCommitRatio;
}
/**
* @return dedicatedHostClusterId
*/
public String getDedicatedHostClusterId() {
return this.dedicatedHostClusterId;
}
/**
* @return dedicatedHostName
*/
public String getDedicatedHostName() {
return this.dedicatedHostName;
}
/**
* @return dedicatedHostType
*/
public String getDedicatedHostType() {
return this.dedicatedHostType;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return minQuantity
*/
public Integer getMinQuantity() {
return this.minQuantity;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return period
*/
public Integer getPeriod() {
return this.period;
}
/**
* @return periodUnit
*/
public String getPeriodUnit() {
return this.periodUnit;
}
/**
* @return quantity
*/
public Integer getQuantity() {
return this.quantity;
}
/**
* @return regionId
*/
public String getRegionId() {
return this.regionId;
}
/**
* @return resourceGroupId
*/
public String getResourceGroupId() {
return this.resourceGroupId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return tag
*/
public java.util.List < Tag> getTag() {
return this.tag;
}
/**
* @return zoneId
*/
public String getZoneId() {
return this.zoneId;
}
public static final class Builder extends Request.Builder {
private NetworkAttributes networkAttributes;
private String sourceRegionId;
private String actionOnMaintenance;
private String autoPlacement;
private String autoReleaseTime;
private Boolean autoRenew;
private Integer autoRenewPeriod;
private String chargeType;
private String clientToken;
private Float cpuOverCommitRatio;
private String dedicatedHostClusterId;
private String dedicatedHostName;
private String dedicatedHostType;
private String description;
private Integer minQuantity;
private String ownerAccount;
private Long ownerId;
private Integer period;
private String periodUnit;
private Integer quantity;
private String regionId;
private String resourceGroupId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private java.util.List < Tag> tag;
private String zoneId;
private Builder() {
super();
}
private Builder(AllocateDedicatedHostsRequest request) {
super(request);
this.networkAttributes = request.networkAttributes;
this.sourceRegionId = request.sourceRegionId;
this.actionOnMaintenance = request.actionOnMaintenance;
this.autoPlacement = request.autoPlacement;
this.autoReleaseTime = request.autoReleaseTime;
this.autoRenew = request.autoRenew;
this.autoRenewPeriod = request.autoRenewPeriod;
this.chargeType = request.chargeType;
this.clientToken = request.clientToken;
this.cpuOverCommitRatio = request.cpuOverCommitRatio;
this.dedicatedHostClusterId = request.dedicatedHostClusterId;
this.dedicatedHostName = request.dedicatedHostName;
this.dedicatedHostType = request.dedicatedHostType;
this.description = request.description;
this.minQuantity = request.minQuantity;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.period = request.period;
this.periodUnit = request.periodUnit;
this.quantity = request.quantity;
this.regionId = request.regionId;
this.resourceGroupId = request.resourceGroupId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.tag = request.tag;
this.zoneId = request.zoneId;
}
/**
* NetworkAttributes.
*/
public Builder networkAttributes(NetworkAttributes networkAttributes) {
this.putQueryParameter("NetworkAttributes", networkAttributes);
this.networkAttributes = networkAttributes;
return this;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* ActionOnMaintenance.
*/
public Builder actionOnMaintenance(String actionOnMaintenance) {
this.putQueryParameter("ActionOnMaintenance", actionOnMaintenance);
this.actionOnMaintenance = actionOnMaintenance;
return this;
}
/**
* AutoPlacement.
*/
public Builder autoPlacement(String autoPlacement) {
this.putQueryParameter("AutoPlacement", autoPlacement);
this.autoPlacement = autoPlacement;
return this;
}
/**
* AutoReleaseTime.
*/
public Builder autoReleaseTime(String autoReleaseTime) {
this.putQueryParameter("AutoReleaseTime", autoReleaseTime);
this.autoReleaseTime = autoReleaseTime;
return this;
}
/**
* AutoRenew.
*/
public Builder autoRenew(Boolean autoRenew) {
this.putQueryParameter("AutoRenew", autoRenew);
this.autoRenew = autoRenew;
return this;
}
/**
* AutoRenewPeriod.
*/
public Builder autoRenewPeriod(Integer autoRenewPeriod) {
this.putQueryParameter("AutoRenewPeriod", autoRenewPeriod);
this.autoRenewPeriod = autoRenewPeriod;
return this;
}
/**
* ChargeType.
*/
public Builder chargeType(String chargeType) {
this.putQueryParameter("ChargeType", chargeType);
this.chargeType = chargeType;
return this;
}
/**
* ClientToken.
*/
public Builder clientToken(String clientToken) {
this.putQueryParameter("ClientToken", clientToken);
this.clientToken = clientToken;
return this;
}
/**
* CpuOverCommitRatio.
*/
public Builder cpuOverCommitRatio(Float cpuOverCommitRatio) {
this.putQueryParameter("CpuOverCommitRatio", cpuOverCommitRatio);
this.cpuOverCommitRatio = cpuOverCommitRatio;
return this;
}
/**
* DedicatedHostClusterId.
*/
public Builder dedicatedHostClusterId(String dedicatedHostClusterId) {
this.putQueryParameter("DedicatedHostClusterId", dedicatedHostClusterId);
this.dedicatedHostClusterId = dedicatedHostClusterId;
return this;
}
/**
* DedicatedHostName.
*/
public Builder dedicatedHostName(String dedicatedHostName) {
this.putQueryParameter("DedicatedHostName", dedicatedHostName);
this.dedicatedHostName = dedicatedHostName;
return this;
}
/**
* DedicatedHostType.
*/
public Builder dedicatedHostType(String dedicatedHostType) {
this.putQueryParameter("DedicatedHostType", dedicatedHostType);
this.dedicatedHostType = dedicatedHostType;
return this;
}
/**
* Description.
*/
public Builder description(String description) {
this.putQueryParameter("Description", description);
this.description = description;
return this;
}
/**
* MinQuantity.
*/
public Builder minQuantity(Integer minQuantity) {
this.putQueryParameter("MinQuantity", minQuantity);
this.minQuantity = minQuantity;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* Period.
*/
public Builder period(Integer period) {
this.putQueryParameter("Period", period);
this.period = period;
return this;
}
/**
* PeriodUnit.
*/
public Builder periodUnit(String periodUnit) {
this.putQueryParameter("PeriodUnit", periodUnit);
this.periodUnit = periodUnit;
return this;
}
/**
* Quantity.
*/
public Builder quantity(Integer quantity) {
this.putQueryParameter("Quantity", quantity);
this.quantity = quantity;
return this;
}
/**
* RegionId.
*/
public Builder regionId(String regionId) {
this.putQueryParameter("RegionId", regionId);
this.regionId = regionId;
return this;
}
/**
* ResourceGroupId.
*/
public Builder resourceGroupId(String resourceGroupId) {
this.putQueryParameter("ResourceGroupId", resourceGroupId);
this.resourceGroupId = resourceGroupId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* Tag.
*/
public Builder tag(java.util.List < Tag> tag) {
this.putQueryParameter("Tag", tag);
this.tag = tag;
return this;
}
/**
* ZoneId.
*/
public Builder zoneId(String zoneId) {
this.putQueryParameter("ZoneId", zoneId);
this.zoneId = zoneId;
return this;
}
@Override
public AllocateDedicatedHostsRequest build() {
return new AllocateDedicatedHostsRequest(this);
}
}
public static class NetworkAttributes extends TeaModel {
@NameInMap("SlbUdpTimeout")
private Integer slbUdpTimeout;
@NameInMap("UdpTimeout")
private Integer udpTimeout;
private NetworkAttributes(Builder builder) {
this.slbUdpTimeout = builder.slbUdpTimeout;
this.udpTimeout = builder.udpTimeout;
}
public static Builder builder() {
return new Builder();
}
public static NetworkAttributes create() {
return builder().build();
}
/**
* @return slbUdpTimeout
*/
public Integer getSlbUdpTimeout() {
return this.slbUdpTimeout;
}
/**
* @return udpTimeout
*/
public Integer getUdpTimeout() {
return this.udpTimeout;
}
public static final class Builder {
private Integer slbUdpTimeout;
private Integer udpTimeout;
/**
* SlbUdpTimeout.
*/
public Builder slbUdpTimeout(Integer slbUdpTimeout) {
this.slbUdpTimeout = slbUdpTimeout;
return this;
}
/**
* UdpTimeout.
*/
public Builder udpTimeout(Integer udpTimeout) {
this.udpTimeout = udpTimeout;
return this;
}
public NetworkAttributes build() {
return new NetworkAttributes(this);
}
}
}
public static class Tag extends TeaModel {
@NameInMap("Key")
private String key;
@NameInMap("Value")
private String value;
private Tag(Builder builder) {
this.key = builder.key;
this.value = builder.value;
}
public static Builder builder() {
return new Builder();
}
public static Tag create() {
return builder().build();
}
/**
* @return key
*/
public String getKey() {
return this.key;
}
/**
* @return value
*/
public String getValue() {
return this.value;
}
public static final class Builder {
private String key;
private String value;
/**
* Key.
*/
public Builder key(String key) {
this.key = key;
return this;
}
/**
* Value.
*/
public Builder value(String value) {
this.value = value;
return this;
}
public Tag build() {
return new Tag(this);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy