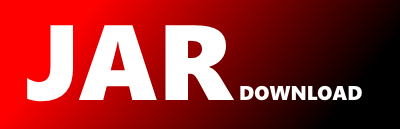
com.aliyun.sdk.service.ecs20140526.models.CreateActivationRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-ecs20140526 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
* {@link CreateActivationRequest} extends {@link RequestModel}
*
* CreateActivationRequest
*/
public class CreateActivationRequest extends Request {
@com.aliyun.core.annotation.Host
@com.aliyun.core.annotation.NameInMap("SourceRegionId")
private String sourceRegionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Description")
private String description;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InstanceCount")
private Integer instanceCount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InstanceName")
private String instanceName;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("IpAddressRange")
private String ipAddressRange;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("RegionId")
@com.aliyun.core.annotation.Validation(required = true)
private String regionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceGroupId")
private String resourceGroupId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Tag")
private java.util.List < Tag> tag;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("TimeToLiveInHours")
private Long timeToLiveInHours;
private CreateActivationRequest(Builder builder) {
super(builder);
this.sourceRegionId = builder.sourceRegionId;
this.description = builder.description;
this.instanceCount = builder.instanceCount;
this.instanceName = builder.instanceName;
this.ipAddressRange = builder.ipAddressRange;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.regionId = builder.regionId;
this.resourceGroupId = builder.resourceGroupId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.tag = builder.tag;
this.timeToLiveInHours = builder.timeToLiveInHours;
}
public static Builder builder() {
return new Builder();
}
public static CreateActivationRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return instanceCount
*/
public Integer getInstanceCount() {
return this.instanceCount;
}
/**
* @return instanceName
*/
public String getInstanceName() {
return this.instanceName;
}
/**
* @return ipAddressRange
*/
public String getIpAddressRange() {
return this.ipAddressRange;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return regionId
*/
public String getRegionId() {
return this.regionId;
}
/**
* @return resourceGroupId
*/
public String getResourceGroupId() {
return this.resourceGroupId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return tag
*/
public java.util.List < Tag> getTag() {
return this.tag;
}
/**
* @return timeToLiveInHours
*/
public Long getTimeToLiveInHours() {
return this.timeToLiveInHours;
}
public static final class Builder extends Request.Builder {
private String sourceRegionId;
private String description;
private Integer instanceCount;
private String instanceName;
private String ipAddressRange;
private String ownerAccount;
private Long ownerId;
private String regionId;
private String resourceGroupId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private java.util.List < Tag> tag;
private Long timeToLiveInHours;
private Builder() {
super();
}
private Builder(CreateActivationRequest request) {
super(request);
this.sourceRegionId = request.sourceRegionId;
this.description = request.description;
this.instanceCount = request.instanceCount;
this.instanceName = request.instanceName;
this.ipAddressRange = request.ipAddressRange;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.regionId = request.regionId;
this.resourceGroupId = request.resourceGroupId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.tag = request.tag;
this.timeToLiveInHours = request.timeToLiveInHours;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* The description of the activation code. The description must be 1 to 100 characters in length.
*/
public Builder description(String description) {
this.putQueryParameter("Description", description);
this.description = description;
return this;
}
/**
* The maximum number of times that you can use the activation code to register managed instances. Valid values: 1 to 1000.
*
*
* Default value: 10.
*/
public Builder instanceCount(Integer instanceCount) {
this.putQueryParameter("InstanceCount", instanceCount);
this.instanceCount = instanceCount;
return this;
}
/**
* The default instance name prefix. The prefix must be 2 to 50 characters in length and can contain letters, digits, periods (.), underscores (\_), hyphens (-), and colons (:). The prefix must start with a letter and cannot start with a digit, a special character, `http://`, or `https://`.
*
*
* If you use the activation code that is created by calling the CreateActivation operation to register managed instances, the instances are assigned sequential names that include the value of this parameter as a prefix. You can also specify a new instance name to replace the assigned sequential name when you register a managed instance.
*
* If you specify InstanceName when you register a managed instance, an instance name in the `-` format is generated. The number of digits in the \ value varies based on the number of digits in the `InstanceCount` value. Example: `001`. If you do not specify InstanceName, the hostname (Hostname) is used as the instance name.
*/
public Builder instanceName(String instanceName) {
this.putQueryParameter("InstanceName", instanceName);
this.instanceName = instanceName;
return this;
}
/**
* The IP addresses of hosts that can use the activation code. The value can be IPv4 addresses, IPv6 addresses, or CIDR blocks.
*/
public Builder ipAddressRange(String ipAddressRange) {
this.putQueryParameter("IpAddressRange", ipAddressRange);
this.ipAddressRange = ipAddressRange;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* The region ID. Supported regions: China (Qingdao), China (Beijing), China (Zhangjiakou), China (Hohhot), China (Ulanqab), China (Hangzhou), China (Shanghai), China (Shenzhen), China (Heyuan), China (Guangzhou), China (Chengdu), China (Hong Kong), Singapore, Japan (Tokyo), US (Silicon Valley), and US (Virginia). You can call the [DescribeRegions](~~25609~~) operation to query the most recent region list.
*/
public Builder regionId(String regionId) {
this.putQueryParameter("RegionId", regionId);
this.regionId = regionId;
return this;
}
/**
* The ID of the resource group to which to assign the activation code.
*/
public Builder resourceGroupId(String resourceGroupId) {
this.putQueryParameter("ResourceGroupId", resourceGroupId);
this.resourceGroupId = resourceGroupId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* The tags to add to the activation code.
*/
public Builder tag(java.util.List < Tag> tag) {
this.putQueryParameter("Tag", tag);
this.tag = tag;
return this;
}
/**
* The validity period of the activation code. After the validity period ends, you can no longer use the activation code to register managed instances. Unit: hours. Valid values: 1 to 876576. 876576 hours is equal to 100 years.
*
*
* Default value: 4.
*/
public Builder timeToLiveInHours(Long timeToLiveInHours) {
this.putQueryParameter("TimeToLiveInHours", timeToLiveInHours);
this.timeToLiveInHours = timeToLiveInHours;
return this;
}
@Override
public CreateActivationRequest build() {
return new CreateActivationRequest(this);
}
}
public static class Tag extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Key")
private String key;
@com.aliyun.core.annotation.NameInMap("Value")
private String value;
private Tag(Builder builder) {
this.key = builder.key;
this.value = builder.value;
}
public static Builder builder() {
return new Builder();
}
public static Tag create() {
return builder().build();
}
/**
* @return key
*/
public String getKey() {
return this.key;
}
/**
* @return value
*/
public String getValue() {
return this.value;
}
public static final class Builder {
private String key;
private String value;
/**
* The key of tag N to add to the activation code. Valid values of N: 1 to 20. The tag key cannot be an empty string.
*
*
* If a single tag is specified to query resources, up to 1,000 resources that have this tag added can be displayed in the response. If multiple tags are specified to query resources, up to 1,000 resources that have all these tags added can be displayed in the response. To query more than 1,000 resources that have specified tags, call [ListTagResources](~~110425~~).
*
* The tag key can be up to 64 characters in length and cannot start with `acs:` or `aliyun`. It cannot contain `http://` or `https://`.
*/
public Builder key(String key) {
this.key = key;
return this;
}
/**
* The value of tag N to add to the activation code. Valid values of N: 1 to 20. The tag value can be an empty string.
*
*
* It can be up to 128 characters in length and cannot contain `http://` or `https://`.
*/
public Builder value(String value) {
this.value = value;
return this;
}
public Tag build() {
return new Tag(this);
}
}
}
}