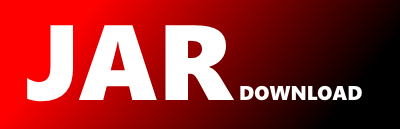
com.aliyun.sdk.service.ecs20140526.models.ModifySecurityGroupEgressRuleRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-ecs20140526 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
* {@link ModifySecurityGroupEgressRuleRequest} extends {@link RequestModel}
*
* ModifySecurityGroupEgressRuleRequest
*/
public class ModifySecurityGroupEgressRuleRequest extends Request {
@com.aliyun.core.annotation.Host
@com.aliyun.core.annotation.NameInMap("SourceRegionId")
private String sourceRegionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ClientToken")
private String clientToken;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Description")
private String description;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DestCidrIp")
private String destCidrIp;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DestGroupId")
private String destGroupId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DestGroupOwnerAccount")
private String destGroupOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DestGroupOwnerId")
private Long destGroupOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DestPrefixListId")
private String destPrefixListId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("IpProtocol")
private String ipProtocol;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Ipv6DestCidrIp")
private String ipv6DestCidrIp;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Ipv6SourceCidrIp")
private String ipv6SourceCidrIp;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("NicType")
private String nicType;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Policy")
private String policy;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("PortRange")
private String portRange;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Priority")
private String priority;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("RegionId")
@com.aliyun.core.annotation.Validation(required = true)
private String regionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("SecurityGroupId")
@com.aliyun.core.annotation.Validation(required = true)
private String securityGroupId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("SecurityGroupRuleId")
private String securityGroupRuleId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("SourceCidrIp")
private String sourceCidrIp;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("SourcePortRange")
private String sourcePortRange;
private ModifySecurityGroupEgressRuleRequest(Builder builder) {
super(builder);
this.sourceRegionId = builder.sourceRegionId;
this.clientToken = builder.clientToken;
this.description = builder.description;
this.destCidrIp = builder.destCidrIp;
this.destGroupId = builder.destGroupId;
this.destGroupOwnerAccount = builder.destGroupOwnerAccount;
this.destGroupOwnerId = builder.destGroupOwnerId;
this.destPrefixListId = builder.destPrefixListId;
this.ipProtocol = builder.ipProtocol;
this.ipv6DestCidrIp = builder.ipv6DestCidrIp;
this.ipv6SourceCidrIp = builder.ipv6SourceCidrIp;
this.nicType = builder.nicType;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.policy = builder.policy;
this.portRange = builder.portRange;
this.priority = builder.priority;
this.regionId = builder.regionId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.securityGroupId = builder.securityGroupId;
this.securityGroupRuleId = builder.securityGroupRuleId;
this.sourceCidrIp = builder.sourceCidrIp;
this.sourcePortRange = builder.sourcePortRange;
}
public static Builder builder() {
return new Builder();
}
public static ModifySecurityGroupEgressRuleRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return clientToken
*/
public String getClientToken() {
return this.clientToken;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return destCidrIp
*/
public String getDestCidrIp() {
return this.destCidrIp;
}
/**
* @return destGroupId
*/
public String getDestGroupId() {
return this.destGroupId;
}
/**
* @return destGroupOwnerAccount
*/
public String getDestGroupOwnerAccount() {
return this.destGroupOwnerAccount;
}
/**
* @return destGroupOwnerId
*/
public Long getDestGroupOwnerId() {
return this.destGroupOwnerId;
}
/**
* @return destPrefixListId
*/
public String getDestPrefixListId() {
return this.destPrefixListId;
}
/**
* @return ipProtocol
*/
public String getIpProtocol() {
return this.ipProtocol;
}
/**
* @return ipv6DestCidrIp
*/
public String getIpv6DestCidrIp() {
return this.ipv6DestCidrIp;
}
/**
* @return ipv6SourceCidrIp
*/
public String getIpv6SourceCidrIp() {
return this.ipv6SourceCidrIp;
}
/**
* @return nicType
*/
public String getNicType() {
return this.nicType;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return policy
*/
public String getPolicy() {
return this.policy;
}
/**
* @return portRange
*/
public String getPortRange() {
return this.portRange;
}
/**
* @return priority
*/
public String getPriority() {
return this.priority;
}
/**
* @return regionId
*/
public String getRegionId() {
return this.regionId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return securityGroupId
*/
public String getSecurityGroupId() {
return this.securityGroupId;
}
/**
* @return securityGroupRuleId
*/
public String getSecurityGroupRuleId() {
return this.securityGroupRuleId;
}
/**
* @return sourceCidrIp
*/
public String getSourceCidrIp() {
return this.sourceCidrIp;
}
/**
* @return sourcePortRange
*/
public String getSourcePortRange() {
return this.sourcePortRange;
}
public static final class Builder extends Request.Builder {
private String sourceRegionId;
private String clientToken;
private String description;
private String destCidrIp;
private String destGroupId;
private String destGroupOwnerAccount;
private Long destGroupOwnerId;
private String destPrefixListId;
private String ipProtocol;
private String ipv6DestCidrIp;
private String ipv6SourceCidrIp;
private String nicType;
private String ownerAccount;
private Long ownerId;
private String policy;
private String portRange;
private String priority;
private String regionId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private String securityGroupId;
private String securityGroupRuleId;
private String sourceCidrIp;
private String sourcePortRange;
private Builder() {
super();
}
private Builder(ModifySecurityGroupEgressRuleRequest request) {
super(request);
this.sourceRegionId = request.sourceRegionId;
this.clientToken = request.clientToken;
this.description = request.description;
this.destCidrIp = request.destCidrIp;
this.destGroupId = request.destGroupId;
this.destGroupOwnerAccount = request.destGroupOwnerAccount;
this.destGroupOwnerId = request.destGroupOwnerId;
this.destPrefixListId = request.destPrefixListId;
this.ipProtocol = request.ipProtocol;
this.ipv6DestCidrIp = request.ipv6DestCidrIp;
this.ipv6SourceCidrIp = request.ipv6SourceCidrIp;
this.nicType = request.nicType;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.policy = request.policy;
this.portRange = request.portRange;
this.priority = request.priority;
this.regionId = request.regionId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.securityGroupId = request.securityGroupId;
this.securityGroupRuleId = request.securityGroupRuleId;
this.sourceCidrIp = request.sourceCidrIp;
this.sourcePortRange = request.sourcePortRange;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* The client token that is used to ensure the idempotence of the request. You can use the client to generate the token, but you must make sure that the token is unique among different requests. The token can contain only ASCII characters and cannot exceed 64 characters in length.**** For more information, see [How to ensure idempotence](~~25693~~).
*/
public Builder clientToken(String clientToken) {
this.putQueryParameter("ClientToken", clientToken);
this.clientToken = clientToken;
return this;
}
/**
* The description of the security group rule. The description must be 1 to 512 characters in length.
*/
public Builder description(String description) {
this.putQueryParameter("Description", description);
this.description = description;
return this;
}
/**
* The destination IPv4 CIDR block. IPv4 CIDR blocks and IPv4 addresses are supported.
*
*
* By default, this parameter is left empty.
*/
public Builder destCidrIp(String destCidrIp) {
this.putQueryParameter("DestCidrIp", destCidrIp);
this.destCidrIp = destCidrIp;
return this;
}
/**
* The ID of the destination security group. You must specify at least one of `DestGroupId` and `DestCidrIp`.
*
*
* * At least one of DestGroupId, DestCidrIp, Ipv6DestCidrIp, and DestPrefixListId must be specified.
* * If DestGroupId is specified but DestCidrIp is not specified, the NicType parameter can be set only to intranet.
* * If both DestGroupId and DestCidrIp are specified, DestCidrIp takes precedence.
*/
public Builder destGroupId(String destGroupId) {
this.putQueryParameter("DestGroupId", destGroupId);
this.destGroupId = destGroupId;
return this;
}
/**
* The Alibaba Cloud account that manages the destination security group when you set security group rule N across accounts.
*/
public Builder destGroupOwnerAccount(String destGroupOwnerAccount) {
this.putQueryParameter("DestGroupOwnerAccount", destGroupOwnerAccount);
this.destGroupOwnerAccount = destGroupOwnerAccount;
return this;
}
/**
* The ID of the Alibaba Cloud account that manages the destination security group when you set security group rule N across accounts.
*/
public Builder destGroupOwnerId(Long destGroupOwnerId) {
this.putQueryParameter("DestGroupOwnerId", destGroupOwnerId);
this.destGroupOwnerId = destGroupOwnerId;
return this;
}
/**
* The ID of the destination prefix list. You can call the [DescribePrefixLists](~~205046~~) operation to query the IDs of available prefix lists.
*
*
* If you specify `DestCidrIp`, `Ipv6DestCidrIp`, or `DestGroupId`, this parameter is ignored.
*/
public Builder destPrefixListId(String destPrefixListId) {
this.putQueryParameter("DestPrefixListId", destPrefixListId);
this.destPrefixListId = destPrefixListId;
return this;
}
/**
* The transport layer protocol of the security group rule. The value of this parameter is case-insensitive. Valid values:
*
*
* * ICMP
* * GRE
* * TCP
* * UDP
* * ALL: All protocols are supported.
*/
public Builder ipProtocol(String ipProtocol) {
this.putQueryParameter("IpProtocol", ipProtocol);
this.ipProtocol = ipProtocol;
return this;
}
/**
* The destination IPv6 CIDR block. IPv6 CIDR blocks and IPv6 addresses are supported.
*
*
* > Only the IP addresses of instances in virtual private clouds (VPCs) are supported. You cannot specify both Ipv6DestCidrIp and `DestCidrIp`.
*
* By default, this parameter is left empty.
*/
public Builder ipv6DestCidrIp(String ipv6DestCidrIp) {
this.putQueryParameter("Ipv6DestCidrIp", ipv6DestCidrIp);
this.ipv6DestCidrIp = ipv6DestCidrIp;
return this;
}
/**
* The source IPv6 CIDR block. IPv6 CIDR blocks and IPv6 addresses are supported.
*
*
* > Only the IP addresses of instances in VPCs are supported. You cannot specify both Ipv6SourceCidrIp and `SourceCidrIp`.
*
* By default, this parameter is left empty.
*/
public Builder ipv6SourceCidrIp(String ipv6SourceCidrIp) {
this.putQueryParameter("Ipv6SourceCidrIp", ipv6SourceCidrIp);
this.ipv6SourceCidrIp = ipv6SourceCidrIp;
return this;
}
/**
* You cannot modify this parameter when you modify a security group rule by specifying its ID.\
*
* You can add a new rule that meets your business requirements and delete the original rule.
*/
public Builder nicType(String nicType) {
this.putQueryParameter("NicType", nicType);
this.nicType = nicType;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* The action of the security group rule. Valid values:
*
*
* * accept: allows access.
* * drop: denies access and returns no responses.
*
* Default value: accept.
*/
public Builder policy(String policy) {
this.putQueryParameter("Policy", policy);
this.policy = policy;
return this;
}
/**
* The range of destination ports that correspond to the transport layer protocol. Valid values:
*
*
* * If you set IpProtocol to TCP or UDP, the port number range is 1 to 65535. Separate the start port number and the end port number with a forward slash (/). Example: 1/200.
* * If you set IpProtocol to ICMP, the port number range is -1/-1.
* * If you set IpProtocol to GRE, the port number range is -1/-1.
* * If you set IpProtocol to ALL, the port number range is -1/-1.
*/
public Builder portRange(String portRange) {
this.putQueryParameter("PortRange", portRange);
this.portRange = portRange;
return this;
}
/**
* The priority of the security group rule. Valid values: 1 to 100.
*
*
* Default value: 1.
*/
public Builder priority(String priority) {
this.putQueryParameter("Priority", priority);
this.priority = priority;
return this;
}
/**
* The region ID of the security group. You can call the [DescribeRegions](~~25609~~) operation to query the most recent region list.
*/
public Builder regionId(String regionId) {
this.putQueryParameter("RegionId", regionId);
this.regionId = regionId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* The ID of the security group.
*/
public Builder securityGroupId(String securityGroupId) {
this.putQueryParameter("SecurityGroupId", securityGroupId);
this.securityGroupId = securityGroupId;
return this;
}
/**
* The ID of the security group rule.\
*
* This parameter is required when you modify a security group rule based on the security group rule ID.
*/
public Builder securityGroupRuleId(String securityGroupRuleId) {
this.putQueryParameter("SecurityGroupRuleId", securityGroupRuleId);
this.securityGroupRuleId = securityGroupRuleId;
return this;
}
/**
* The source IPv4 CIDR block. IPv4 CIDR blocks and IPv4 addresses are supported.
*
*
* By default, this parameter is left empty.
*/
public Builder sourceCidrIp(String sourceCidrIp) {
this.putQueryParameter("SourceCidrIp", sourceCidrIp);
this.sourceCidrIp = sourceCidrIp;
return this;
}
/**
* The range of source ports that correspond to the transport layer protocol. Valid values:
*
*
* * If you set IpProtocol to TCP or UDP, the port number range is 1 to 65535. Separate the start port number and the end port number with a forward slash (/). Example: 1/200.
* * If you set IpProtocol to ICMP, the port number range is -1/-1.
* * If you set IpProtocol to GRE, the port number range is -1/-1.
* * If you set IpProtocol to ALL, the port number range is -1/-1.
*/
public Builder sourcePortRange(String sourcePortRange) {
this.putQueryParameter("SourcePortRange", sourcePortRange);
this.sourcePortRange = sourcePortRange;
return this;
}
@Override
public ModifySecurityGroupEgressRuleRequest build() {
return new ModifySecurityGroupEgressRuleRequest(this);
}
}
}