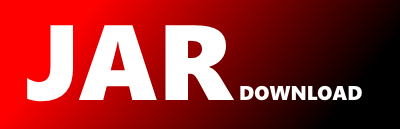
com.aliyun.sdk.service.ecs20140526.models.StopInstancesRequest Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import com.aliyun.core.annotation.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
* {@link StopInstancesRequest} extends {@link RequestModel}
*
* StopInstancesRequest
*/
public class StopInstancesRequest extends Request {
@Host
@NameInMap("SourceRegionId")
private String sourceRegionId;
@Query
@NameInMap("BatchOptimization")
private String batchOptimization;
@Query
@NameInMap("DryRun")
private Boolean dryRun;
@Query
@NameInMap("ForceStop")
private Boolean forceStop;
@Query
@NameInMap("InstanceId")
@Validation(required = true)
private java.util.List < String > instanceId;
@Query
@NameInMap("OwnerAccount")
private String ownerAccount;
@Query
@NameInMap("OwnerId")
private Long ownerId;
@Query
@NameInMap("RegionId")
@Validation(required = true)
private String regionId;
@Query
@NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@Query
@NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@Query
@NameInMap("StoppedMode")
private String stoppedMode;
private StopInstancesRequest(Builder builder) {
super(builder);
this.sourceRegionId = builder.sourceRegionId;
this.batchOptimization = builder.batchOptimization;
this.dryRun = builder.dryRun;
this.forceStop = builder.forceStop;
this.instanceId = builder.instanceId;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.regionId = builder.regionId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.stoppedMode = builder.stoppedMode;
}
public static Builder builder() {
return new Builder();
}
public static StopInstancesRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return batchOptimization
*/
public String getBatchOptimization() {
return this.batchOptimization;
}
/**
* @return dryRun
*/
public Boolean getDryRun() {
return this.dryRun;
}
/**
* @return forceStop
*/
public Boolean getForceStop() {
return this.forceStop;
}
/**
* @return instanceId
*/
public java.util.List < String > getInstanceId() {
return this.instanceId;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return regionId
*/
public String getRegionId() {
return this.regionId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return stoppedMode
*/
public String getStoppedMode() {
return this.stoppedMode;
}
public static final class Builder extends Request.Builder {
private String sourceRegionId;
private String batchOptimization;
private Boolean dryRun;
private Boolean forceStop;
private java.util.List < String > instanceId;
private String ownerAccount;
private Long ownerId;
private String regionId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private String stoppedMode;
private Builder() {
super();
}
private Builder(StopInstancesRequest request) {
super(request);
this.sourceRegionId = request.sourceRegionId;
this.batchOptimization = request.batchOptimization;
this.dryRun = request.dryRun;
this.forceStop = request.forceStop;
this.instanceId = request.instanceId;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.regionId = request.regionId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.stoppedMode = request.stoppedMode;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* The IDs of instances.
*/
public Builder batchOptimization(String batchOptimization) {
this.putQueryParameter("BatchOptimization", batchOptimization);
this.batchOptimization = batchOptimization;
return this;
}
/**
* The region ID of the instance. You can call the [DescribeRegions](~~25609~~) operation to query the most recent region list.
*/
public Builder dryRun(Boolean dryRun) {
this.putQueryParameter("DryRun", dryRun);
this.dryRun = dryRun;
return this;
}
/**
* The stop mode of the pay-as-you-go instance. Valid values:
*
*
* * StopCharging: economical mode. For information about the conditions on which `StopCharging` takes effect, see the "Conditions for enabling economical mode" section in [Economical mode](~~63353~~).
* * KeepCharging: standard mode. You continue to be charged for instances that are stopped in standard mode.
*
* Default value: If the conditions for enabling the economical mode are met and you have enabled this mode in the ECS console, the default value is [StopCharging](~~63353#default~~). For more information, see the "Enable economical mode" section in `Economical mode`. Otherwise, the default value is `KeepCharging`.
*/
public Builder forceStop(Boolean forceStop) {
this.putQueryParameter("ForceStop", forceStop);
this.forceStop = forceStop;
return this;
}
/**
* The ID of instance N. Valid values of N: 1 to 100.
*/
public Builder instanceId(java.util.List < String > instanceId) {
this.putQueryParameter("InstanceId", instanceId);
this.instanceId = instanceId;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* Specifies whether to forcibly stop the instance. Valid values:
*
*
* * true: forcibly stops the instance. This operation is equivalent to the power-off operation in common scenarios. Cache data that is not written to storage devices on the instance is lost.
* * false: normally stops the instance.
*
* Default value: false.
*/
public Builder regionId(String regionId) {
this.putQueryParameter("RegionId", regionId);
this.regionId = regionId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* Specifies the batch operation mode. Valid values:
*
*
* * AllTogether: In this mode, a success message is returned if all specified instances are stopped. If one or more of the specified instances fail the check when you set the DryRun parameter to false, none of the specified instances can be stopped and an error message is returned.
* * SuccessFirst: In this mode, each instance is separately stopped. The response contains the operation results for each instance.
*
* Default value: AllTogether.
*/
public Builder stoppedMode(String stoppedMode) {
this.putQueryParameter("StoppedMode", stoppedMode);
this.stoppedMode = stoppedMode;
return this;
}
@Override
public StopInstancesRequest build() {
return new StopInstancesRequest(this);
}
}
}